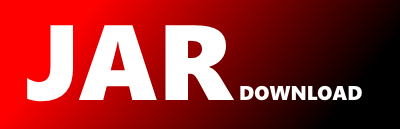
com.infusers.core.secrets.AppConfig Maven / Gradle / Ivy
package com.infusers.core.secrets;
import javax.sql.DataSource;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.boot.jdbc.DataSourceBuilder;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import com.google.gson.Gson;
import com.infusers.core.secrets.dto.DBSecrets;
@Configuration
public class AppConfig {
@Value("${spring.secretsmanager.db.details}")
String secretNameDB;
@Value("${spring.aws.secretsmanager.region}")
String region;
@Value("${spring.datasource.url}")
String url;
@Value("${spring.datasource.username}")
String userName;
@Value("${spring.datasource.password}")
String password;
private Logger log = LogManager.getLogger(AppConfig.class);
@Autowired
private SecretManager secretManager;
public String getSecretValue(String region, String secretName) {
return secretManager.getSecret(region, secretName);
}
@Bean
public DataSource dataSource() {
DBSecrets secrets = getDBSecrets();
if(secrets!=null) {
log.warn("AppConfig.dataSource() Aws/GCP secrets are available.");
return DataSourceBuilder.create()
.url("jdbc:" + secrets.getEngine() + "://" + secrets.getHost() + ":" + secrets.getPort() + "/"+secrets.getDbname())
.username(secrets.getUsername()).password(secrets.getPassword()).build();
}
else {
log.info("==============================================================================");
log.warn("AppConfig.dataSource() Secrets are NOT available, falling back to Inmemory DB.");
log.info("==============================================================================");
return DataSourceBuilder.create()
.url(url)
.username(userName).password(password).build();
}
}
private DBSecrets getDBSecrets() {
String secretsStr = secretManager.getSecret(region, secretNameDB);
Gson gson = new Gson();
return gson.fromJson(secretsStr, DBSecrets.class);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy