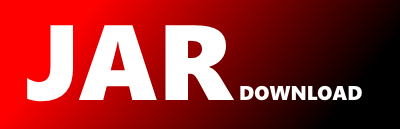
hu.bme.mit.theta.xta.XtaSystem Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of theta-xta Show documentation
Show all versions of theta-xta Show documentation
Xta subproject in the Theta model checking framework
The newest version!
/*
* Copyright 2024 Budapest University of Technology and Economics
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package hu.bme.mit.theta.xta;
import hu.bme.mit.theta.common.container.Containers;
import hu.bme.mit.theta.core.decl.VarDecl;
import hu.bme.mit.theta.core.model.MutableValuation;
import hu.bme.mit.theta.core.model.Valuation;
import hu.bme.mit.theta.core.type.LitExpr;
import hu.bme.mit.theta.core.type.rattype.RatType;
import hu.bme.mit.theta.xta.XtaProcess.Loc;
import java.util.*;
import static com.google.common.base.Preconditions.checkArgument;
import static com.google.common.base.Preconditions.checkNotNull;
import static com.google.common.collect.ImmutableList.toImmutableList;
public final class XtaSystem {
private final List processes;
private final Collection> dataVars;
private final Collection> clockVars;
private final MutableValuation initVal;
private final List unmodProcesses;
private final Collection> unmodDataVars;
private final Collection> unmodClockVars;
private XtaSystem() {
processes = new ArrayList<>();
dataVars = Containers.createSet();
clockVars = Containers.createSet();
initVal = new MutableValuation();
unmodProcesses = Collections.unmodifiableList(processes);
unmodDataVars = Collections.unmodifiableCollection(dataVars);
unmodClockVars = Collections.unmodifiableCollection(clockVars);
}
public static XtaSystem create() {
return new XtaSystem();
}
public List getProcesses() {
return unmodProcesses;
}
public Collection> getDataVars() {
return unmodDataVars;
}
public Collection> getClockVars() {
return unmodClockVars;
}
// TODO Return value is not immutable
public Valuation getInitVal() {
return initVal;
}
public List getInitLocs() {
return processes.stream().map(XtaProcess::getInitLoc).collect(toImmutableList());
}
////
public void addDataVar(final VarDecl> varDecl, final LitExpr> initValue) {
checkNotNull(varDecl);
checkNotNull(initValue);
checkArgument(!clockVars.contains(varDecl));
dataVars.add(varDecl);
initVal.put(varDecl, initValue);
}
public void addClockVar(final VarDecl varDecl) {
checkNotNull(varDecl);
checkArgument(!dataVars.contains(varDecl));
clockVars.add(varDecl);
}
public XtaProcess createProcess(final String name) {
final XtaProcess process = XtaProcess.create(this, name);
processes.add(process);
return process;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy