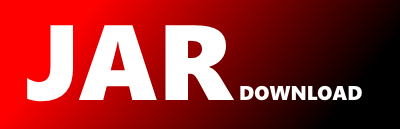
com.infusers.core.user.dto.APIUser Maven / Gradle / Ivy
package com.infusers.core.user.dto;
import java.io.Serializable;
import java.util.Date;
import jakarta.persistence.Column;
import jakarta.persistence.Entity;
import jakarta.persistence.GeneratedValue;
import jakarta.persistence.GenerationType;
import jakarta.persistence.Id;
import jakarta.persistence.PrePersist;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonProperty;
@Entity
public class APIUser implements Serializable {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private long id;
private Date createdAt;
@PrePersist
void createdAt() {
this.createdAt = new Date();
}
@Column(name = "username", unique=true)
private String username;
@JsonIgnore
@Column(name = "password")
private String password;
@Column(name = "firstname")
private String firstname;
@Column(name = "lastname")
private String lastname;
@Column(name = "admin")
private boolean admin = false;
@Column(name = "active")
private boolean active = true;
@Column(name = "provider")
private String provider;
@Column(name = "mobilenumber")
private Long mobilenumber;
@Column(name = "countrycode")
private String countrycode;
@Column(name = "verification_code", length = 64)
private String verificationCode;
public APIUser() {
super();
}
public APIUser(long id, String username, String password, String firstName, String lastName, boolean isAdmin, boolean isActive, String provider, String countrycode, Long mobilenumber, String verificationCode) {
super();
this.id = id;
this.username = username;
this.password = password;
this.firstname = firstName;
this.lastname = lastName;
this.admin = isAdmin;
this.active = isActive;
this.provider = provider;
this.countrycode = countrycode;
this.mobilenumber = mobilenumber;
this.verificationCode = verificationCode;
this.createdAt();
}
public String getCountrycode() {
return countrycode;
}
public void setCountrycode(String countrycode) {
this.countrycode = countrycode;
}
public Long getMobilenumber() {
return mobilenumber;
}
public void setMobilenumber(Long mobilenumber) {
this.mobilenumber = mobilenumber;
}
public Date getCreatedAt() {
return createdAt;
}
public String getProvider() {
return provider;
}
public void setProvider(String provider) {
this.provider = provider;
}
public boolean isAdmin() {
return admin;
}
public void setAdmin(boolean isAdmin) {
this.admin = isAdmin;
}
public boolean isActive() {
return active;
}
public void setActive(boolean isActive) {
this.active = isActive;
}
public String getFirstname() {
return firstname;
}
public void setFirstname(String firstName) {
this.firstname = firstName;
}
public String getLastname() {
return lastname;
}
public void setLastname(String lastName) {
this.lastname = lastName;
}
public long getId()
{
return id;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
@JsonIgnore
public String getPassword() {
return password;
}
@JsonProperty
public void setPassword(String password) {
this.password = password;
}
public void setId(long id) {
this.id = id;
}
public boolean isValid() {
return username!=null && username.length()!=0 && ((password!=null && password.length()!=0) || (this.provider!=null && this.provider.trim().length()!=0)) && firstname!=null && firstname.length()!=0 && lastname!=null && lastname.length()!=0;
}
public String getVerificationCode() {
return verificationCode;
}
public void setVerificationCode(String verificationCode) {
this.verificationCode = verificationCode;
}
@Override
public int hashCode() {
return username!=null ? username.hashCode() : -1;
}
@Override
public boolean equals(Object obj) {
// if both the object references are
// referring to the same object.
if(this == obj)
return true;
if(obj == null || obj.getClass()!= this.getClass())
return false;
// type casting of the argument.
APIUser user = (APIUser) obj;
// comparing the state of argument with
// the state of 'this' Object.
return (user.username.equals(this.username));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy