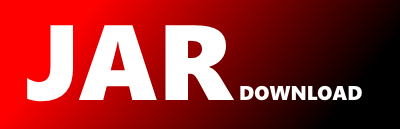
javaposse.jobdsl.dsl.helpers.publisher.StaticAnalysisPublisherContext.groovy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of job-dsl-core Show documentation
Show all versions of job-dsl-core Show documentation
Javaposse jenkins job-dsl-core
package javaposse.jobdsl.dsl.helpers.publisher
import javaposse.jobdsl.dsl.helpers.AbstractContextHelper
/**
* This class adds support for the Publishers from
* Static Code Analysis Plugins
*
* The class {@link javaposse.jobdsl.dsl.helpers.publisher.PublisherContext} uses this class
* as a delegate to make the corresponding methods appear as methods of the publishers
Closure.
*
* Every Publisher has the following common set of xml, which is not added to the corresponding xml struckture in the javadoc
* of the method:
*
* {@code
*
*
* low
* <>
*
* false
* false
* false
*
* 1
* 2
* 3
* 4
* 9
* 10
* 11
* 12
* 5
* 6
* 7
* 8
* 13
* 14
* 15
* 16
*
* false
* false
* true
* }
*
*/
class StaticAnalysisPublisherContext {
List publisherNodes
StaticAnalysisPublisherContext(List publisherNodes) {
this.publisherNodes = publisherNodes
}
/**
* Configures the findbugs publisher
*
*
* {@code
*
* ...
* **/findbugsXml.xml
* false
*
* }
*
**/
def findbugs(String pattern, boolean isRankActivated = false, Closure staticAnalysisClosure = null) {
StaticAnalysisContext staticAnalysisContext = new StaticAnalysisContext()
AbstractContextHelper.executeInContext(staticAnalysisClosure, staticAnalysisContext)
publisherNodes << NodeBuilder.newInstance().'hudson.plugins.findbugs.FindBugsPublisher' {
addStaticAnalysisContextAndPattern(delegate, staticAnalysisContext, pattern)
delegate.isRankActivated(isRankActivated)
}
}
/**
* Configures the PMD Publisher
*
*
* {@code
*
* ...
* pmd.xml
*
* }
*
*/
def pmd(String pattern, Closure staticAnalysisClosure = null) {
publisherNodes << createDefaultStaticAnalysisNode(
'hudson.plugins.pmd.PmdPublisher',
staticAnalysisClosure,
pattern
)
}
/**
* Configures the Checkstyle Publisher
*
*
* {@code
*
* ...
* checkstyle.xml
*
* }
*
*/
def checkstyle(String pattern, Closure staticAnalysisClosure = null) {
publisherNodes << createDefaultStaticAnalysisNode(
'hudson.plugins.checkstyle.CheckStylePublisher',
staticAnalysisClosure,
pattern
)
}
/**
* Configures the JsHint checkstyle Publisher
*
*
* {@code
*
* ...
* checkstyle.xml
*
* }
*
*/
def jshint(String pattern, Closure staticAnalysisClosure = null) {
publisherNodes << createDefaultStaticAnalysisNode(
'hudson.plugins.jshint.CheckStylePublisher',
staticAnalysisClosure,
pattern
)
}
/**
* Configures the DRY Publisher
*
*
* {@code
*
* ...
* cpd.xml
* 85
* 13
*
* }
*
*/
def dry(String pattern, highThreshold = 50, normalThreshold = 25, Closure staticAnalysisClosure = null) {
StaticAnalysisContext staticAnalysisContext = new StaticAnalysisContext()
AbstractContextHelper.executeInContext(staticAnalysisClosure, staticAnalysisContext)
publisherNodes << NodeBuilder.newInstance().'hudson.plugins.dry.DryPublisher' {
addStaticAnalysisContextAndPattern(delegate, staticAnalysisContext, pattern)
delegate.highThreshold(highThreshold)
delegate.normalThreshold(normalThreshold)
}
}
/**
* Configures the Task Scanner Publisher
*
*
* {@code
*
* ...
* *.java
* FIXM
* TOD
* LOW
* true
* *.groovy
*
* }
*
*/
def tasks(String pattern, excludePattern = '', high = '', normal = '', low = '', ignoreCase = false, Closure staticAnalysisClosure = null) {
StaticAnalysisContext staticAnalysisContext = new StaticAnalysisContext()
AbstractContextHelper.executeInContext(staticAnalysisClosure, staticAnalysisContext)
publisherNodes << NodeBuilder.newInstance().'hudson.plugins.tasks.TasksPublisher' {
addStaticAnalysisContextAndPattern(delegate, staticAnalysisContext, pattern)
delegate.high(high)
delegate.normal(normal)
delegate.low(low)
delegate.ignoreCase(ignoreCase)
delegate.excludePattern(excludePattern)
}
}
/**
* Configures the CCM Publisher
*
*
* {@code
*
* ...
* ccm.xml
*
* }
*
*/
def ccm(String pattern, Closure staticAnalysisClosure = null) {
publisherNodes << createDefaultStaticAnalysisNode(
'hudson.plugins.ccm.CcmPublisher',
staticAnalysisClosure,
pattern
)
}
/**
* Configures the Android Lint Publisher
*
*
* {@code
*
* ...
* lint.xml
*
* }
*
*/
def androidLint(String pattern, Closure staticAnalysisClosure = null) {
publisherNodes << createDefaultStaticAnalysisNode(
'org.jenkinsci.plugins.android__lint.LintPublisher',
staticAnalysisClosure,
pattern
)
}
/**
* Configures the OWASP Dependency-Check Publisher
*
*
* {@code
*
* ...
* dep.xml
*
* }
*
*/
def dependencyCheck(String pattern, Closure staticAnalysisClosure = null) {
publisherNodes << createDefaultStaticAnalysisNode(
'org.jenkinsci.plugins.DependencyCheck.DependencyCheckPublisher',
staticAnalysisClosure,
pattern
)
}
/**
* Configures the Compiler Warnings Publisher
*
*
* {@code
*
* ...
* .*include.*
* .*exclude.*
*
*
* Java Compiler (javac)
*
*
*
*
* *.log
* Java Compiler (javac)
*
*
*
* }
*
*/
def warnings(List consoleParsers, Map parserConfigurations = [:], Closure warningsClosure = null) {
WarningsContext warningsContext = new WarningsContext()
AbstractContextHelper.executeInContext(warningsClosure, warningsContext)
def nodeBuilder = NodeBuilder.newInstance()
publisherNodes << nodeBuilder.'hudson.plugins.warnings.WarningsPublisher' {
addStaticAnalysisContext(delegate, warningsContext)
includePattern(warningsContext.includePattern)
excludePattern(warningsContext.excludePattern)
nodeBuilder.consoleParsers {
(consoleParsers ?: []).each { name ->
nodeBuilder.'hudson.plugins.warnings.ConsoleParser' {
parserName(name)
}
}
}
nodeBuilder.parserConfigurations {
(parserConfigurations ?: [:]).each { name, filePattern ->
nodeBuilder.'hudson.plugins.warnings.ParserConfiguration' {
pattern(filePattern)
parserName(name)
}
}
}
}
}
private createDefaultStaticAnalysisNode(String publisherClassName, Closure staticAnalysisClosure, String pattern) {
StaticAnalysisContext staticAnalysisContext = new StaticAnalysisContext()
AbstractContextHelper.executeInContext(staticAnalysisClosure, staticAnalysisContext)
NodeBuilder.newInstance()."${publisherClassName}" {
addStaticAnalysisContextAndPattern(delegate, staticAnalysisContext, pattern)
}
}
private def addStaticAnalysisContext(nodeBuilder, StaticAnalysisContext context) {
nodeBuilder.with {
healthy(context.healthy)
unHealthy(context.unHealthy)
thresholdLimit(context.thresholdLimit)
defaultEncoding(context.defaultEncoding)
canRunOnFailed(context.canRunOnFailed)
useStableBuildAsReference(context.useStableBuildAsReference)
useDeltaValues(context.useDeltaValues)
thresholds {
context.thresholdMap.each { threshold, values ->
values.each { value, num ->
nodeBuilder."${threshold}${value.capitalize()}"(num)
}
}
}
shouldDetectModules(context.shouldDetectModules)
dontComputeNew(context.dontComputeNew)
doNotResolveRelativePaths(context.doNotResolveRelativePaths)
}
}
private def addStaticAnalysisPattern(nodeBuilder, String pattern) {
nodeBuilder.pattern(pattern)
}
private def addStaticAnalysisContextAndPattern(nodeBuilder, StaticAnalysisContext context, String pattern) {
addStaticAnalysisContext(nodeBuilder, context)
addStaticAnalysisPattern(nodeBuilder, pattern)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy