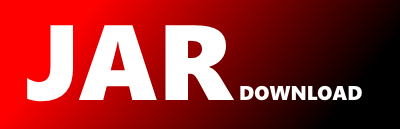
com.infusers.core.user.dto.UserAddressDto Maven / Gradle / Ivy
The newest version!
package com.infusers.core.user.dto;
import java.io.Serializable;
import org.springframework.data.redis.core.RedisHash;
import org.springframework.data.redis.serializer.JdkSerializationRedisSerializer;
import org.springframework.security.oauth2.core.oidc.AddressStandardClaim;
import lombok.Data;
@Data // This generates getters, setters, toString, equals, and hashCode
@RedisHash("UserAddressDto")
public class UserAddressDto extends JdkSerializationRedisSerializer implements Serializable, IUserAddressDto {
private static final long serialVersionUID = 443911446941799444L;
private Long id;
private String userId;
private String type;
private String street;
private String city;
private String state;
private String pincode;
private String country;
private boolean isDefault;
public UserAddressDto() {
}
public UserAddressDto(IUserAddressDto userAddress) {
id = userAddress.getId();
userId = userAddress.getUserId();
update(userAddress);
}
public void update(AddressStandardClaim oidcAddress) {
if(oidcAddress!=null) {
this.street = oidcAddress.getStreetAddress();
this.city = oidcAddress.getLocality();
this.state = oidcAddress.getRegion();
this.pincode = oidcAddress.getPostalCode();
this.country = oidcAddress.getCountry();
this.isDefault = true;
}
}
public void update(IUserAddressDto address) {
if(address!=null) {
this.type = address.getType();
this.street = address.getStreet();
this.city = address.getCity();
this.state = address.getState();
this.pincode = address.getPincode();
this.country = address.getCountry();
this.isDefault = address.isDefault();
}
}
public String getUserId() {
return userId;
}
public void setUserId(String userId) {
this.userId = userId;
}
public Long getId() {
return id;
}
public String getType() {
return type;
}
public boolean isDefault() {
return isDefault;
}
public void setDefault(boolean isDefault) {
this.isDefault = isDefault;
}
public String getStreet() {
return street;
}
public String getCity() {
return city;
}
public String getState() {
return state;
}
public String getPincode() {
return pincode;
}
public String getCountry() {
return country;
}
@Override
public String toString() {
return "UserAddressDto [id=" + id + ", userId=" + userId + ", type=" + type + ", street=" + street + ", city="
+ city + ", state=" + state + ", pincode=" + pincode + ", country=" + country + ", isDefault="
+ isDefault + "]";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy