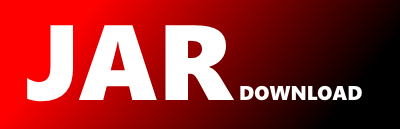
com.alibaba.fastjson.serializer.EasyjCollectionCodec Maven / Gradle / Ivy
/*
* Copyright 2021-2022 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.alibaba.fastjson.serializer;
import java.io.IOException;
import java.lang.reflect.Type;
import java.util.Collection;
import java.util.HashSet;
import java.util.TreeSet;
import com.alibaba.fastjson.JSONArray;
import com.alibaba.fastjson.JSONException;
import com.alibaba.fastjson.parser.DefaultJSONParser;
import com.alibaba.fastjson.parser.JSONToken;
import com.alibaba.fastjson.parser.deserializer.ObjectDeserializer;
import com.alibaba.fastjson.util.TypeUtils;
/**
* @author wenshao[[email protected]]
*/
public class EasyjCollectionCodec implements ObjectSerializer, ObjectDeserializer {
public static final EasyjCollectionCodec INSTANCE = new EasyjCollectionCodec();
@Override
public void write(JSONSerializer serializer, Object object, Object fieldName, Type fieldType, int features) throws IOException {
SerializeWriter out = serializer.out;
if (object == null) {
out.writeNull(SerializerFeature.WriteNullListAsEmpty);
return;
}
Type elementType = null;
if (out.isEnabled(SerializerFeature.WriteClassName)
|| SerializerFeature.isEnabled(features, SerializerFeature.WriteClassName)) {
elementType = TypeUtils.getCollectionItemType(fieldType);
}
Collection> collection = (Collection>)object;
SerialContext context = serializer.context;
serializer.setContext(context, object, fieldName, 0);
if (out.isEnabled(SerializerFeature.WriteClassName)) {
if (HashSet.class.isAssignableFrom(collection.getClass())) {
out.append("Set");
} else if (TreeSet.class == collection.getClass()) {
out.append("TreeSet");
}
}
try {
int i = 0;
out.append('[');
for (Object item : collection) {
if (i++ != 0) {
out.append(',');
}
if (item == null) {
out.writeNull();
continue;
}
Class> clazz = item.getClass();
if (clazz == Integer.class) {
out.writeInt(((Integer)item).intValue());
continue;
}
if (clazz == Long.class) {
ObjectSerializer writer = serializer.config.getObjectWriter(clazz);
try {
writer.write(serializer, item, null, null, 0);
} catch (IOException e) {
throw new JSONException(e.getMessage(), e);
}
continue;
}
ObjectSerializer itemSerializer = serializer.getObjectWriter(clazz);
if (SerializerFeature.isEnabled(features, SerializerFeature.WriteClassName)
&& itemSerializer instanceof JavaBeanSerializer) {
JavaBeanSerializer javaBeanSerializer = (JavaBeanSerializer)itemSerializer;
javaBeanSerializer.writeNoneASM(serializer, item, i - 1, elementType, features);
} else {
itemSerializer.write(serializer, item, i - 1, elementType, features);
}
}
out.append(']');
} finally {
serializer.context = context;
}
}
@SuppressWarnings({"unchecked", "rawtypes"})
@Override
public T deserialze(DefaultJSONParser parser, Type type, Object fieldName) {
if (parser.lexer.token() == JSONToken.NULL) {
parser.lexer.nextToken(JSONToken.COMMA);
return null;
}
if (type == JSONArray.class) {
JSONArray array = new JSONArray();
parser.parseArray(array);
return (T)array;
}
Collection list = TypeUtils.createCollection(type);
Type itemType = TypeUtils.getCollectionItemType(type);
parser.parseArray(itemType, list, fieldName);
return (T)list;
}
@Override
public int getFastMatchToken() {
return JSONToken.LBRACKET;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy