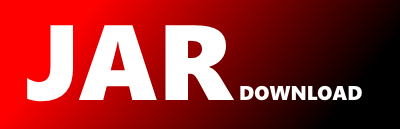
com.infusers.core.secrets.SecretsLoader Maven / Gradle / Ivy
package com.infusers.core.secrets;
import java.util.Properties;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import org.springframework.core.env.ConfigurableEnvironment;
import org.springframework.core.env.PropertiesPropertySource;
import org.springframework.stereotype.Component;
import org.springframework.boot.ApplicationRunner;
import org.springframework.context.annotation.Lazy;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.ApplicationArguments;
import com.google.gson.Gson;
import com.infusers.core.constants.InfuserConstants;
import com.infusers.core.logger.ILogger;
import com.infusers.core.secrets.dto.EmailSecrets;
import com.infusers.core.secrets.dto.OtherSecrets;
import com.infusers.core.secrets.dto.RabbitMQSecrets;
import com.infusers.core.util.InfusersUtility;
//@Component
//@Lazy(false) // Set @Lazy to false for eager initialization
public class SecretsLoader { //implements ApplicationRunner {
private ILogger log = new ILogger(SecretsLoader.class);
String emailSecretName = "infusers-email-configuration";
String otherSecretName = "infusers-others";
//@Value("${spring.aws.secretsmanager.region}")
//String region = "us-east-1";
public static final String PROPERTY_NAME_EMAIL_PASSWORD = "spring.mail.password";
public static final String PROPERTY_NAME_EMAIL_USER_NAME = "spring.mail.username";
public static final String PROPERTY_NAME_EMAIL_HOST = "spring.mail.host";
public static final String PROPERTY_NAME_EMAIL_PORT = "spring.mail.port";
private static final String RABBIT_MQ_SECRET_NAME = "infusers-prod-rabbitmq";
private static final String PROPERTY_NAME_RABBIT_MQ_HOST = "spring.rabbitmq.host";
private static final String PROPERTY_NAME_RABBIT_MQ_PORT = "spring.rabbitmq.port";
private static final String PROPERTY_NAME_RABBIT_MQ_USER_NAME = "spring.rabbitmq.username";
private static final String PROPERTY_NAME_RABBIT_MQ_PASSWORD = "spring.rabbitmq.password";
private SecretManager secretManager;
private ConfigurableEnvironment configEnvironment;
public SecretsLoader(SecretManager secretManager, ConfigurableEnvironment configEnvironment) {
this.secretManager = secretManager;
this.configEnvironment = configEnvironment;
this.loadSecrets();
}
/*@Override
public void run(ApplicationArguments args) throws Exception {
loadSecrets();
} */
private void loadSecrets() {
try {
log.warnWithSeparator("SecretsLoader.loadSecrets() isCloudEnvironment() = "+this.secretManager.isCloudEnvironment());
if(this.secretManager.isCloudEnvironment()) {
log.warnWithSeparator("SecretsLoader.loadSecrets() :: This is Cloud Environment/Profile, about to load secrets!!");
if(secretManager==null) {
log.errorWithSeparator("SecretsLoader.loadSecrets() :: Something is seriously wrong. STOP STOP STOP!! Secrets Manager is NULL..");
throw new RuntimeException("SecretsLoader.loadSecrets() :: Something is seriously wrong. STOP STOP STOP!! Secrets Manager is NULL..");
}
loadEmailConfiguration();
loadOtherConfiguration();
loadRabbitMQConfiguration();
}
else {
log.warnWithSeparator("SecretsLoader.loadSecrets() :: Email's NOT configured, Active Prfile is NOT a Cloud Enviornment/Profile!!");
}
}
catch(Exception e) {
log.errorWithSeparator("SecretsLoader.loadSecrets() :: Error loading secrets."+e.getMessage());
}
}
private void loadOtherConfiguration() {
String secretsStr = secretManager.getSecret(InfuserConstants.STATIC_TEXT_ACTIVE_AWS_REGION, otherSecretName);
Gson gson = new Gson();
OtherSecrets secrets = gson.fromJson(secretsStr, OtherSecrets.class);
if(secrets==null) {
log.errorWithSeparator("SecretsLoader.loadOtherConfiguration() :: OtherSecrets are NOT available.");
throw new RuntimeException("Others secrets are NULL, needs attention!!");
}
log.warn("SecretsLoader.loadOtherConfiguration() :: OtherSecrets are available.");
double expiryHours = Double.parseDouble(secrets.getJwtTokenExpiryHours());
//if(expiryHours<=0) {
// expiryHours = 2;
//}
OtherSecrets.getInstance().setJwttokensecret(secrets.getJwttokensecret());
OtherSecrets.getInstance().setJwtTokenExpiryHoursNumber(expiryHours);
InfusersUtility.getInstance().setJWTTokenDynamicEnabled(true);
}
private void loadEmailConfiguration() {
String secretsStr = secretManager.getSecret(InfuserConstants.STATIC_TEXT_ACTIVE_AWS_REGION, emailSecretName);
Gson gson = new Gson();
EmailSecrets secrets = gson.fromJson(secretsStr, EmailSecrets.class);
if(secrets==null) {
log.errorWithSeparator("SecretsLoader.loadEmailConfiguration() :: EmailSecrets are NOT available. Emails won't work!!");
return;
}
log.warn("SecretsLoader.loadEmailConfiguration() :: EmailSecrets are available. Host = "+secrets.getHost()+" :: Port = "+secrets.getPort());
Properties props = new Properties();
props.put(PROPERTY_NAME_EMAIL_PASSWORD, secrets.getPassword());
props.put(PROPERTY_NAME_EMAIL_HOST, secrets.getHost());
props.put(PROPERTY_NAME_EMAIL_PORT, secrets.getPort());
props.put(PROPERTY_NAME_EMAIL_USER_NAME, secrets.getUsername());
configEnvironment.getPropertySources().addFirst(new PropertiesPropertySource("aws.secret.manager", props));
InfusersUtility.getInstance().setEmailConfigrued(true);
}
private void loadRabbitMQConfiguration() {
try {
String secretsStr = secretManager.getSecret(InfuserConstants.STATIC_TEXT_ACTIVE_AWS_REGION, RABBIT_MQ_SECRET_NAME);
Gson gson = new Gson();
RabbitMQSecrets secrets = gson.fromJson(secretsStr, RabbitMQSecrets.class);
if(secrets!=null) {
log.warnWithSeparator("SecretsLoader.loadRabbitMQConfiguration() :: RabbitMQSecrets are available");
String host = secrets.getHost();
String port = secrets.getPort();
Properties props = new Properties();
props.put(PROPERTY_NAME_RABBIT_MQ_PASSWORD, secrets.getPassword());
props.put(PROPERTY_NAME_RABBIT_MQ_USER_NAME, secrets.getUsername());
if(host!=null && port!=null && host.trim().length()>0 && port.trim().length()>0) {
log.warnWithSeparator("SecretsLoader.loadRabbitMQConfiguration() :: RabbitMQSecrets Host/Port details also available. host = "+host+" :: port = "+port);
props.put(PROPERTY_NAME_RABBIT_MQ_HOST, secrets.getHost());
props.put(PROPERTY_NAME_RABBIT_MQ_PORT, secrets.getPort());
}
configEnvironment.getPropertySources().addFirst(new PropertiesPropertySource("aws.secret.manager", props));
}
else {
log.errorWithSeparator("SecretsLoader.loadRabbitMQConfiguration() :: RabbitMQSecrets are NULL, Its okay if you don't have RabbitMQ installed, otherwise, needs attention!!");
}
}
catch(Exception e) {
log.error("SecretsLoader.loadRabbitMQConfiguration() :: Exception while loading RabbitMQ secretsL. "+e.getMessage());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy