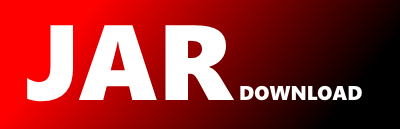
com.infusers.core.secrets.SecretsService Maven / Gradle / Ivy
package com.infusers.core.secrets;
import java.util.Properties;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.core.env.ConfigurableEnvironment;
import org.springframework.core.env.PropertiesPropertySource;
import org.springframework.stereotype.Service;
import com.google.gson.Gson;
import com.infusers.core.logger.ILogger;
import com.infusers.core.secrets.dto.DBSecrets;
import com.infusers.core.secrets.dto.EmailSecrets;
import com.infusers.core.secrets.dto.ISecrets;
import com.infusers.core.secrets.dto.OtherSecrets;
import com.infusers.core.secrets.dto.RabbitMQSecrets;
@Service
public class SecretsService {
private ILogger log = new ILogger(SecretsService.class);
private static final String CLASS_NAME = "SecretsService";
public static final String PROPERTY_NAME_EMAIL_PASSWORD = "spring.mail.password";
public static final String PROPERTY_NAME_EMAIL_USER_NAME = "spring.mail.username";
public static final String PROPERTY_NAME_EMAIL_HOST = "spring.mail.host";
public static final String PROPERTY_NAME_EMAIL_PORT = "spring.mail.port";
private static final String PROPERTY_NAME_RABBIT_MQ_HOST = "spring.rabbitmq.host";
private static final String PROPERTY_NAME_RABBIT_MQ_PORT = "spring.rabbitmq.port";
private static final String PROPERTY_NAME_RABBIT_MQ_USER_NAME = "spring.rabbitmq.username";
private static final String PROPERTY_NAME_RABBIT_MQ_PASSWORD = "spring.rabbitmq.password";
//@Value("${spring.aws.secretsmanager.region}")
private String region = "us-east-1";
//@Value("${spring.secretsmanager.db.details}")
private static final String secretNameDB = "infusers-prod-db-postgre";
private static final String RABBIT_MQ_SECRET_NAME = "infusers-prod-rabbitmq";
private static final String emailSecretName = "infusers-email-configuration";
private static final String otherSecretName = "infusers-others";
private Gson gson = new Gson();
private OtherSecrets otherSecrets;
private EmailSecrets emailSecrets;
private RabbitMQSecrets rabbitSecrets;
private DBSecrets dbSecrets;
@Autowired
private ConfigurableEnvironment configEnvironment;
@Autowired
private SecretManager secretManager;
public SecretsService(SecretManager secretManager, ConfigurableEnvironment configEnvironment, DBSecrets dbSecrets, RabbitMQSecrets rabbitSecrets, EmailSecrets emailSecrets, OtherSecrets otherSecrets) {
this.secretManager = secretManager;
this.configEnvironment = configEnvironment;
this.dbSecrets = dbSecrets;
this.rabbitSecrets = rabbitSecrets;
this.emailSecrets = emailSecrets;
this.otherSecrets = otherSecrets;
init();
}
private void init() {
loadSecrets();
updateApplicationProperties();
}
private void loadSecrets() {
if(secretManager==null) {
String error = CLASS_NAME+".loadSecrets() :: Secrets Manager is NULL.. Something is seriously wrong. STOP STOP STOP!!";
log.errorWithSeparator(error);
throw new RuntimeException(error);
}
if(secretManager.isCloudEnvironment()) {
log.warnWithSeparator(CLASS_NAME+".loadSecrets() :: This is Cloud Environment/Profile, about to load secrets!!");
dbSecrets = (DBSecrets) loadSecret(secretNameDB, DBSecrets.class);
rabbitSecrets = (RabbitMQSecrets) loadSecret(RABBIT_MQ_SECRET_NAME, RabbitMQSecrets.class);
otherSecrets = (OtherSecrets) loadSecret(otherSecretName, OtherSecrets.class);
emailSecrets = (EmailSecrets) loadSecret(emailSecretName, EmailSecrets.class);
}
else {
log.warnWithSeparator(CLASS_NAME+".loadSecrets() :: This is NOT a Cloud Environment/Profile, secrets won't be available!!");
}
if(this.rabbitSecrets==null || !this.rabbitSecrets.isValid()) {
log.errorWithSeparator(CLASS_NAME+".loadSecrets()--> rabbitSecrets are NULL/invalid. "+rabbitSecrets);
}
if(this.emailSecrets==null || !this.emailSecrets.isValid()) {
log.errorWithSeparator(CLASS_NAME+".loadSecrets()--> emailSecrets are NULL/invalid. "+emailSecrets);
}
{
boolean dbSecretsValid = this.dbSecrets!=null;
if(dbSecretsValid) {
if(secretManager.isCloudEnvironment()) {
dbSecretsValid = this.dbSecrets.isValidCloud();
}
else {
dbSecretsValid = this.dbSecrets.isValidNonCloud();
}
}
if(!dbSecretsValid) {
String error = CLASS_NAME+".loadSecrets()--> dbSecrets are NULL/invalid. "+dbSecrets;
log.errorWithSeparator(error);
throw new RuntimeException(error);
}
}
if(this.otherSecrets==null || !this.otherSecrets.isValid()) {
String error = CLASS_NAME+".loadSecrets()--> otherSecrets are NULL/invalid. "+otherSecrets;
log.errorWithSeparator(error);
throw new RuntimeException(error);
}
}
private ISecrets loadSecret(String secretName, Class className) {
String msg = CLASS_NAME+".loadSecret() --> It's cloud environment, secretManager = "+secretManager+" :: secretName = "+secretName+" :: className = "+className;
log.debugWithSeparator(msg);
if(secretName==null || secretName.trim().length()<1) {
String error = CLASS_NAME+".loadSecret() --> It's cloud environment, but Secret Name is empty/null. Something is NOT right here, needs attention!! secretName = "+secretName;
log.errorWithSeparator(error);
return null;
}
String secretsStr = secretManager.getSecret(region, secretName);
return (ISecrets) gson.fromJson(secretsStr, className);
}
private void updateApplicationProperties() {
loadEmailConfiguration();
loadRabbitMQConfiguration();
}
private void loadEmailConfiguration() {
if(emailSecrets!=null && emailSecrets.isValid()) {
log.warnWithSeparator(CLASS_NAME+".loadEmailConfiguration() :: EmailSecrets are available.");
Properties props = new Properties();
props.put(PROPERTY_NAME_EMAIL_PASSWORD, emailSecrets.getPassword());
props.put(PROPERTY_NAME_EMAIL_HOST, emailSecrets.getHost());
props.put(PROPERTY_NAME_EMAIL_PORT, emailSecrets.getPort());
props.put(PROPERTY_NAME_EMAIL_USER_NAME, emailSecrets.getUsername());
configEnvironment.getPropertySources().addFirst(new PropertiesPropertySource("aws.secret.manager", props));
}
else {
log.errorWithSeparator(CLASS_NAME+".loadEmailConfiguration() :: EmailSecrets are NULL/Invalid, Email functionality won't work!! "+emailSecrets);
}
}
private void loadRabbitMQConfiguration() {
try {
if(this.rabbitSecrets!=null && this.rabbitSecrets.isValid()) {
log.warnWithSeparator(CLASS_NAME+".loadRabbitMQConfiguration() :: RabbitMQSecrets are available.");
Properties props = new Properties();
props.put(PROPERTY_NAME_RABBIT_MQ_PASSWORD, rabbitSecrets.getPassword());
props.put(PROPERTY_NAME_RABBIT_MQ_USER_NAME, rabbitSecrets.getUsername());
props.put(PROPERTY_NAME_RABBIT_MQ_HOST, rabbitSecrets.getHost());
props.put(PROPERTY_NAME_RABBIT_MQ_PORT, rabbitSecrets.getPort());
configEnvironment.getPropertySources().addFirst(new PropertiesPropertySource("aws.secret.manager", props));
}
else {
log.errorWithSeparator(CLASS_NAME+".loadRabbitMQConfiguration() :: RabbitMQSecrets are NULL/Not Valid, Its okay if you don't have RabbitMQ installed, otherwise, needs attention!! "+this.rabbitSecrets);
}
}
catch(Exception e) {
log.error(CLASS_NAME+".loadRabbitMQConfiguration() :: Exception while loading RabbitMQ secretsL. "+e.getMessage());
}
}
public String getRegion() {
return region;
}
public OtherSecrets getOtherSecrets() {
return otherSecrets;
}
public EmailSecrets getEmailSecrets() {
return emailSecrets;
}
public RabbitMQSecrets getRabbitSecrets() {
return rabbitSecrets;
}
public DBSecrets getDBSecrets() {
return dbSecrets;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy