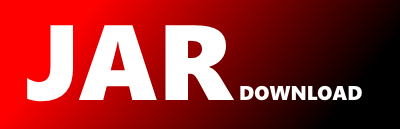
javaposse.jobdsl.dsl.helpers.step.AbstractStepContext.groovy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of job-dsl-core Show documentation
Show all versions of job-dsl-core Show documentation
Javaposse jenkins job-dsl-core
package javaposse.jobdsl.dsl.helpers.step
import com.google.common.base.Preconditions
import javaposse.jobdsl.dsl.WithXmlAction
import javaposse.jobdsl.dsl.helpers.AbstractContextHelper
import javaposse.jobdsl.dsl.helpers.Context
import javaposse.jobdsl.dsl.helpers.common.DownstreamContext
import static javaposse.jobdsl.dsl.helpers.common.MavenContext.LocalRepositoryLocation.LocalToWorkspace
class AbstractStepContext implements Context {
List stepNodes = []
AbstractStepContext(List stepNodes = []) {
this.stepNodes = stepNodes
}
/**
echo Hello
*/
def shell(String commandStr) {
def nodeBuilder = new NodeBuilder()
stepNodes << nodeBuilder.'hudson.tasks.Shell' {
'command' commandStr
}
}
/**
echo Hello from Windows
*/
def batchFile(String commandStr) {
def nodeBuilder = new NodeBuilder()
stepNodes << nodeBuilder.'hudson.tasks.BatchFile' {
'command' commandStr
}
}
def gradle(String tasksArg){
gradle(tasksArg,null,null)
}
def gradle(String tasksArg=null, String switchesArg=null, Closure gradleClosure) {
GradleContext gradleContext = new GradleContext()
if(gradleClosure!=null){
AbstractContextHelper.executeInContext(gradleClosure, gradleContext)
}
if(switchesArg){
gradleContext.switches = switchesArg
}
if(tasksArg){
gradleContext.tasks = tasksArg
}
def nodeBuilder = new NodeBuilder()
def gradleNode = nodeBuilder.'hudson.plugins.gradle.Gradle' {
description gradleContext.description
switches gradleContext.switches
tasks gradleContext.tasks
rootBuildScriptDir gradleContext.rootBuildScriptDir
buildFile gradleContext.buildFile
useWrapper gradleContext.useWrapper.toString()
if(gradleContext.fromRootBuildScriptDir!=null){
fromRootBuildScriptDir gradleContext.fromRootBuildScriptDir.toString()
}
if(gradleContext.makeExecutable!=null){
makeExecutable gradleContext.makeExecutable.toString()
}
if(gradleContext.gradleName != null){
gradleName gradleContext.gradleName
}
}
stepNodes << gradleNode
}
/**
-Dtiming-multiple=5 -P${Status}=true -I ${WORKSPACE}/netflix-oss.gradle ${Option}
clean${Task}
true
*/
def gradle(String tasksArg, String switchesArg, Boolean useWrapperArg=null, Closure configure=null) {
def nodeBuilder = new NodeBuilder()
def gradleNode = nodeBuilder.'hudson.plugins.gradle.Gradle' {
description ''
switches switchesArg?:''
tasks tasksArg?:''
rootBuildScriptDir ''
buildFile ''
useWrapper useWrapperArg==null?'true':useWrapperArg.toString()
wrapperScript ''
}
// Apply Context
if (configure) {
WithXmlAction action = new WithXmlAction(configure)
action.execute(gradleNode)
}
stepNodes << gradleNode
}
/**
SBT 0.12.3
-XX:+CMSClassUnloadingEnabled -XX:MaxPermSize=512M -Dfile.encoding=UTF-8 -Xmx2G -Xms512M
-Dsbt.log.noformat=true
clean update "env development" test dist publish
*/
def sbt(String sbtNameArg, String actionsArg = null, String sbtFlagsArg=null, String jvmFlagsArg=null, String subdirPathArg=null, Closure configure = null) {
def nodeBuilder = new NodeBuilder()
def attributes = [plugin:'[email protected]']
def sbtNode = nodeBuilder.'org.jvnet.hudson.plugins.SbtPluginBuilder'(attributes) {
name Preconditions.checkNotNull(sbtNameArg, "Please provide the name of the SBT to use" as Object)
jvmFlags jvmFlagsArg?:''
sbtFlags sbtFlagsArg?:''
actions actionsArg?:''
subdirPath subdirPathArg?:''
}
// Apply Context
if (configure) {
WithXmlAction action = new WithXmlAction(configure)
action.execute(sbtNode)
}
stepNodes << sbtNode
}
/**
sbt-template.groovy
false
false
IGNORE
*/
def dsl(Closure configure = null) {
DslContext context = new DslContext()
AbstractContextHelper.executeInContext(configure, context)
buildDslNode(context)
}
def dsl(String scriptText, String removedJobAction = null, boolean ignoreExisting = false) {
DslContext ctx = new DslContext()
ctx.text(scriptText)
if (removedJobAction) {
ctx.removeAction(removedJobAction)
}
ctx.ignoreExisting = ignoreExisting
buildDslNode(ctx)
}
def dsl(Collection externalScripts, String removedJobAction = null, boolean ignoreExisting = false) {
DslContext ctx = new DslContext()
ctx.external(externalScripts.toArray(new String[0]))
if (removedJobAction) {
ctx.removeAction(removedJobAction)
}
ctx.ignoreExisting = ignoreExisting
buildDslNode(ctx)
}
private void buildDslNode(context) {
def nodeBuilder = new NodeBuilder()
def dslNode = nodeBuilder.'javaposse.jobdsl.plugin.ExecuteDslScripts' {
targets context.targets
usingScriptText context.useScriptText()
scriptText context.scriptText
ignoreExisting context.ignoreExisting
removedJobAction context.removedJobAction.name()
}
stepNodes << dslNode
}
/**
target
Ant 1.8
-Xmx1g -XX:MaxPermSize=128M -Dorg.apache.jasper.compiler.Parser.STRICT_QUOTE_ESCAPING=false
build.xml
test.jvmargs=-Xmx=1g
test.maxmemory=2g
multiline=true
Empty:
(Default)
*/
def ant(Closure antClosure = null) {
ant(null, null, null, antClosure)
}
def ant(String targetsStr, Closure antClosure = null) {
ant(targetsStr, null, null, antClosure)
}
def ant(String targetsStr, String buildFileStr, Closure antClosure = null) {
ant(targetsStr, buildFileStr, null, antClosure)
}
def ant(String targetsArg, String buildFileArg, String antInstallation, Closure antClosure = null) {
AntContext antContext = new AntContext()
AbstractContextHelper.executeInContext(antClosure, antContext)
def targetList = []
if (targetsArg) {
targetList.addAll targetsArg.contains('\n') ? targetsArg.split('\n') : targetsArg.split(' ')
}
targetList.addAll antContext.targets
// Build File
if (!buildFileArg && antContext.buildFile) { // Fall back to context
buildFileArg = antContext.buildFile
}
def antOptsList = antContext.antOpts
if(!antInstallation) {
antInstallation = antContext.antName?:'(Default)'
}
def propertiesList = []
propertiesList += antContext.props
def nodeBuilder = NodeBuilder.newInstance()
def antNode = nodeBuilder.'hudson.tasks.Ant' {
targets targetList.join(' ')
antName antInstallation
if (antOptsList) {
antOpts antOptsList.join('\n')
}
if (buildFileArg) {
buildFile buildFileArg
}
}
if(propertiesList) {
antNode.appendNode('properties', propertiesList.join('\n'))
}
stepNodes << antNode
}
/**
Command
(Default)
*/
def groovyCommand(String command, Closure groovyClosure = null) {
groovy(command, true, null, groovyClosure)
}
def groovyCommand(String command, String groovyName, Closure groovyClosure = null) {
groovy(command, true, groovyName, groovyClosure)
}
/**
acme.groovy
(Default)
*/
def groovyScriptFile(String fileName, Closure groovyClosure = null) {
groovy(fileName, false, null, groovyClosure)
}
def groovyScriptFile(String fileName, String groovyName, Closure groovyClosure = null) {
groovy(fileName, false, groovyName, groovyClosure)
}
private def groovyScriptSource(String commandOrFileName, boolean isCommand) {
def nodeBuilder = new NodeBuilder()
nodeBuilder.scriptSource(class: "hudson.plugins.groovy.${isCommand ? 'String' : 'File'}ScriptSource") {
if (isCommand) {
command commandOrFileName
} else {
scriptFile commandOrFileName
}
}
}
private def groovy(String commandOrFileName, boolean isCommand, String groovyInstallation, Closure groovyClosure) {
def groovyContext = new GroovyContext()
AbstractContextHelper.executeInContext(groovyClosure, groovyContext)
def groovyNode = NodeBuilder.newInstance().'hudson.plugins.groovy.Groovy' {
groovyName groovyInstallation ?: groovyContext.groovyInstallation ?: '(Default)'
parameters groovyContext.groovyParams.join('\n')
scriptParameters groovyContext.scriptParams.join('\n')
javaOpts groovyContext.javaOpts.join(' ')
classPath groovyContext.classpathEntries.join(File.pathSeparator)
}
groovyNode.append(groovyScriptSource(commandOrFileName, isCommand))
groovyNode.appendNode('properties', groovyContext.props.join('\n'))
stepNodes << groovyNode
}
/**
System Groovy
*/
def systemGroovyCommand(String command, Closure systemGroovyClosure = null) {
systemGroovy(command, true, systemGroovyClosure)
}
/**
System Groovy
*/
def systemGroovyScriptFile(String fileName, Closure systemGroovyClosure = null) {
systemGroovy(fileName, false, systemGroovyClosure)
}
private def systemGroovy(String commandOrFileName, boolean isCommand, Closure systemGroovyClosure) {
def systemGroovyContext = new SystemGroovyContext()
AbstractContextHelper.executeInContext(systemGroovyClosure, systemGroovyContext)
def systemGroovyNode = NodeBuilder.newInstance().'hudson.plugins.groovy.SystemGroovy' {
bindings systemGroovyContext.bindings.collect({ key, value -> "${key}=${value}" }).join('\n')
classpath systemGroovyContext.classpathEntries.join(File.pathSeparator)
}
systemGroovyNode.append(groovyScriptSource(commandOrFileName, isCommand))
stepNodes << systemGroovyNode
}
/**
*
* install
* (Default)
* -Xmx512m
* pom.xml
* false
*
*/
def maven(Closure closure) {
MavenContext mavenContext = new MavenContext()
AbstractContextHelper.executeInContext(closure, mavenContext)
Node mavenNode = new NodeBuilder().'hudson.tasks.Maven' {
targets mavenContext.goals.join(' ')
mavenName mavenContext.mavenInstallation
jvmOptions mavenContext.mavenOpts.join(' ')
if (mavenContext.rootPOM) {
pom mavenContext.rootPOM
}
usePrivateRepository mavenContext.localRepositoryLocation == LocalToWorkspace ? 'true' : 'false'
}
// Apply Context
if (mavenContext.configureBlock) {
WithXmlAction action = new WithXmlAction(mavenContext.configureBlock)
action.execute(mavenNode)
}
stepNodes << mavenNode
}
def maven(String targetsArg = null, String pomArg = null, Closure configure = null) {
maven {
delegate.goals(targetsArg)
delegate.rootPOM(pomArg)
delegate.configure(configure)
}
}
/**
(Default)
false
true
false
*/
def grails(Closure grailsClosure) {
grails null, false, grailsClosure
}
def grails(String targetsArg, Closure grailsClosure) {
grails targetsArg, false, grailsClosure
}
def grails(String targetsArg = null, boolean useWrapperArg = false, Closure grailsClosure = null) {
GrailsContext grailsContext = new GrailsContext(
useWrapper: useWrapperArg
)
AbstractContextHelper.executeInContext(grailsClosure, grailsContext)
def nodeBuilder = new NodeBuilder()
def grailsNode = nodeBuilder.'com.g2one.hudson.grails.GrailsBuilder' {
targets targetsArg ?: grailsContext.targetsString
name grailsContext.name
grailsWorkDir grailsContext.grailsWorkDir
projectWorkDir grailsContext.projectWorkDir
projectBaseDir grailsContext.projectBaseDir
serverPort grailsContext.serverPort
'properties' grailsContext.propertiesString
forceUpgrade grailsContext.forceUpgrade.toString()
nonInteractive grailsContext.nonInteractive.toString()
useWrapper grailsContext.useWrapper.toString()
}
stepNodes << grailsNode
}
/**
jryan-odin-test
*ivy-locked.xml
target/
true
true
jryan-odin-test
*ivy-locked.xml
lastBuild
lastStableBuild
43
BUILD_SELECTOR
*/
def copyArtifacts(String jobName, String includeGlob, Closure copyArtifactClosure) {
return copyArtifacts(jobName, includeGlob, '', copyArtifactClosure)
}
def copyArtifacts(String jobName, String includeGlob, String targetPath, Closure copyArtifactClosure) {
return copyArtifacts(jobName, includeGlob, targetPath, false, copyArtifactClosure)
}
def copyArtifacts(String jobName, String includeGlob, String targetPath = '', boolean flattenFiles, Closure copyArtifactClosure) {
return copyArtifacts(jobName, includeGlob, targetPath, flattenFiles, false, copyArtifactClosure)
}
def copyArtifacts(String jobName, String includeGlob, String targetPath = '', boolean flattenFiles, boolean optionalAllowed, Closure copyArtifactClosure) {
CopyArtifactContext copyArtifactContext = new CopyArtifactContext()
AbstractContextHelper.executeInContext(copyArtifactClosure, copyArtifactContext)
if (!copyArtifactContext.selectedSelector) {
throw new IllegalArgumentException("A selector has to be select in the closure argument")
}
def nodeBuilder = NodeBuilder.newInstance()
def copyArtifactNode = nodeBuilder.'hudson.plugins.copyartifact.CopyArtifact' {
projectName jobName // Older name for field
project jobName // Newer name for field
filter includeGlob
target targetPath?:''
selector('class':"hudson.plugins.copyartifact.${copyArtifactContext.selectedSelector}Selector") {
if (copyArtifactContext.selectedSelector == 'TriggeredBuild' && copyArtifactContext.fallback) {
fallbackToLastSuccessful 'true'
}
if (copyArtifactContext.selectedSelector == 'PermalinkBuild') {
id copyArtifactContext.permalinkName
}
if (copyArtifactContext.selectedSelector == 'SpecificBuild') {
buildNumber Integer.toString(copyArtifactContext.buildNumber)
}
if (copyArtifactContext.selectedSelector == 'ParameterizedBuild') {
parameterName copyArtifactContext.parameterName
}
}
if (flattenFiles) {
flatten 'true'
}
if (optionalAllowed) {
optional 'true'
}
}
stepNodes << copyArtifactNode
}
/**
* phaseName will have to be provided in the closure
* @param phaseContext
* @return
*/
def phase(Closure phaseContext) {
phase(null, 'SUCCESSFUL', phaseContext)
}
def phase(String phaseName, Closure phaseContext = null) {
phase(phaseName, 'SUCCESSFUL', phaseContext)
}
def phase(String name, String continuationConditionArg, Closure phaseClosure) {
PhaseContext phaseContext = new PhaseContext(name, continuationConditionArg)
AbstractContextHelper.executeInContext(phaseClosure, phaseContext)
Preconditions.checkArgument phaseContext.phaseName as Boolean, "A phase needs a name"
def validConditions = ['SUCCESSFUL', 'UNSTABLE', 'COMPLETED']
Preconditions.checkArgument(validConditions.contains(phaseContext.continuationCondition), "Continuation Condition need to be one of these values: ${validConditions.join(',')}" )
def nodeBuilder = NodeBuilder.newInstance()
def multiJobPhaseNode = nodeBuilder.'com.tikal.jenkins.plugins.multijob.MultiJobBuilder' {
phaseName phaseContext.phaseName
continuationCondition phaseContext.continuationCondition
phaseJobs {
phaseContext.jobsInPhase.each { jobInPhase ->
'com.tikal.jenkins.plugins.multijob.PhaseJobsConfig' {
jobName jobInPhase.jobName
currParams jobInPhase.currentJobParameters?'true':'false'
exposedSCM jobInPhase.exposedScm?'true':'false'
if (jobInPhase.hasConfig()) {
configs(jobInPhase.configAsNode().children())
} else {
configs('class': 'java.util.Collections$EmptyList')
}
}
}
}
}
stepNodes << multiJobPhaseNode
}
/**
*
* project-A,project-B
* false
*
*/
def prerequisite(String projectList = '', boolean warningOnlyBool = false) {
def nodeBuilder = new NodeBuilder()
def preReqNode = nodeBuilder.'dk.hlyh.ciplugins.prereqbuildstep.PrereqBuilder' {
// Important that there are no spaces for comma delimited values, plugin doesn't handle by trimming, so we will
projectList = projectList.tokenize(',').collect{ it.trim() }.join(',')
projects(projectList)
warningOnly(warningOnlyBool)
}
stepNodes << preReqNode
}
/**
// Current build parameters
// Parameters from properties file
some.properties
// Pass-through Git commit that was built
false
// Predefined properties
prop1=value1
prop2=value2
// Restrict matrix execution to a subset
label=="${TARGET}"
// Subversion revision
one-project,another-project
ALWAYS
false
UNSTABLE
1
YELLOW
FAILURE
2
RED
FAILURE
2
RED
false
*/
def downstreamParameterized(Closure downstreamClosure) {
DownstreamContext downstreamContext = new DownstreamContext()
AbstractContextHelper.executeInContext(downstreamClosure, downstreamContext)
def stepNode = downstreamContext.createDownstreamNode(true)
stepNodes << stepNode
}
/**
false
*/
def conditionalSteps(Closure conditonalStepsClosure) {
ConditionalStepsContext conditionalStepsContext = new ConditionalStepsContext()
AbstractContextHelper.executeInContext(conditonalStepsClosure, conditionalStepsContext)
if (conditionalStepsContext.stepNodes.size() > 1) {
stepNodes << conditionalStepsContext.createMultiStepNode()
} else {
stepNodes << conditionalStepsContext.createSingleStepNode()
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy