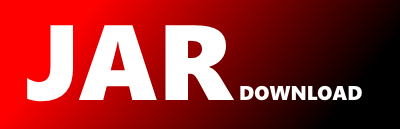
javaposse.jobdsl.dsl.helpers.ScmContext.groovy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of job-dsl-core Show documentation
Show all versions of job-dsl-core Show documentation
Javaposse jenkins job-dsl-core
package javaposse.jobdsl.dsl.helpers
import com.google.common.base.Preconditions
import hudson.plugins.perforce.PerforcePasswordEncryptor
import javaposse.jobdsl.dsl.JobManagement
import javaposse.jobdsl.dsl.WithXmlAction
import javaposse.jobdsl.dsl.helpers.scm.GitContext
import static javaposse.jobdsl.dsl.helpers.AbstractContextHelper.executeInContext
import static javaposse.jobdsl.dsl.helpers.publisher.PublisherContext.getValidCloneWorkspaceCriteria
class ScmContext implements Context {
boolean multiEnabled
List scmNodes = []
List withXmlActions = []
JobManagement jobManagement
ScmContext(multiEnabled = false, withXmlActions = [], jobManagement = null) {
this.multiEnabled = multiEnabled
this.withXmlActions = withXmlActions
this.jobManagement = jobManagement
}
// Package scope
ScmContext(Node singleNode, multiEnabled = false) {
this.multiEnabled = multiEnabled
scmNodes << singleNode // Safe since this is the constructor
}
/**
* Helper method for dealing with a single scm node
*/
def getScmNode() {
return scmNodes[0]
}
private validateMulti(){
Preconditions.checkState(scmNodes.size() < (multiEnabled?10:1), 'Outside "multiscm", only one SCM can be specified')
}
/**
* Generate configuration for Mercurial.
*
http://selenic.com/repo/hello
sample-module1 sample-module2
path-to-check-out-into
true
http://selenic.com/repo/hello/
*/
def hg(String url, String branch = null, Closure configure = null) {
validateMulti()
Preconditions.checkNotNull(url)
// TODO Validate url as a Mercurial url (e.g. http, https or ssh)
// TODO Attempt to update existing scm node
def nodeBuilder = new NodeBuilder()
Node scmNode = nodeBuilder.scm(class:'hudson.plugins.mercurial.MercurialSCM') {
source url
modules ''
clean false
}
scmNode.appendNode('branch', branch?:'')
// Apply Context
if (configure) {
WithXmlAction action = new WithXmlAction(configure)
action.execute(scmNode)
}
scmNodes << scmNode
}
/**
2
[email protected]:jenkinsci/job-dsl-plugin.git
**
false
false
false
false
false
false
false
false
false
Default
false
false
*/
def git(Closure gitClosure) {
validateMulti()
GitContext gitContext = new GitContext(withXmlActions, jobManagement)
executeInContext(gitClosure, gitContext)
if (gitContext.branches.empty) {
gitContext.branches << '**'
}
// TODO Attempt to update existing scm node
def nodeBuilder = new NodeBuilder()
Node gitNode = nodeBuilder.scm(class:'hudson.plugins.git.GitSCM') {
userRemoteConfigs(gitContext.remoteConfigs)
branches {
gitContext.branches.each { String branch ->
'hudson.plugins.git.BranchSpec' {
name(branch)
}
}
}
configVersion '2'
disableSubmodules 'false'
recursiveSubmodules 'false'
doGenerateSubmoduleConfigurations 'false'
authorOrCommitter 'false'
clean gitContext.clean
wipeOutWorkspace gitContext.wipeOutWorkspace
pruneBranches 'false'
remotePoll gitContext.remotePoll
ignoreNotifyCommit 'false'
//buildChooser class="hudson.plugins.git.util.DefaultBuildChooser"
gitTool 'Default'
//submoduleCfg 'class="list"'
if (gitContext.relativeTargetDir) {
relativeTargetDir gitContext.relativeTargetDir
}
if (gitContext.reference) {
reference gitContext.reference
}
//excludedRegions
//excludedUsers
//gitConfigName
//gitConfigEmail
skipTag !gitContext.createTag
//includedRegions
//scmName
if (gitContext.shallowClone) {
useShallowClone gitContext.shallowClone
}
}
if (gitContext.browser) {
gitNode.children().add(gitContext.browser)
}
if (gitContext.mergeOptions) {
gitNode.children().add(gitContext.mergeOptions)
}
// Apply Context
if (gitContext.withXmlClosure) {
WithXmlAction action = new WithXmlAction(gitContext.withXmlClosure)
action.execute(gitNode)
}
scmNodes << gitNode
}
/**
* @param url
* @param branch
* @param configure
* @return
*/
def git(String url, Closure configure = null) {
git(url, null, configure)
}
def git(String url, String branch, Closure configure = null) {
git {
remote {
delegate.url(url)
}
if (branch) {
delegate.branch(branch)
}
if (configure) {
delegate.configure(configure)
}
delegate.createTag()
}
}
def github(String ownerAndProject, String branch = null, String protocol = "https", Closure closure) {
github(ownerAndProject, branch, protocol, "github.com", closure)
}
def github(String ownerAndProject, String branch = null, String protocol = "https", String host = "github.com", Closure closure = null) {
git {
remote {
delegate.github(ownerAndProject, protocol, host)
}
if (branch) {
delegate.branch(branch)
}
if (closure) {
delegate.configure(closure)
}
}
}
/**
http://svn/repo
.
http://mycompany.com/viewvn/repo_name
OR
http://mycompany.com/viewvn/repo_name
my_root_module
*/
def svn(String svnUrl, Closure configure = null) {
svn(svnUrl, '.', configure)
}
def svn(String svnUrl, String localDir, Closure configure = null) {
Preconditions.checkNotNull(svnUrl)
Preconditions.checkNotNull(localDir)
validateMulti()
// TODO Validate url as a svn url (e.g. https or http)
// TODO Attempt to update existing scm node
def nodeBuilder = new NodeBuilder()
Node svnNode = nodeBuilder.scm(class:'hudson.scm.SubversionSCM') {
locations {
'hudson.scm.SubversionSCM_-ModuleLocation' {
remote "${svnUrl}"
local "${localDir}"
}
}
excludedRegions ''
includedRegions ''
excludedUsers ''
excludedRevprop ''
excludedCommitMessages ''
workspaceUpdater(class:'hudson.scm.subversion.UpdateUpdater')
}
// Apply Context
if (configure) {
WithXmlAction action = new WithXmlAction(configure)
action.execute(svnNode)
}
scmNodes << svnNode
}
/**
rolem
perforce:1666
builds-workspace
//depot/webapplication/...
//depot/Tools/build/...
noallwrite clobber nocompress unlocked nomodtime rmdir
p4
C:
C:\WINDOWS
false
false
false
false
false
false
false
true
false
false
false
false
true
false
-1
${basename}-${nodename}
false
false
false
true
*/
def p4(String viewspec, Closure configure = null) {
return p4(viewspec, 'rolem', '', configure)
}
def p4(String viewspec, String user, Closure configure = null) {
return p4(viewspec, user, '', configure)
}
def p4(String viewspec, String user, String password, Closure configure = null) {
Preconditions.checkNotNull(viewspec)
validateMulti()
// TODO Validate viewspec as valid viewspec
// TODO Attempt to update existing scm node
def nodeBuilder = new NodeBuilder()
PerforcePasswordEncryptor encryptor = new PerforcePasswordEncryptor();
String cleanPassword = encryptor.appearsToBeAnEncryptedPassword(password)?password:encryptor.encryptString(password)
Node p4Node = nodeBuilder.scm(class:'hudson.plugins.perforce.PerforceSCM') {
p4User user
p4Passwd cleanPassword
p4Port 'perforce:1666'
p4Client 'builds-${JOB_NAME}'
projectPath "${viewspec}"
projectOptions 'noallwrite clobber nocompress unlocked nomodtime rmdir'
p4Tool 'p4'
p4SysDrive 'C:'
p4SysRoot 'C:\\WINDOWS'
useClientSpec 'false'
forceSync 'false'
alwaysForceSync 'false'
dontUpdateServer 'false'
disableAutoSync 'false'
disableSyncOnly 'false'
useOldClientName 'false'
updateView 'true'
dontRenameClient 'false'
updateCounterValue 'false'
dontUpdateClient 'false'
exposeP4Passwd 'false'
wipeBeforeBuild 'true'
wipeRepoBeforeBuild 'false'
firstChange '-1'
slaveClientNameFormat '${basename}-${nodename}'
lineEndValue ''
useViewMask 'false'
useViewMaskForPolling 'false'
useViewMaskForSyncing 'false'
pollOnlyOnMaster 'true'
}
// Apply Context
if (configure) {
WithXmlAction action = new WithXmlAction(configure)
action.execute(p4Node)
}
scmNodes << p4Node
}
/**
*
* test-job
* Successful
*
*/
def cloneWorkspace(String parentProject, String criteriaArg = 'Any') {
Preconditions.checkNotNull(parentProject)
Preconditions.checkArgument(
validCloneWorkspaceCriteria.contains(criteriaArg),
"Clone Workspace Criteria needs to be one of these values: ${validCloneWorkspaceCriteria.join(',')}")
validateMulti()
scmNodes << NodeBuilder.newInstance().scm(class: 'hudson.plugins.cloneworkspace.CloneWorkspaceSCM') {
parentJobName(parentProject)
criteria(criteriaArg)
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy