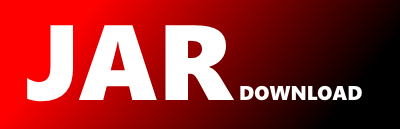
com.infusers.core.logger.ILogger Maven / Gradle / Ivy
package com.infusers.core.logger;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
public class ILogger {
private final Logger logger;
public enum LogTypes {
INFO,
DEBUG,
WARN,
WARN_SEP,
ERROR,
ERROR_SEP
}
public ILogger(Class> clazz) {
this.logger = LogManager.getLogger(clazz);
}
public void log(LogTypes type, String message) {
switch (type) {
case INFO:
this.info(message);
break;
case DEBUG:
this.debug(message);
break;
case WARN:
this.warn(message);
break;
case WARN_SEP:
this.warnWithSeparator(message);
break;
case ERROR:
this.error(message);
break;
case ERROR_SEP:
this.errorWithSeparator(message);
break;
default:
this.errorWithSeparator("Unknown log type, update ILogger's log(LogTypes) to include this type = "+type+" Message to log :: "+message);
break;
}
}
public void infoSeparator() {
logger.info("******************************Info****************************************");
}
public void debugSeparator() {
logger.debug("*****************************Debug*****************************************");
}
public void warnSeparator() {
logger.warn("******************************Warn****************************************");
}
public void errorSeparator() {
logger.error("*****************************Error*****************************************");
}
public void info(String message) {
logger.info(message);
}
public void debug(String message) {
logger.debug(message);
}
public void warn(String message) {
logger.warn(message);
}
public void warnWithSeparator(String message) {
this.warnSeparator();
this.warn(message);
this.warnSeparator();
}
public void warnWithSeparator(String message, boolean b) {
this.warnSeparator();
this.warn(message, b);
this.warnSeparator();
}
public void warn(String message, boolean b) {
logger.warn(message, b);
}
public void error(String message) {
logger.error(message);
}
public void errorWithSeparator(String message) {
this.errorSeparator();
this.error(message);
this.errorSeparator();
}
public void debugWithSeparator(String message) {
this.debugSeparator();
this.debug(message);
this.debugSeparator();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy