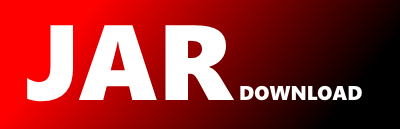
com.infusers.core.user.util.UserUtility Maven / Gradle / Ivy
package com.infusers.core.user.util;
import java.util.Date;
import java.util.Map;
import javax.crypto.SecretKey;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.infusers.core.constants.Constants;
import com.infusers.core.exception.security.InfusersTokenExpiredException;
import com.infusers.core.logger.ILogger;
import com.infusers.core.secrets.SecretsService;
import com.infusers.core.secrets.dto.OtherSecrets;
import com.infusers.core.user.dto.UserCredDto;
import com.infusers.core.util.InfusersUtility;
import io.jsonwebtoken.Claims;
import io.jsonwebtoken.ExpiredJwtException;
import io.jsonwebtoken.Jws;
import io.jsonwebtoken.Jwts;
import io.jsonwebtoken.io.Decoders;
import io.jsonwebtoken.security.Keys;
@Component
public class UserUtility {
@Autowired
private SecretsService secrets;
private ILogger log = new ILogger(UserUtility.class);
private static final String CLASS_NAME = "UserUtility";
@Autowired
private InfusersUtility infusersUtility;
public UserUtility(SecretsService secrets) {
this.secrets = secrets;
}
public static boolean isValid(UserCredDto userCred) {
String username = userCred.getUsername();
String password = userCred.getPassword();
return username!=null && username.length()!=0 && password!=null && password.length()!=0;
}
public String getUser(final String token) {
String jwtToken = token;
if(jwtToken!=null) {
jwtToken = jwtToken.trim().replace(Constants.STATIC_TEXT_BEARER,"").trim();
if(isValidJWTFormat(jwtToken)) {
try {
Jws claimsJws =
Jwts.parser()
.verifyWith(getKey())
.build().parseSignedClaims(jwtToken);
return claimsJws.getPayload().getSubject();
}
catch(ExpiredJwtException ex) {
log.error(CLASS_NAME+".getUser() :: JWT Token expired. "+ex.getMessage()+" :: token = "+token+" :: jwtToken = "+jwtToken);
throw new InfusersTokenExpiredException("Token expired"+" :: "+ex.getMessage());
}
catch (Exception e) {
log.error(CLASS_NAME+".getUser() :: exception. "+e.getMessage()+" :: token = "+token+" :: jwtToken = "+jwtToken);
}
}
}
return null;
}
private boolean isValidJWTFormat(final String token) {
if(token!=null) {
try {
// Split the token into its components
String[] tokenParts = token.split("\\.");
// Ensure there are three parts (header, payload, signature)
if (tokenParts.length != 3) {
return false;
}
// Decode each part (not verifying the signature)
// String decodedHeader = new String(Decoders.BASE64URL.decode(tokenParts[0]));
// String decodedPayload = new String(Decoders.BASE64URL.decode(tokenParts[1]));
// Parse JSON objects
// You can perform additional checks on the decoded header and payload if needed
return true; // Token format is valid
}
catch (Exception e) {
log.error(CLASS_NAME+".isJWTFormatValid() :: exception. "+e.getMessage()+" :: token = "+token);
}
}
return false;
}
public String getToken(final String userName) {
return Jwts.builder()
.issuedAt(new Date())
.expiration(new Date(System.currentTimeMillis() + (int)(getAutoLogoutTimeMinutes()*60*1000)))
.claim("name", userName)
.claim("email", userName)
.subject(userName)
.issuer("infusers.in")
.signWith(getKey())
.compact();
}
public String getToken() {
return getToken(infusersUtility.getLoggedInUserName());
}
private SecretKey getKey() {
OtherSecrets otherSec = secrets.getOtherSecrets();
if(otherSec == null || !otherSec.isValid()) {
String error = CLASS_NAME+".getKey() Other secrets are NULL/invalid!!, "+otherSec;
log.errorWithSeparator(error);
throw new RuntimeException(error);
}
return Keys.hmacShaKeyFor(Decoders.BASE64URL.decode(otherSec.getJwttokensecret()));
}
public Long getAutoLogoutTimeMinutes() {
OtherSecrets otherSec = secrets.getOtherSecrets();
if(otherSec == null || !otherSec.isValid()) {
String error = CLASS_NAME+".getAutoLogoutTimeMinutes() Other secrets are NULL/invalid!!, "+otherSec;
log.errorWithSeparator(error);
throw new RuntimeException(error);
}
return Long.valueOf((long)otherSec.getJwtTokenExpiryHoursNumber()*60);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy