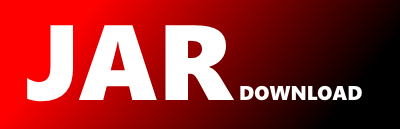
com.infusers.core.audit.AuditService Maven / Gradle / Ivy
package com.infusers.core.audit;
import java.io.UnsupportedEncodingException;
import java.net.URI;
import java.net.URLDecoder;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.Pageable;
import org.springframework.data.jpa.domain.Specification;
import org.springframework.http.ResponseEntity;
import org.springframework.stereotype.Service;
import org.springframework.web.servlet.support.ServletUriComponentsBuilder;
import com.infusers.core.logger.ILogger;
import com.infusers.core.reports.IReportService;
import com.infusers.core.util.InfusersUtility;
import jakarta.servlet.http.HttpServletRequest;
@Service
public class AuditService implements IReportService {
private final Logger log = LogManager.getLogger(AuditService.class);
private static final String CLASS_NAME = "AuditService";
@Autowired(required = true)
private AuditRepository auditRepository;
@Autowired
private InfusersUtility infusersUtility;
@Override
public Page
© 2015 - 2025 Weber Informatics LLC | Privacy Policy