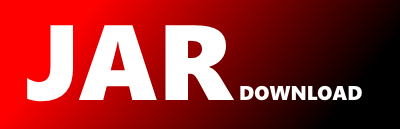
com.infusers.core.email.remote.RemoteEmailUtility Maven / Gradle / Ivy
The newest version!
package com.infusers.core.email.remote;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.ApplicationEventPublisher;
import org.springframework.context.event.EventListener;
import org.springframework.scheduling.annotation.Async;
import org.springframework.stereotype.Service;
import com.infusers.core.email.remote.eventdriven.EmailEvent;
import com.infusers.core.logger.ILogger;
import com.infusers.core.user.dto.IUserDto;
import com.infusers.core.user.event.UserLoggedInEvent;
@Service
public class RemoteEmailUtility {
private final ILogger log = new ILogger(RemoteEmailUtility.class);
private static final String CLASS_NAME = RemoteEmailUtility.class.getSimpleName();
@Value("${infusers.auth.verify.url}")
private String authVerifyEndPoint;
@Value("${infusers.email.endpoint}")
private String emailEndPoint;
@Value("${infusers.email.url}")
private String host;
@Value("${myapp.common.prefix.mapping}")
private String requestMapping;
@Autowired
private ApplicationEventPublisher eventPublisher;
public boolean sendEmailNewAccountCreated(IUserDto user) {
if(user==null) {
log.error(CLASS_NAME+".sendEmailNewAccountCreated()--> user is NULL." + user);
return false;
}
String subject = "[FYA] Warm welcome to Infusers!! Please verify your registration.";
String content =
"You have created a new Account!
"
+ "Please click the link below to verify your registration:
"
+ "VERIFY
";
String verifyURL = authVerifyEndPoint + "?code=" + user.getVerificationCode();
content = content.replace("[[URL]]", verifyURL);
return sendEmail(user.getUsername(), subject, content);
}
@EventListener
public void handleUserLoggedInEvent(UserLoggedInEvent event) {
try {
String subject = "[FYI] Dear Infuser, Welcome Back :)";
String message = "Thank you for your continued support in using Infuser's services, Have a great time ahead!!";
sendEmail(event.getUserName(), subject, message);
}
catch(Exception e) {
log.error(CLASS_NAME + ".handleUserLoggedInEvent() -> Error hadling user logged in event: "+ e+" :: User Name = "+event.getUserName());
}
}
@Async
public boolean sendEmail(String userName, String subject, String message) {
String methodName = "sendEmail";
log.debug(CLASS_NAME+"."+methodName+" userName : " + userName+" :: subject = "+subject);
EmailMessage eMessage = new EmailMessage(userName, subject, message, host + requestMapping + emailEndPoint);
eventPublisher.publishEvent(new EmailEvent(this, eMessage));
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy