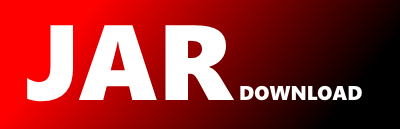
com.infusers.core.aws.s3.AwsS3Service Maven / Gradle / Ivy
package com.infusers.core.aws.s3;
import com.amazonaws.services.s3.AmazonS3;
import com.amazonaws.services.s3.model.PutObjectRequest;
import com.amazonaws.services.s3.model.S3Object;
import com.infusers.core.audit.AuditEventType;
import com.infusers.core.audit.AuditRecord;
import com.infusers.core.audit.AuditService;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.core.io.InputStreamResource;
import org.springframework.stereotype.Service;
import org.springframework.web.multipart.MultipartFile;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.Objects;
@Service
public class AwsS3Service {
@Autowired
private AmazonS3 amazonS3Client;
@Autowired(required = true)
private AuditService auditService;
final Logger log = LogManager.getLogger(AwsS3Service.class);
private static final String CLASS_NAME = "AwsS3Service";
@Value("${infusers.file.aws.s3.bucketname}")
private String bucketName;
public String getBucketName() {
return bucketName;
}
public boolean isAWSS3Configured() {
return bucketName!=null && bucketName.trim().length()!=0;
}
private boolean createBucket() {
if(!isAWSS3Configured()) {
return false;
}
if (amazonS3Client.doesBucketExistV2(bucketName)) {
return true;
//throw new RuntimeException("Bucket already exists");
}
return amazonS3Client.createBucket(bucketName)!=null;
}
public InputStreamResource downloadFile(String fileName) throws IOException {
if(!isAWSS3Configured()) {
log.error("AwsS3Service.downloadFile() File delete not possible, bucket name is not configured and/or aws is not accessable. bucket name = "+bucketName+" :: fileName = "+fileName);
auditService.createAuditRecord(new AuditRecord(fileName, AuditEventType.LEVEL_1, CLASS_NAME, "downloadFile()-> File delete not possible, bucket name is not configured and/or aws is not accessable. bucket name = "+bucketName+" :: fileName = "+fileName));
return null;
}
S3Object s3Object = amazonS3Client.getObject(bucketName, fileName);
return new InputStreamResource(Objects.requireNonNull(s3Object.getObjectContent()), "File");
}
public void deleteFile(String fileName) {
if(!isAWSS3Configured()) {
log.error("AwsS3Service.deleteFile() File delete not possible, bucket name is not configured and/or aws is not accessable. bucket name = "+bucketName);
auditService.createAuditRecord(new AuditRecord(fileName, AuditEventType.LEVEL_1, CLASS_NAME, "deleteFile()-> File delete not possible, bucket name is not configured and/or aws is not accessable. bucket name = "+bucketName));
return;
}
boolean success = createBucket();
if(success) {
amazonS3Client.deleteObject(bucketName, fileName);
}
else {
log.error("AwsS3Service.deleteFile() File delete not possible, bucket doesn't exits/not possible to create/aws is not accessable. bucket name = "+bucketName);
auditService.createAuditRecord(new AuditRecord(fileName, AuditEventType.LEVEL_1, CLASS_NAME, "deleteFile()-> File delete not possible, bucket doesn't exits/not possible to create/aws is not accessable. bucket name = "+bucketName));
}
}
public void uploadFile(MultipartFile multipartFile, String fileName2Save) throws IOException {
if(!isAWSS3Configured()) {
log.error("AwsS3Service.uploadFile() File upload not possible, bucket name is not configured and/or aws is not accessable. bucket name = "+bucketName+" :: fileName2Save = "+fileName2Save);
auditService.createAuditRecord(new AuditRecord(multipartFile.getName(), AuditEventType.LEVEL_1, CLASS_NAME, "uploadFile()-> File upload not possible, bucket name is not configured and/or aws is not accessable. bucket name = "+bucketName+":: fileName2Save = "+fileName2Save));
return;
}
boolean success = createBucket();
if(success) {
File file = convertMultiPartFileToFile(multipartFile);
PutObjectRequest putObjectRequest = new PutObjectRequest(bucketName, fileName2Save, file);
amazonS3Client.putObject(putObjectRequest);
success = file.delete();
}
else {
log.error("AwsS3Service.uploadFile() File delete not possible, bucket doesn't exits/not possible to create/aws is not accessable. bucket name = "+bucketName);
auditService.createAuditRecord(new AuditRecord(fileName2Save, AuditEventType.LEVEL_1, CLASS_NAME, "uploadFile()-> File delete not possible, bucket doesn't exits/not possible to create/aws is not accessable. bucket name = "+bucketName));
}
}
private File convertMultiPartFileToFile(MultipartFile multipartFile) throws IOException {
File file = new File(multipartFile.getOriginalFilename());
FileOutputStream outputStream = null;
try {
outputStream = new FileOutputStream(file);
outputStream.write(multipartFile.getBytes());
}
catch(Exception e) {
log.error("AwsS3Service.convertMultiPartFileToFile() Exception in converting multipart file to file = "+multipartFile.getOriginalFilename());
}
finally {
if(outputStream!=null) {
outputStream.close();
}
}
return file;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy