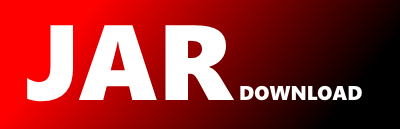
com.infusers.core.audit.AuditService Maven / Gradle / Ivy
package com.infusers.core.audit;
import java.net.URI;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.cache.annotation.CacheEvict;
import org.springframework.cache.annotation.CachePut;
import org.springframework.cache.annotation.Cacheable;
import org.springframework.cache.annotation.Caching;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.PageRequest;
import org.springframework.data.domain.Pageable;
import org.springframework.data.domain.Sort;
import org.springframework.data.jpa.domain.Specification;
import org.springframework.http.ResponseEntity;
import org.springframework.stereotype.Service;
import org.springframework.web.servlet.support.ServletUriComponentsBuilder;
import com.infusers.core.audit.response.AuditRecordsListDto;
import com.infusers.core.audit.search.AuditSpecification;
import com.infusers.core.user.RemoteUserService;
import com.infusers.core.user.dto.UserDetailsDto;
@Service
public class AuditService {
final Logger log = LogManager.getLogger(AuditService.class);
private static final String CLASS_NAME = "AuditService";
@Autowired(required = true)
private AuditRepository auditRepository;
@Autowired(required = true)
private RemoteUserService userService;
//@Cacheable(value = "auditList", key = "{ #pageNo, #pageSize, #sortBy, #order, #searchString, #userName }")
public AuditRecordsListDto getAllAuditRecords(Integer pageNo, Integer pageSize, String sortBy, String order, String searchString, String userName) {
log.debug(String.format(CLASS_NAME+".getAllAuditRecords() Fetching List of Audit Records on Pagination :: pageNo =%d :: pageSize = %d :: sortBy = '%s' :: order = '%s' :: searchString = %s :: userName = %s", pageNo, pageSize, sortBy, order, searchString, userName));
UserDetailsDto userDto = userService.getUser(userName);
Pageable paging;
if (order.equalsIgnoreCase("asc")) {
paging = PageRequest.of(pageNo, pageSize, Sort.by(sortBy).ascending());
}
else {
paging = PageRequest.of(pageNo, pageSize, Sort.by(sortBy).descending());
}
Page pagedResult = null;
boolean searchActive = searchString != null && !searchString.isEmpty();
if(searchActive) {
AuditSpecification spec = null;
if(userDto!=null && userDto.isAdmin()) {
spec = new AuditSpecification(null, searchString);;
}
else {
spec = new AuditSpecification(userDto!=null ? userDto.getUsername() : "", searchString);
}
pagedResult = auditRepository.findAll((Specification) spec.getSpec(), paging);
}
else {
if(userDto!=null && userDto.isAdmin()) {
pagedResult = auditRepository.findAll(paging);
}
else {
pagedResult = auditRepository.findByUserEmailId(userDto!=null ? userDto.getUsername() : "", paging);
}
}
AuditRecordsListDto auditList = new AuditRecordsListDto(userService);
if (pagedResult.hasContent()) {
auditList.copy(pagedResult.getContent(), pagedResult.getTotalElements());
}
return auditList;
}
//@Caching(evict = { @CacheEvict(value = "auditList", allEntries = true), }, put = {
//@CachePut(value="auditList", key="#auditRecord.getId()", condition="#id!=null")})
//@Caching(put = {
// @CachePut(value = "auditList", key = "#auditRecord.getId()", condition = "#auditRecord.getId() != null")
// })
//@CacheEvict(value = "auditList", allEntries = true)
private ResponseEntity
© 2015 - 2025 Weber Informatics LLC | Privacy Policy