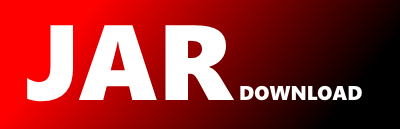
hu.bme.mit.theta.xcfa.cli.utils.GraphmlWitnessWriter.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of theta-xcfa-cli Show documentation
Show all versions of theta-xcfa-cli Show documentation
Xcfa Cli subproject in the Theta model checking framework
The newest version!
/*
* Copyright 2024 Budapest University of Technology and Economics
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package hu.bme.mit.theta.xcfa.cli.utils
import hu.bme.mit.theta.analysis.Trace
import hu.bme.mit.theta.analysis.algorithm.SafetyResult
import hu.bme.mit.theta.analysis.expl.ExplState
import hu.bme.mit.theta.analysis.ptr.PtrState
import hu.bme.mit.theta.frontend.ParseContext
import hu.bme.mit.theta.solver.SolverFactory
import hu.bme.mit.theta.xcfa.analysis.XcfaAction
import hu.bme.mit.theta.xcfa.analysis.XcfaState
import hu.bme.mit.theta.xcfa.cli.witnesses.GraphmlWitness
import hu.bme.mit.theta.xcfa.cli.witnesses.XcfaTraceConcretizer
import hu.bme.mit.theta.xcfa.cli.witnesses.traceToWitness
import java.io.BufferedWriter
import java.io.File
import java.io.FileWriter
import java.io.IOException
import java.text.DateFormat
import java.text.SimpleDateFormat
import java.util.*
class GraphmlWitnessWriter {
fun writeWitness(
safetyResult: SafetyResult<*, *>,
inputFile: File,
cexSolverFactory: SolverFactory,
parseContext: ParseContext,
witnessfile: File,
) {
// TODO eliminate the need for the instanceof check
if (safetyResult.isUnsafe && safetyResult.asUnsafe().cex is Trace<*, *>) {
val concrTrace: Trace, XcfaAction> =
XcfaTraceConcretizer.concretize(
safetyResult.asUnsafe().cex as Trace>, XcfaAction>?,
cexSolverFactory,
parseContext,
)
val witnessTrace = traceToWitness(trace = concrTrace, parseContext = parseContext)
val graphmlWitness = GraphmlWitness(witnessTrace, inputFile)
val xml = graphmlWitness.toPrettyXml()
witnessfile.writeText(xml)
} else if (safetyResult.isSafe) {
val taskHash = WitnessWriter.createTaskHash(inputFile.absolutePath)
val dummyWitness = StringBuilder()
dummyWitness
.append(
""
)
.append(System.lineSeparator())
.append("")
.append(System.lineSeparator())
.append("")
.append(System.lineSeparator())
.append("")
.append(System.lineSeparator())
.append("false ")
.append(System.lineSeparator())
.append(" ")
.append(System.lineSeparator())
.append("")
.append(System.lineSeparator())
.append("false ")
.append(System.lineSeparator())
.append(" ")
.append(System.lineSeparator())
.append(
""
)
.append(System.lineSeparator())
.append("")
.append(System.lineSeparator())
.append(
""
)
.append(System.lineSeparator())
.append(
""
)
.append(System.lineSeparator())
.append(
""
)
.append(System.lineSeparator())
.append(
""
)
.append(System.lineSeparator())
.append("")
.append(System.lineSeparator())
.append("correctness_witness")
.append(System.lineSeparator())
.append("theta")
.append(System.lineSeparator())
.append(
"CHECK( init(main()), LTL(G ! call(reach_error())) )"
)
.append(System.lineSeparator())
.append("C")
.append(System.lineSeparator())
.append("32bit")
.append(System.lineSeparator())
.append("")
dummyWitness.append(taskHash)
dummyWitness
.append("")
.append(System.lineSeparator())
.append("")
val tz: TimeZone = TimeZone.getTimeZone("UTC")
val df: DateFormat =
SimpleDateFormat(
"yyyy-MM-dd'T'HH:mm:ss'Z'"
) // Quoted "Z" to indicate UTC, no timezone offset
df.timeZone = tz
val isoDate: String = df.format(Date())
dummyWitness.append(isoDate)
dummyWitness
.append("")
.append(System.lineSeparator())
.append("")
dummyWitness.append(inputFile.name)
dummyWitness
.append("")
.append(System.lineSeparator())
.append("")
.append(System.lineSeparator())
.append("true")
.append(System.lineSeparator())
.append(" ")
.append(System.lineSeparator())
.append(" ")
.append(System.lineSeparator())
.append(" ")
try {
BufferedWriter(FileWriter(witnessfile)).use { bw -> bw.write(dummyWitness.toString()) }
} catch (ioe: IOException) {
ioe.printStackTrace()
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy