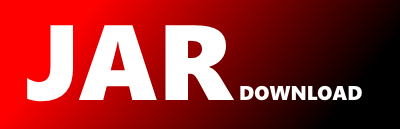
in.ashwanthkumar.slack.webhook.service.SlackService Maven / Gradle / Ivy
package in.ashwanthkumar.slack.webhook.service;
import com.google.api.client.http.GenericUrl;
import com.google.api.client.http.HttpRequestFactory;
import com.google.api.client.http.HttpTransport;
import com.google.api.client.http.UrlEncodedContent;
import com.google.api.client.http.javanet.NetHttpTransport;
import com.google.api.client.util.Maps;
import com.google.gson.Gson;
import in.ashwanthkumar.slack.webhook.SlackAttachment;
import in.ashwanthkumar.slack.webhook.SlackMessage;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import static in.ashwanthkumar.slack.webhook.util.StringUtils.isNotEmpty;
import static in.ashwanthkumar.slack.webhook.util.StringUtils.startsWith;
public class SlackService {
private final HttpTransport HTTP_TRANSPORT = new NetHttpTransport();
private final HttpRequestFactory requestFactory = HTTP_TRANSPORT.createRequestFactory();
public void push(String webHookUrl, SlackMessage text, String username, String imageOrIcon, String destination, List attachments) throws IOException {
Map payload = new HashMap();
if (isNotEmpty(username)) {
payload.put("username", username);
}
if (startsWith(imageOrIcon, "http")) {
payload.put("icon_url", imageOrIcon);
} else if (isNotEmpty(imageOrIcon)) {
payload.put("icon_emoji", imageOrIcon);
}
if (isNotEmpty(destination)) {
payload.put("channel", destination);
}
if (!attachments.isEmpty()) {
payload.put("attachments", attachments);
}
payload.put("text", text.toString());
execute(webHookUrl, payload);
}
public void push(String webHookUrl, SlackMessage text, String username, String imageOrIcon, String destination) throws IOException {
push(webHookUrl, text, username, imageOrIcon, destination, new ArrayList());
}
public void execute(String webHookUrl, Map payload) throws IOException {
String jsonEncodedMessage = new Gson().toJson(payload);
HashMap
© 2015 - 2024 Weber Informatics LLC | Privacy Policy