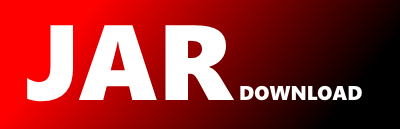
com.infusers.core.secrets.gcp.GCPSecretManager Maven / Gradle / Ivy
package com.infusers.core.secrets.gcp;
import java.io.IOException;
import java.util.concurrent.TimeUnit;
import javax.annotation.PreDestroy;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Bean;
import com.google.cloud.secretmanager.v1.AccessSecretVersionResponse;
import com.google.cloud.secretmanager.v1.SecretManagerServiceClient;
import com.google.cloud.secretmanager.v1.SecretVersionName;
import com.infusers.core.secrets.SecretManager;
public class GCPSecretManager implements SecretManager {
private Logger log = LogManager.getLogger(GCPSecretManager.class);
@Value("${infusers.secretsmanager.gcp.projectdetails}")
private String gcpProjectDetails;
@Bean
public SecretManagerServiceClient secretManagerServiceClient() throws IOException {
try {
return SecretManagerServiceClient.create();
}
catch (IOException e) {
log.error("GCPSecretManager.secretManagerServiceClient()-> Failed to create SecretManagerServiceClient, looks like this is NOT GCP environment?" + e.getMessage());
throw e; // Rethrow the exception
}
}
@Override
public boolean isCloudEnvironment() {
return true;
}
@Override
public String getSecret(String region, String secretName) {
//String secretFullName = this.gcpProjectDetails+secretName;
try {
SecretManagerServiceClient secretManagerServiceClient = secretManagerServiceClient();
if (secretManagerServiceClient ==null) {
log.error("GCPSecretManager.getSecret()-> SecretManagerServiceClient is NULL, looks like this is NOT GCP environment?");
return null;
}
SecretVersionName secretVersionName = SecretVersionName.of("540509425443", secretName, "latest");
AccessSecretVersionResponse response = secretManagerServiceClient.accessSecretVersion(secretVersionName);
log.warn("GCPSecretManager.getSecret() :: FOUND!! secretName = "+secretName+" :: secretVersionName = "+secretVersionName);
return response.getPayload().getData().toStringUtf8();
}
catch (Exception e) {
log.error("GCPSecretManager.getSecret()" + e.getMessage()+" :: secretName = "+secretName);
//e.printStackTrace();
}
return null;
}
@PreDestroy
public void shutdown() {
SecretManagerServiceClient client;
try {
client = secretManagerServiceClient();
client.shutdown();
try {
client.awaitTermination(5, TimeUnit.SECONDS);
}
catch (InterruptedException e) {
log.error("GCPSecretManager.shutdown() InterruptedException : " + e.getMessage());
}
}
catch (IOException e) {
log.error("GCPSecretManager.shutdown() IOException : " + e.getMessage());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy