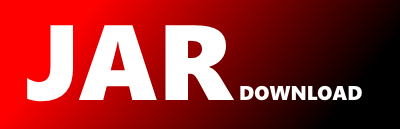
javaposse.jobdsl.dsl.helpers.publisher.PublisherContext.groovy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of job-dsl-core Show documentation
Show all versions of job-dsl-core Show documentation
Javaposse jenkins job-dsl-core
package javaposse.jobdsl.dsl.helpers.publisher
import javaposse.jobdsl.dsl.WithXmlAction
import javaposse.jobdsl.dsl.helpers.AbstractContextHelper
import javaposse.jobdsl.dsl.helpers.Context
import javaposse.jobdsl.dsl.helpers.common.DownstreamContext
class PublisherContext implements Context {
List publisherNodes = []
@Delegate
StaticAnalysisPublisherContext staticAnalysisPublisherHelper
PublisherContext() {
staticAnalysisPublisherHelper = new StaticAnalysisPublisherContext(publisherNodes)
}
PublisherContext(List publisherNodes) {
this.publisherNodes = publisherNodes
staticAnalysisPublisherHelper = new StaticAnalysisPublisherContext(this.publisherNodes)
}
/**
[email protected]
$PROJECT_DEFAULT_SUBJECT
$PROJECT_DEFAULT_CONTENT
false
false
false
true
$PROJECT_DEFAULT_SUBJECT
$PROJECT_DEFAULT_CONTENT
false
false
false
true
default
$DEFAULT_SUBJECT
$DEFAULT_CONTENT
* @return
* TODO Support list for recipients
* TODO Escape XML for all subject and content fields
*/
def extendedEmail(String recipients = null, Closure emailClosure = null) {
return extendedEmail(recipients, null, emailClosure)
}
def extendedEmail(String recipients, String subjectTemplate, Closure emailClosure = null) {
return extendedEmail(recipients, subjectTemplate, null, emailClosure)
}
def extendedEmail(String recipients, String subjectTemplate, String contentTemplate, Closure emailClosure = null) {
EmailContext emailContext = new EmailContext()
AbstractContextHelper.executeInContext(emailClosure, emailContext)
// Validate that we have the typical triggers, if nothing is provided
if (emailContext.emailTriggers.isEmpty()) {
emailContext.emailTriggers << new EmailContext.EmailTrigger('Failure')
emailContext.emailTriggers << new EmailContext.EmailTrigger('Success')
}
recipients = recipients != null ? recipients : '$DEFAULT_RECIPIENTS'
subjectTemplate = subjectTemplate ?: '$DEFAULT_SUBJECT'
contentTemplate = contentTemplate ?: '$DEFAULT_CONTENT'
// Now that the context has what we need
def nodeBuilder = NodeBuilder.newInstance()
def emailNode = nodeBuilder.'hudson.plugins.emailext.ExtendedEmailPublisher' {
recipientList recipients
contentType 'default'
defaultSubject subjectTemplate
defaultContent contentTemplate
attachmentsPattern ''
configuredTriggers {
emailContext.emailTriggers.each { EmailContext.EmailTrigger trigger ->
"hudson.plugins.emailext.plugins.trigger.${trigger.triggerShortName}Trigger" {
email {
recipientList trigger.recipientList
subject trigger.subject
body trigger.body
sendToDevelopers trigger.sendToDevelopers as String
sendToRequester trigger.sendToRequester as String
includeCulprits trigger.includeCulprits as String
sendToRecipientList trigger.sendToRecipientList as String
}
}
}
}
}
// Apply their overrides
if (emailContext.configureClosure) {
emailContext.configureClosure.resolveStrategy = Closure.DELEGATE_FIRST
WithXmlAction action = new WithXmlAction(emailContext.configureClosure)
action.execute(emailNode)
}
publisherNodes << emailNode
}
/**
[email protected]
false
true
*/
def mailer(String mailRecipients, Boolean dontNotifyEveryUnstableBuildBoolean = false, Boolean sendToIndividualsBoolean = false) {
def nodeBuilder = new NodeBuilder()
Node mailerNode = nodeBuilder.'hudson.tasks.Mailer' {
recipients(mailRecipients)
dontNotifyEveryUnstableBuild(dontNotifyEveryUnstableBuildBoolean)
sendToIndividuals(sendToIndividualsBoolean)
}
publisherNodes << mailerNode
}
/**
build/libs/*
build/libs/bad/*
false
false
Note: allowEmpty is not compatible with jenkins <= 1.480
* @param glob
* @param excludeGlob
* @param latestOnly
*/
def archiveArtifacts(Closure artifactsClosure) {
ArchiveArtifactsContext artifactsContext = new ArchiveArtifactsContext()
AbstractContextHelper.executeInContext(artifactsClosure, artifactsContext)
def nodeBuilder = new NodeBuilder()
Node archiverNode = nodeBuilder.'hudson.tasks.ArtifactArchiver' {
artifacts artifactsContext.patternValue
latestOnly artifactsContext.latestOnlyValue ? 'true' : 'false'
if (artifactsContext.allowEmptyValue != null) {
allowEmptyArchive artifactsContext.allowEmptyValue ? 'true' : 'false'
}
if (artifactsContext.excludesValue) {
excludes artifactsContext.excludesValue
}
}
publisherNodes << archiverNode
}
def archiveArtifacts(String glob, String excludeGlob = null, Boolean latestOnlyBoolean = false) {
archiveArtifacts {
pattern glob
exclude excludeGlob
latestOnly latestOnlyBoolean
}
}
/**
* Everything checked:
build/test/*.xml // Can be empty
true
// Empty if no extra publishers
// Allow claiming of failed tests
// Publish test attachments
*/
def archiveJunit(String glob, boolean retainLongStdout = false, boolean allowClaimingOfFailedTests = false, boolean publishTestAttachments = false) {
def nodeBuilder = new NodeBuilder()
Node archiverNode = nodeBuilder.'hudson.tasks.junit.JUnitResultArchiver' {
testResults glob
keepLongStdio retainLongStdout ? 'true' : 'false'
testDataPublishers {
if (allowClaimingOfFailedTests) {
'hudson.plugins.claim.ClaimTestDataPublisher' ''
}
if (publishTestAttachments) {
'hudson.plugins.junitattachments.AttachmentPublisher' ''
}
}
}
publisherNodes << archiverNode
}
/**
"target/*.exec"
"target/classes"
"src/main/java"
"*.class"
"*.Test*"
0
0
0
0
0
0
0
0
0
0
0
0
**/
def jacocoCodeCoverage(Closure jacocoClosure = null) {
JacocoContext jacocoContext = new JacocoContext()
AbstractContextHelper.executeInContext(jacocoClosure, jacocoContext)
def nodeBuilder = NodeBuilder.newInstance()
Node jacocoNode = nodeBuilder.'hudson.plugins.jacoco.JacocoPublisher' {
execPattern jacocoContext.execPattern
classPattern jacocoContext.classPattern
sourcePattern jacocoContext.sourcePattern
inclusionPattern jacocoContext.inclusionPattern
exclusionPattern jacocoContext.exclusionPattern
minimumInstructionCoverage jacocoContext.minimumInstructionCoverage
minimumBranchCoverage jacocoContext.minimumBranchCoverage
minimumComplexityCoverage jacocoContext.minimumComplexityCoverage
minimumLineCoverage jacocoContext.minimumLineCoverage
minimumMethodCoverage jacocoContext.minimumMethodCoverage
minimumClassCoverage jacocoContext.minimumClassCoverage
maximumInstructionCoverage jacocoContext.maximumInstructionCoverage
maximumBranchCoverage jacocoContext.maximumBranchCoverage
maximumComplexityCoverage jacocoContext.maximumComplexityCoverage
maximumLineCoverage jacocoContext.maximumLineCoverage
maximumMethodCoverage jacocoContext.maximumMethodCoverage
maximumClassCoverage jacocoContext.maximumClassCoverage
}
publisherNodes << jacocoNode
}
/**
Gradle Tests
build/reports/tests/
index.html
false
htmlpublisher-wrapper.html
*/
def publishHtml(Closure htmlReportContext) {
HtmlReportContext reportContext = new HtmlReportContext()
AbstractContextHelper.executeInContext(htmlReportContext, reportContext)
// Now that the context has what we need
def nodeBuilder = NodeBuilder.newInstance()
def htmlPublisherNode = nodeBuilder.'htmlpublisher.HtmlPublisher' {
reportTargets {
reportContext.targets.each { HtmlReportContext.HtmlPublisherTarget target ->
'htmlpublisher.HtmlPublisherTarget' {
// All fields can have a blank, odd.
reportName target.reportName
reportDir target.reportDir
reportFiles target.reportFiles
keepAll target.keepAll
wrapperName target.wrapperName
}
}
}
}
publisherNodes << htmlPublisherNode
}
/**
* With only the target specified:
[email protected]
false
ALL // all
or FAILURE_AND_FIXED // failure and fixed
or ANY_FAILURE // failure
or STATECHANGE_ONLY // change
false // Notify on build starts
false // Notify SCM committers
false // Notify SCM culprits
false // Notify upstream committers
false // Notify SCM fixers
// Channel Notification Message
// Summary + SCM change
or // Just Summary
or // Summary and build parameters
or // Summary, SCM changes and failed tests
ONLY_CONFIGURATIONS
*/
def publishJabber(String target, Closure jabberClosure = null) {
publishJabber(target, null, null, jabberClosure)
}
def publishJabber(String target, String strategyName, Closure jabberClosure = null) {
publishJabber(target, strategyName, null, jabberClosure)
}
def publishJabber(String targetsArg, String strategyName, String channelNotificationName, Closure jabberClosure = null) {
JabberContext jabberContext = new JabberContext()
jabberContext.strategyName = strategyName ?: 'ALL'
jabberContext.channelNotificationName = channelNotificationName ?: 'Default'
AbstractContextHelper.executeInContext(jabberClosure, jabberContext)
// Validate values
assert validJabberStrategyNames.contains(jabberContext.strategyName), "Jabber Strategy needs to be one of these values: ${validJabberStrategyNames.join(',')}"
assert validJabberChannelNotificationNames.contains(jabberContext.channelNotificationName), "Jabber Channel Notification name needs to be one of these values: ${validJabberChannelNotificationNames.join(',')}"
def nodeBuilder = NodeBuilder.newInstance()
def publishNode = nodeBuilder.'hudson.plugins.jabber.im.transport.JabberPublisher' {
targets {
targetsArg.split().each { target ->
def isGroup = target.startsWith('*')
def targetClean = isGroup ? target.substring(1) : target
'hudson.plugins.im.GroupChatIMMessageTarget' {
delegate.createNode('name', targetClean)
if (isGroup) {
notificationOnly 'false'
}
}
}
}
strategy jabberContext.strategyName
notifyOnBuildStart jabberContext.notifyOnBuildStart ? 'true' : 'false'
notifySuspects jabberContext.notifyOnBuildStart ? 'true' : 'false'
notifyCulprits jabberContext.notifyCulprits ? 'true' : 'false'
notifyFixers jabberContext.notifyFixers ? 'true' : 'false'
notifyUpstreamCommitters jabberContext.notifyUpstreamCommitters ? 'true' : 'false'
buildToChatNotifier('class': "hudson.plugins.im.build_notify.${jabberContext.channelNotificationName}BuildToChatNotifier")
matrixMultiplier 'ONLY_CONFIGURATIONS'
}
publisherNodes << publishNode
}
def validJabberStrategyNames = ['ALL', 'FAILURE_AND_FIXED', 'ANY_FAILURE', 'STATECHANGE_ONLY']
def validJabberChannelNotificationNames = ['Default', 'SummaryOnly', 'BuildParameters', 'PrintFailingTests']
/**
javadoc
api-sdk/*
true
*/
def publishScp(String site, Closure scpClosure) {
ScpContext scpContext = new ScpContext()
AbstractContextHelper.executeInContext(scpClosure, scpContext)
// Validate values
assert !scpContext.entries.isEmpty(), "Scp publish requires at least one entry"
def nodeBuilder = NodeBuilder.newInstance()
// TODO Possibility to update existing publish node
def publishNode = nodeBuilder.'be.certipost.hudson.plugin.SCPRepositoryPublisher' {
siteName site
entries {
scpContext.entries.each { ScpContext.ScpEntry entry ->
'be.certipost.hudson.plugin.Entry' {
filePath entry.destination
sourceFile entry.source
keepHierarchy entry.keepHierarchy ? 'true' : 'false'
}
}
}
}
publisherNodes << publishNode
}
/**
* Clone Workspace SCM
*
*
*
*
* Any
* TAR
* true
*
*/
def publishCloneWorkspace(String workspaceGlob, Closure cloneWorkspaceClosure) {
publishCloneWorkspace(workspaceGlob, '', 'Any', 'TAR', false, cloneWorkspaceClosure)
}
def publishCloneWorkspace(String workspaceGlob, String workspaceExcludeGlob, Closure cloneWorkspaceClosure) {
publishCloneWorkspace(workspaceGlob, workspaceExcludeGlob, 'Any', 'TAR', false, cloneWorkspaceClosure)
}
def publishCloneWorkspace(String workspaceGlob, String workspaceExcludeGlob, String criteria, String archiveMethod, Closure cloneWorkspaceClosure) {
publishCloneWorkspace(workspaceGlob, workspaceExcludeGlob, criteria, archiveMethod, false, cloneWorkspaceClosure)
}
def publishCloneWorkspace(String workspaceGlobArg, String workspaceExcludeGlobArg = '', String criteriaArg = 'Any', String archiveMethodArg = 'TAR', boolean overrideDefaultExcludesArg = false, Closure cloneWorkspaceClosure = null) {
CloneWorkspaceContext cloneWorkspaceContext = new CloneWorkspaceContext()
cloneWorkspaceContext.criteria = criteriaArg ?: 'Any'
cloneWorkspaceContext.archiveMethod = archiveMethodArg ?: 'TAR'
cloneWorkspaceContext.workspaceExcludeGlob = workspaceExcludeGlobArg ?: ''
cloneWorkspaceContext.overrideDefaultExcludes = overrideDefaultExcludesArg ?: false
AbstractContextHelper.executeInContext(cloneWorkspaceClosure, cloneWorkspaceContext)
// Validate values
assert validCloneWorkspaceCriteria.contains(cloneWorkspaceContext.criteria), "Clone Workspace Criteria needs to be one of these values: ${validCloneWorkspaceCriteria.join(',')}"
assert validCloneWorkspaceArchiveMethods.contains(cloneWorkspaceContext.archiveMethod), "Clone Workspace Archive Method needs to be one of these values: ${validCloneWorkspaceArchiveMethods.join(',')}"
def nodeBuilder = NodeBuilder.newInstance()
def publishNode = nodeBuilder.'hudson.plugins.cloneworkspace.CloneWorkspacePublisher' {
workspaceGlob workspaceGlobArg
workspaceExcludeGlob cloneWorkspaceContext.workspaceExcludeGlob
criteria cloneWorkspaceContext.criteria
archiveMethod cloneWorkspaceContext.archiveMethod
overrideDefaultExcludes cloneWorkspaceContext.overrideDefaultExcludes
}
publisherNodes << publishNode
}
static List validCloneWorkspaceCriteria = ['Any', 'Not Failed', 'Successful']
def validCloneWorkspaceArchiveMethods = ['TAR', 'ZIP']
/**
* Downstream build
*
DSL-Tutorial-1-Test
SUCCESS
0
BLUE
// or
UNSTABLE 1 YELLOW
// or
FAILURE 2 RED
*/
def downstream(String projectName, String thresholdName = 'SUCCESS') {
assert DownstreamContext.THRESHOLD_COLOR_MAP.containsKey(thresholdName), "thresholdName must be one of these values ${DownstreamContext.THRESHOLD_COLOR_MAP.keySet().join(',')}"
def nodeBuilder = new NodeBuilder()
Node publishNode = nodeBuilder.'hudson.tasks.BuildTrigger' {
childProjects projectName
threshold {
delegate.createNode('name', thresholdName)
ordinal DownstreamContext.THRESHOLD_ORDINAL_MAP[thresholdName]
color DownstreamContext.THRESHOLD_COLOR_MAP[thresholdName]
}
}
publisherNodes << publishNode
}
/**
Trigger parameterized build on other projects.
// Current build parameters
// Parameters from properties file
some.properties
// Pass-through Git commit that was built
false
// Predefined properties
prop1=value1
prop2=value2
// Restrict matrix execution to a subset
label=="${TARGET}"
// Subversion revision
NEBULA-ubuntu-packaging-plugin
SUCCESS
false
DSL-Tutorial-1-Test
SUCCESS // SUCCESS, UNSTABLE, UNSTABLE_OR_BETTER, UNSTABLE_OR_WORSE, FAILED, ALWAYS
false
*/
def downstreamParameterized(Closure downstreamClosure) {
DownstreamContext downstreamContext = new DownstreamContext()
AbstractContextHelper.executeInContext(downstreamClosure, downstreamContext)
def publishNode = downstreamContext.createDownstreamNode(false)
publisherNodes << publishNode
}
def violations(Closure violationsClosure = null) {
violations(100, violationsClosure)
}
def violations(int perFileDisplayLimit, Closure violationsClosure = null) {
ViolationsContext violationsContext = new ViolationsContext()
violationsContext.perFileDisplayLimit = perFileDisplayLimit
AbstractContextHelper.executeInContext(violationsClosure, violationsContext)
def nodeBuilder = NodeBuilder.newInstance()
def publishNode = nodeBuilder.'hudson.plugins.violations.ViolationsPublisher'(plugin: '[email protected]') {
config {
suppressions(class: "tree-set") {
'no-comparator'()
}
typeConfigs {
'no-comparator'()
violationsContext.entries.each { String key, ViolationsContext.ViolationsEntry violationsEntry ->
entry {
string(key)
'hudson.plugins.violations.TypeConfig' {
type(key)
// These values are protected from ever being null or empty.
min(violationsEntry.min.toString())
max(violationsEntry.max.toString())
unstable(violationsEntry.unstable.toString())
usePattern(violationsEntry.pattern ? 'true' : 'false')
pattern(violationsEntry.pattern ?: '')
}
}
}
}
limit(violationsContext.perFileDisplayLimit.toString())
sourcePathPattern(violationsContext.sourcePathPattern ?: '')
fauxProjectPath(violationsContext.fauxProjectPath ?: '')
encoding(violationsContext.sourceEncoding ?: 'default')
}
}
publisherNodes << publishNode
}
/*
*/
def chucknorris() {
def nodeBuilder = NodeBuilder.newInstance()
def publishNode = nodeBuilder.'hudson.plugins.chucknorris.CordellWalkerRecorder' {
'factGenerator' ''
}
publisherNodes << publishNode
}
def irc(Closure ircClosure) {
IrcContext ircContext = new IrcContext()
AbstractContextHelper.executeInContext(ircClosure, ircContext)
def nodeBuilder = NodeBuilder.newInstance()
def publishNode = nodeBuilder.'hudson.plugins.ircbot.IrcPublisher' {
targets {
ircContext.channels.each { IrcContext.IrcPublisherChannel channel ->
'hudson.plugins.im.GroupChatIMMessageTarget' {
delegate.createNode('name', channel.name)
password channel.password
notificationOnly channel.notificationOnly ? 'true' : 'false'
}
}
}
strategy ircContext.strategy
notifyOnBuildStart ircContext.notifyOnBuildStarts ? 'true' : 'false'
notifySuspects ircContext.notifyScmCommitters ? 'true' : 'false'
notifyCulprits ircContext.notifyScmCulprits ? 'true' : 'false'
notifyFixers ircContext.notifyScmFixers ? 'true' : 'false'
notifyUpstreamCommitters ircContext.notifyUpstreamCommitters ? 'true' : 'false'
def className = "hudson.plugins.im.build_notify.${ircContext.notificationMessage}BuildToChatNotifier"
buildToChatNotifier(class: className)
}
publisherNodes << publishNode
}
def cobertura(String reportFile, Closure coberturaClosure = null) {
CoberturaContext coberturaContext = new CoberturaContext()
AbstractContextHelper.executeInContext(coberturaClosure, coberturaContext)
publisherNodes << NodeBuilder.newInstance().'hudson.plugins.cobertura.CoberturaPublisher' {
coberturaReportFile(reportFile)
onlyStable(coberturaContext.onlyStable)
failUnhealthy(coberturaContext.failUnhealthy)
failUnstable(coberturaContext.failUnstable)
autoUpdateHealth(coberturaContext.autoUpdateHealth)
autoUpdateStability(coberturaContext.autoUpdateStability)
zoomCoverageChart(coberturaContext.zoomCoverageChart)
failNoReports(coberturaContext.failNoReports)
['healthyTarget', 'unhealthyTarget', 'failingTarget'].each { targetName ->
"$targetName" {
targets(class: "enum-map", 'enum-type': "hudson.plugins.cobertura.targets.CoverageMetric") {
coberturaContext.targets.values().each { target ->
entry {
'hudson.plugins.cobertura.targets.CoverageMetric' target.targetType
'int' target."$targetName"
}
}
}
}
}
sourceEncoding(coberturaContext.sourceEncoding)
}
}
/**
*
*/
def allowBrokenBuildClaiming() {
publisherNodes << NodeBuilder.newInstance().'hudson.plugins.claim.ClaimPublisher'()
}
/**
* Configures Fingerprinting
*
*
* **
* true
*
*
*/
def fingerprint(String targets, boolean recordBuildArtifacts = false) {
publisherNodes << NodeBuilder.newInstance().'hudson.tasks.Fingerprinter' {
delegate.targets(targets ?: '')
delegate.recordBuildArtifacts(recordBuildArtifacts)
}
}
/**
* Configures the Description Setter Plugin
*
*
*
* foo
* bar
* Hello
* World
* false
*
*/
def buildDescription(String regularExpression, String description = '', String regularExpressionForFailed = '', String descriptionForFailed = '', boolean multiConfigurationBuild = false) {
publisherNodes << NodeBuilder.newInstance().'hudson.plugins.descriptionsetter.DescriptionSetterPublisher' {
regexp(regularExpression)
regexpForFailed(regularExpressionForFailed)
delegate.description(description)
if (descriptionForFailed) {
delegate.descriptionForFailed(descriptionForFailed)
}
setForMatrix(multiConfigurationBuild)
}
}
/**
* Configures the Jenkins Text Finder plugin
*
*
*
* *.txt
*
* false
* false
* false
*
*/
def textFinder(String regularExpression, String fileSet = '', boolean alsoCheckConsoleOutput = false, boolean succeedIfFound = false, unstableIfFound = false) {
publisherNodes << NodeBuilder.newInstance().'hudson.plugins.textfinder.TextFinderPublisher' {
if (fileSet) delegate.fileSet(fileSet)
delegate.regexp(regularExpression)
delegate.alsoCheckConsoleOutput(alsoCheckConsoleOutput)
delegate.succeedIfFound(succeedIfFound)
delegate.unstableIfFound(unstableIfFound)
}
}
/**
* Configures the Jenkins Post Build Task plugin
*
*
*
*
*
*
*
* BUILD SUCCESSFUL
* AND
*
*
* false
* false
*
*
*
*
*/
def postBuildTask(Closure postBuildClosure) {
PostBuildTaskContext postBuildContext = new PostBuildTaskContext()
AbstractContextHelper.executeInContext(postBuildClosure, postBuildContext)
publisherNodes << NodeBuilder.newInstance().'hudson.plugins.postbuildtask.PostbuildTask' {
tasks {
postBuildContext.tasks.each { PostBuildTaskContext.PostBuildTask task ->
'hudson.plugins.postbuildtask.TaskProperties' {
logTexts {
'hudson.plugins.postbuildtask.LogProperties' {
logText(task.logText)
operator(task.operator)
}
}
EscalateStatus(task.escalateStatus)
RunIfJobSuccessful(task.runIfJobSuccessful)
script(task.script)
}
}
}
}
}
/**
* Configures Aggregate Downstream Test Results. Pass no args or null for jobs (first arg) to
* automatically aggregate downstream test results. Pass in comma-delimited list for first arg to manually choose jobs.
* Second argument is optional and sets whether failed builds are included.
*
*
* ...
*
* some-downstream-test
* false
*
* ...
*
*/
def aggregateDownstreamTestResults(String jobs = null, boolean includeFailedBuilds = false) {
publisherNodes << NodeBuilder.newInstance().'hudson.tasks.test.AggregatedTestResultPublisher' {
if (jobs) {
delegate.jobs(jobs)
}
delegate.includeFailedBuilds(includeFailedBuilds)
}
}
def static enum Behavior {
DoNothing(0),
MarkUnstable(1),
MarkFailed(2)
final int value
Behavior(int value) {
this.value = value
}
}
/**
* Configures the Groovy Postbuild script plugin
*
*
*
*
* script
*
* 0
*
*/
def groovyPostBuild(String script, Behavior behavior = Behavior.DoNothing) {
publisherNodes << NodeBuilder.newInstance().'org.jvnet.hudson.plugins.groovypostbuild.GroovyPostbuildRecorder' {
delegate.groovyScript(script)
delegate.behavior(behavior.value)
}
}
/**
* Configures the Javadoc Plugin, used to archive Javadoc artifacts.
*
* Uses the Jenkins Javadoc Plugin: https://wiki.jenkins-ci.org/display/JENKINS/Javadoc+Plugin
*
*
*
* foo
* false
*
*
*/
def archiveJavadoc(Closure javadocClosure = null) {
JavadocContext javadocContext = new JavadocContext()
AbstractContextHelper.executeInContext(javadocClosure, javadocContext)
def nodeBuilder = NodeBuilder.newInstance()
Node javadocNode = nodeBuilder.'hudson.tasks.JavadocArchiver' {
javadocDir javadocContext.javadocDir
keepAll javadocContext.keepAll
}
publisherNodes << javadocNode
}
/**
* Configures the Associated Files plugin to associate archived files from
* outside Jenkins proper.
*
* See https://wiki.jenkins-ci.org/display/JENKINS/Associated+Files+Plugin
*
*
*
* /mnt/jenkins-staging/binary-staging/${JOB_NAME}-${BUILD_ID}
*
*
*/
def associatedFiles(String files = null) {
publisherNodes << NodeBuilder.newInstance().'org.jenkinsci.plugins.associatedfiles.AssociatedFilesPublisher' {
delegate.associatedFiles(files)
}
}
/**
* Configures the Emma Code Coverage plugin
*
*
*
* coverage-results/coverage.xml
*
* 0
* 100
* 0
* 70
* 0
* 80
* 0
* 80
* 0
* 0
*
*
*/
def emma(String fileSet = '', Closure emmaClosure = null) {
EmmaContext emmaContext = new EmmaContext()
AbstractContextHelper.executeInContext(emmaClosure, emmaContext)
publisherNodes << NodeBuilder.newInstance().'hudson.plugins.emma.EmmaPublisher' {
includes(fileSet)
healthReports {
minClass(emmaContext.classRange.getFrom())
maxClass(emmaContext.classRange.getTo())
minMethod(emmaContext.methodRange.getFrom())
maxMethod(emmaContext.methodRange.getTo())
minBlock(emmaContext.blockRange.getFrom())
maxBlock(emmaContext.blockRange.getTo())
minLine(emmaContext.lineRange.getFrom())
maxLine(emmaContext.lineRange.getTo())
minCondition(emmaContext.conditionRange.getFrom())
maxCondition(emmaContext.conditionRange.getTo())
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy