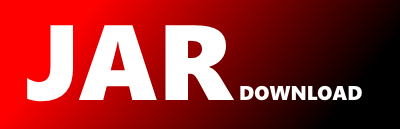
com.infusers.core.util.InfusersUtility Maven / Gradle / Ivy
package com.infusers.core.util;
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.attribute.FileAttribute;
import java.nio.file.attribute.PosixFilePermission;
import java.nio.file.attribute.PosixFilePermissions;
import java.util.Set;
import org.apache.commons.lang3.SystemUtils;
import org.springframework.core.env.Environment;
import org.springframework.security.authentication.AnonymousAuthenticationToken;
import org.springframework.security.core.Authentication;
import org.springframework.security.core.context.SecurityContextHolder;
import org.springframework.security.core.userdetails.UserDetails;
import org.springframework.stereotype.Component;
import org.springframework.web.multipart.MultipartFile;
import com.infusers.core.constants.Constants;
import com.infusers.core.logger.ILogger;
@Component
public class InfusersUtility {
private ILogger log = new ILogger(InfusersUtility.class);
private static final String CLASS_NAME = "InfusersUtility";
public static final String ANONYMOUS_USER = "anonymousUser";
public String getActiveProfile(Environment environment) {
String[] activeProfiles = environment!=null ? environment.getActiveProfiles() : null;
if (activeProfiles!=null && activeProfiles.length > 0) {
return activeProfiles[0];
}
else {
log.error(CLASS_NAME+".getActiveProfile() No active profiles!, " + activeProfiles);
}
return null;
}
public boolean isActiveProfileDevelopment(Environment environment) {
return environment!=null && isActiveProfileDevelopment(getActiveProfile(environment));
}
public boolean isActiveProfileDevelopment(String activeProfile) {
return activeProfile!=null && activeProfile.contains(Constants.STATIC_TEXT_ACTIVE_PROFILE_DEV);
}
public Long parseString2Long(final String stringVal) {
return stringVal==null? 0 : Long.parseLong(stringVal.trim());
}
public String getLoggedInUserName() {
Authentication auth = SecurityContextHolder.getContext().getAuthentication();
if (auth == null || !auth.isAuthenticated()) {
return null; // Or return "anonymousUser" if preferred
}
Object principal = auth.getPrincipal();
if (principal instanceof UserDetails) {
return ((UserDetails) principal).getUsername();
} else if (principal instanceof String) {
return (String) principal;
} else {
log.errorWithSeparator(CLASS_NAME + ".getLoggedInUserName() Unexpected principal type: " + principal.getClass().getName());
return null;
}
}
public String getLoggedInUserNameForAudit() {
Authentication auth = SecurityContextHolder.getContext().getAuthentication();
if (auth == null) {
log.debug(CLASS_NAME + ".getLoggedInUserNameForAudit() No user logged in (Authentication is null).");
return ANONYMOUS_USER;
}
if (auth instanceof AnonymousAuthenticationToken) {
log.debug(CLASS_NAME + ".getLoggedInUserNameForAudit() User is anonymous: " + auth);
return ANONYMOUS_USER;
}
if (auth.isAuthenticated()) {
Object principal = auth.getPrincipal();
if (principal instanceof UserDetails) {
return ((UserDetails) principal).getUsername();
} else if (principal instanceof String) {
return (String) principal;
} else {
log.errorWithSeparator(CLASS_NAME + ".getLoggedInUserNameForAudit() Unexpected principal type: " + principal.getClass().getName());
return ANONYMOUS_USER;
}
} else {
log.errorWithSeparator(CLASS_NAME + ".getLoggedInUserNameForAudit() User is not authenticated: " + auth);
return ANONYMOUS_USER;
}
}
public File convertMultiPartFileToFile(MultipartFile file) throws IOException {
java.io.File tempFile = null;
try {
String originalFilename = file.getOriginalFilename();
String timestamp = String.valueOf(System.currentTimeMillis());
String safeFilename = originalFilename.replaceAll("[^a-zA-Z0-9.-]", "_");
if(SystemUtils.IS_OS_UNIX) {
FileAttribute> attr = PosixFilePermissions.asFileAttribute(PosixFilePermissions.fromString("rwx------"));
tempFile = Files.createTempFile("eng-insights-pom_" + safeFilename + "_", "_" + timestamp + ".tmp", attr).toFile();
}
else {
tempFile = File.createTempFile("eng-insights-pom_" + safeFilename + "_", "_" + timestamp + ".tmp");
tempFile.setReadable(true, true);
tempFile.setWritable(true, true);
tempFile.setExecutable(true, true);
}
file.transferTo(tempFile);
}
catch(Exception e) {
log.error(CLASS_NAME+".convertMultiPartFileToFile() Exception in converting multipart file to file = "+file.getOriginalFilename());
throw e;
}
return tempFile;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy