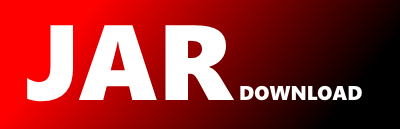
com.infusers.core.util.InfusersUtility Maven / Gradle / Ivy
package com.infusers.core.util;
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.attribute.FileAttribute;
import java.nio.file.attribute.PosixFilePermission;
import java.nio.file.attribute.PosixFilePermissions;
import java.util.Date;
import java.util.Set;
import javax.crypto.SecretKey;
import org.apache.commons.lang3.SystemUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.core.env.Environment;
import org.springframework.security.core.Authentication;
import org.springframework.security.core.context.SecurityContextHolder;
import org.springframework.stereotype.Component;
import org.springframework.web.multipart.MultipartFile;
import com.infusers.core.constants.Constants;
import com.infusers.core.logger.ILogger;
import com.infusers.core.secrets.SecretsService;
import com.infusers.core.secrets.dto.OtherSecrets;
import io.jsonwebtoken.Claims;
import io.jsonwebtoken.Jws;
import io.jsonwebtoken.Jwts;
import io.jsonwebtoken.io.Decoders;
import io.jsonwebtoken.security.Keys;
@Component
public class InfusersUtility {
@Autowired
private SecretsService secrets;
private ILogger log = new ILogger(InfusersUtility.class);
private static final String CLASS_NAME = "InfusersUtility";
private static final Long MINUTES_UNITL_AUTO_LOGOUT = 15L; // in mins
public InfusersUtility(SecretsService secrets) {
this.secrets = secrets;
}
public Long getMaxTimeout() {
return MINUTES_UNITL_AUTO_LOGOUT * 60 * 1000;
}
public String getActiveProfile(Environment environment) {
String[] activeProfiles = environment!=null ? environment.getActiveProfiles() : null;
if (activeProfiles!=null && activeProfiles.length > 0) {
return activeProfiles[0];
}
else {
log.error(CLASS_NAME+".getActiveProfile() No active profiles!, " + activeProfiles);
}
return null;
}
public boolean isActiveProfileDevelopment(Environment environment) {
return environment!=null && isActiveProfileDevelopment(getActiveProfile(environment));
}
public boolean isActiveProfileDevelopment(String activeProfile) {
return activeProfile!=null && activeProfile.contains(Constants.STATIC_TEXT_ACTIVE_PROFILE_DEV);
}
private SecretKey getKey() {
OtherSecrets otherSec = secrets.getOtherSecrets();
if(otherSec == null || !otherSec.isValid()) {
String error = CLASS_NAME+".getKey() Other secrets are NULL/invalid!!, "+otherSec;
log.errorWithSeparator(error);
throw new RuntimeException(error);
}
return Keys.hmacShaKeyFor(Decoders.BASE64URL.decode(otherSec.getJwttokensecret()));
}
public String getToken(final String email) {
OtherSecrets otherSec = secrets.getOtherSecrets();
if(otherSec == null || !otherSec.isValid()) {
String error = CLASS_NAME+".getToken() Other secrets are NULL/invalid!!, "+otherSec;
log.errorWithSeparator(error);
throw new RuntimeException(error);
}
return Jwts.builder()
.issuedAt(new Date())
.expiration(new Date(System.currentTimeMillis() + (int)(otherSec.getJwtTokenExpiryHoursNumber()*60*60*1000)))
.claim("name", email)
.claim("email", email)
.subject(email)
.issuer("infusers.in")
.signWith(getKey())
.compact();
}
public String getUser(final String token) {
String jwtToken = token;
if(jwtToken!=null) {
jwtToken = jwtToken.trim().replace(Constants.STATIC_TEXT_BEARER,"").trim();
if(isValidJWTFormat(jwtToken)) {
try {
Jws claimsJws =
Jwts.parser()
.verifyWith(getKey())
.build().parseSignedClaims(jwtToken);
return claimsJws.getPayload().getSubject();
}
catch (Exception e) {
e.printStackTrace();
log.error(CLASS_NAME+".getUser() :: exception. "+e.getMessage()+" :: token = "+token+" :: jwtToken = "+jwtToken);
}
}
}
return null;
}
private boolean isValidJWTFormat(final String token) {
if(token!=null) {
try {
// Split the token into its components
String[] tokenParts = token.split("\\.");
// Ensure there are three parts (header, payload, signature)
if (tokenParts.length != 3) {
return false;
}
// Decode each part (not verifying the signature)
String decodedHeader = new String(Decoders.BASE64URL.decode(tokenParts[0]));
String decodedPayload = new String(Decoders.BASE64URL.decode(tokenParts[1]));
// Parse JSON objects
// You can perform additional checks on the decoded header and payload if needed
return true; // Token format is valid
}
catch (Exception e) {
log.error(CLASS_NAME+".isJWTFormatValid() :: exception. "+e.getMessage()+" :: token = "+token);
}
}
return false;
}
public Long parseString2Long(final String stringVal) {
return stringVal==null? 0 : Long.parseLong(stringVal.trim());
}
public String getLoggedInUserName() {
Authentication auth = SecurityContextHolder.getContext().getAuthentication();
if (auth != null && auth.isAuthenticated()) {
// User is authenticated, you can access their information here
Object pricipal = auth.getPrincipal();
if(pricipal!=null) {
return (String)pricipal;
}
else {
log.error(CLASS_NAME+".getLoggedInUserName() Principal is NULL, " + pricipal);
return null;
}
}
else {
// User is not authenticated, handle the case accordingly
log.error(CLASS_NAME+".getLoggedInUserName() Authentication is NULL, " + auth);
return null;
}
}
public File convertMultiPartFileToFile(MultipartFile file) throws IOException {
java.io.File tempFile = null;
try {
String originalFilename = file.getOriginalFilename();
String timestamp = String.valueOf(System.currentTimeMillis());
String safeFilename = originalFilename.replaceAll("[^a-zA-Z0-9.-]", "_");
if(SystemUtils.IS_OS_UNIX) {
FileAttribute> attr = PosixFilePermissions.asFileAttribute(PosixFilePermissions.fromString("rwx------"));
tempFile = Files.createTempFile("eng-insights-pom_" + safeFilename + "_", "_" + timestamp + ".tmp", attr).toFile();
}
else {
tempFile = File.createTempFile("eng-insights-pom_" + safeFilename + "_", "_" + timestamp + ".tmp");
tempFile.setReadable(true, true);
tempFile.setWritable(true, true);
tempFile.setExecutable(true, true);
}
file.transferTo(tempFile);
}
catch(Exception e) {
log.error(CLASS_NAME+".convertMultiPartFileToFile() Exception in converting multipart file to file = "+file.getOriginalFilename());
throw e;
}
return tempFile;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy