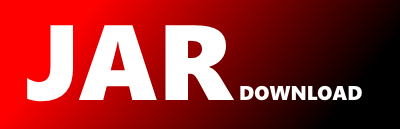
hu.bme.mit.theta.xta.XtaProcess Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of theta-xta Show documentation
Show all versions of theta-xta Show documentation
Xta subproject in the Theta model checking framework
The newest version!
/*
* Copyright 2024 Budapest University of Technology and Economics
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package hu.bme.mit.theta.xta;
import com.google.common.collect.ImmutableList;
import hu.bme.mit.theta.common.Utils;
import hu.bme.mit.theta.common.container.Containers;
import hu.bme.mit.theta.core.decl.VarDecl;
import hu.bme.mit.theta.core.stmt.Stmt;
import hu.bme.mit.theta.core.type.Expr;
import hu.bme.mit.theta.core.type.booltype.BoolType;
import hu.bme.mit.theta.core.utils.ExprUtils;
import hu.bme.mit.theta.core.utils.StmtUtils;
import java.util.*;
import static com.google.common.base.Preconditions.checkArgument;
import static com.google.common.base.Preconditions.checkNotNull;
public final class XtaProcess {
private final String name;
private final XtaSystem system;
private final Collection locs;
private final Collection edges;
private Loc initLoc;
private final Collection unmodLocs;
private final Collection unmodEdges;
////
private XtaProcess(final XtaSystem system, final String name) {
this.system = checkNotNull(system);
this.name = checkNotNull(name);
locs = Containers.createSet();
edges = new ArrayList<>();
unmodLocs = Collections.unmodifiableCollection(locs);
unmodEdges = Collections.unmodifiableCollection(edges);
}
static XtaProcess create(final XtaSystem system, final String name) {
return new XtaProcess(system, name);
}
////
public String getName() {
return name;
}
public XtaSystem getSystem() {
return system;
}
public Collection getLocs() {
return unmodLocs;
}
public Collection getEdges() {
return unmodEdges;
}
public Loc getInitLoc() {
checkNotNull(initLoc);
return initLoc;
}
////
public void setInitLoc(final Loc loc) {
checkNotNull(loc);
checkArgument(locs.contains(loc));
this.initLoc = loc;
}
public Loc createLoc(final String name, final LocKind kind,
final Collection> invars) {
final Loc loc = new Loc(name, kind, invars);
locs.add(loc);
return loc;
}
public Edge createEdge(final Loc source, final Loc target,
final Collection> guards,
final Optional sync, final List updates) {
checkArgument(locs.contains(source));
checkArgument(locs.contains(target));
final Edge edge = new Edge(source, target, guards, sync, updates);
source.outEdges.add(edge);
target.inEdges.add(edge);
return edge;
}
////
private Collection createGuards(final Collection> exprs) {
checkNotNull(exprs);
final ImmutableList.Builder builder = ImmutableList.builder();
for (final Expr expr : exprs) {
final Collection> vars = ExprUtils.getVars(expr);
boolean dataExpr = false;
boolean clockExpr = false;
for (final VarDecl> varDecl : vars) {
if (system.getDataVars().contains(varDecl)) {
dataExpr = true;
} else if (system.getClockVars().contains(varDecl)) {
clockExpr = true;
} else {
throw new IllegalArgumentException("Undeclared variable: " + varDecl.getName());
}
}
final Guard guard;
if (dataExpr && !clockExpr) {
guard = Guard.dataGuard(expr);
} else if (!dataExpr && clockExpr) {
guard = Guard.clockGuard(expr);
} else {
throw new UnsupportedOperationException();
}
builder.add(guard);
}
return builder.build();
}
private List createUpdates(final List stmts) {
checkNotNull(stmts);
final ImmutableList.Builder builder = ImmutableList.builder();
for (final Stmt stmt : stmts) {
final Collection> varsDecls = StmtUtils.getVars(stmt);
boolean dataStmt = false;
boolean clockStmt = false;
for (final VarDecl> varDecl : varsDecls) {
if (system.getDataVars().contains(varDecl)) {
dataStmt = true;
} else if (system.getClockVars().contains(varDecl)) {
clockStmt = true;
} else {
throw new IllegalArgumentException("Undeclared variable: " + varDecl.getName());
}
}
final Update update;
if (dataStmt && !clockStmt) {
update = Update.dataUpdate(stmt);
} else if (!dataStmt && clockStmt) {
update = Update.clockUpdate(stmt);
} else {
throw new UnsupportedOperationException();
}
builder.add(update);
}
return builder.build();
}
////
public enum LocKind {
NORMAL, URGENT, COMMITTED;
}
public final class Loc {
private final Collection inEdges;
private final Collection outEdges;
private final String name;
private final LocKind kind;
private final Collection invars;
private final Collection unmodInEdges;
private final Collection unmodOutEdges;
private Loc(final String name, final LocKind kind,
final Collection> invars) {
inEdges = new ArrayList<>();
outEdges = new ArrayList<>();
this.name = checkNotNull(name);
this.kind = checkNotNull(kind);
this.invars = createGuards(invars);
unmodInEdges = Collections.unmodifiableCollection(inEdges);
unmodOutEdges = Collections.unmodifiableCollection(outEdges);
}
public Collection getInEdges() {
return unmodInEdges;
}
public Collection getOutEdges() {
return unmodOutEdges;
}
public String getName() {
return name;
}
public LocKind getKind() {
return kind;
}
public Collection getInvars() {
return invars;
}
@Override
public String toString() {
return Utils.lispStringBuilder("loc").add(name).toString();
}
}
////
public final class Edge {
private final Loc source;
private final Loc target;
private final Collection guards;
private final Optional sync;
private final List updates;
private Edge(final Loc source, final Loc target, final Collection> guards,
final Optional sync, final List updates) {
this.source = checkNotNull(source);
this.target = checkNotNull(target);
this.guards = createGuards(guards);
this.sync = checkNotNull(sync);
this.updates = createUpdates(updates);
}
public Loc getSource() {
return source;
}
public Loc getTarget() {
return target;
}
public Collection getGuards() {
return guards;
}
public Optional getSync() {
return sync;
}
public List getUpdates() {
return updates;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy