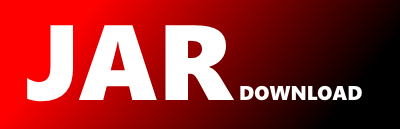
com.infusers.core.audit.AuditService Maven / Gradle / Ivy
package com.infusers.core.audit;
import java.net.URI;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.ResponseEntity;
import org.springframework.stereotype.Service;
import org.springframework.web.servlet.support.ServletUriComponentsBuilder;
@Service
public class AuditService {
final Logger log = LogManager.getLogger(AuditService.class);
@Autowired(required = true)
private AuditRepository auditRepository;
public ResponseEntity
© 2015 - 2025 Weber Informatics LLC | Privacy Policy