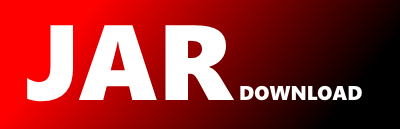
com.infusers.core.version.deployment.CloudProviderService Maven / Gradle / Ivy
package com.infusers.core.version.deployment;
import java.util.HashMap;
import java.util.Map;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.core.env.Environment;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.stereotype.Service;
//import com.infusers.core.audit.AuditService;
@Service
public class CloudProviderService {
private final Environment environment;
private static final String CLASS_NAME = "CloudProviderService";
//@Autowired(required = true)
//private AuditService auditService;
final Logger log = LogManager.getLogger(CloudProviderService.class);
@Autowired
public CloudProviderService(Environment environment) {
this.environment = environment;
}
public ResponseEntity
© 2015 - 2025 Weber Informatics LLC | Privacy Policy