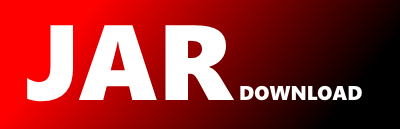
jlibs.nblr.editor.debug.Debugger Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jlibs-nblr Show documentation
Show all versions of jlibs-nblr Show documentation
Non-Blocking Language Recognition
/**
* Copyright 2015 Santhosh Kumar Tekuri
*
* The JLibs authors license this file to you under the Apache License,
* version 2.0 (the "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package jlibs.nblr.editor.debug;
import jlibs.core.annotation.processing.Printer;
import jlibs.core.io.FileUtil;
import jlibs.core.lang.ImpossibleException;
import jlibs.core.lang.StringUtil;
import jlibs.nblr.actions.BufferAction;
import jlibs.nblr.actions.ErrorAction;
import jlibs.nblr.actions.EventAction;
import jlibs.nblr.actions.PublishAction;
import jlibs.nblr.codegen.java.JavaCodeGenerator;
import jlibs.nblr.editor.RuleScene;
import jlibs.nblr.editor.Util;
import jlibs.nblr.rules.Edge;
import jlibs.nblr.rules.Node;
import jlibs.nblr.rules.Rule;
import jlibs.nbp.Chars;
import jlibs.nbp.NBHandler;
import javax.swing.*;
import javax.swing.event.CaretEvent;
import javax.swing.event.CaretListener;
import javax.swing.event.ListSelectionEvent;
import javax.swing.event.ListSelectionListener;
import javax.swing.text.BadLocationException;
import javax.swing.text.Highlighter;
import javax.tools.JavaCompiler;
import javax.tools.ToolProvider;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.io.*;
import java.net.URL;
import java.net.URLClassLoader;
import java.text.ParseException;
import java.util.ArrayList;
import java.util.Observable;
import java.util.Observer;
/**
* @author Santhosh Kumar T
*/
public class Debugger extends JPanel implements NBHandler, Observer{
private RuleScene scene;
public JTextArea input = new JTextArea();
private JList ruleStackList = new JList(new DefaultListModel());
public Debugger(RuleScene scene){
super(new BorderLayout(5, 5));
this.scene = scene;
scene.ruleObservable.addObserver(this);
JToolBar toolbar = Util.toolbar(
runAction,
debugAction,
null,
stepAction,
runToCursorAction,
resumeAction,
suspendAction
);
add(toolbar, BorderLayout.NORTH);
input.setFont(Util.FIXED_WIDTH_FONT);
input.addCaretListener(new CaretListener(){
@Override
public void caretUpdate(CaretEvent ce){
updateActions();
}
});
add(new JScrollPane(input), BorderLayout.CENTER);
ruleStackList.setFont(Util.FIXED_WIDTH_FONT);
ruleStackList.getSelectionModel().addListSelectionListener(new ListSelectionListener() {
@Override
public void valueChanged(ListSelectionEvent lse){
Rule rule = (Rule)ruleStackList.getSelectedValue();
RuleScene scene = Debugger.this.scene;
if(rule!=null && scene.getRule()!=rule)
scene.setRule(scene.getSyntax(), rule);
}
});
add(new JScrollPane(ruleStackList), BorderLayout.EAST);
message.setFont(Util.FIXED_WIDTH_FONT);
add(message, BorderLayout.SOUTH);
updateActions();
}
private String compile(File file){
JavaCompiler compiler = ToolProvider.getSystemJavaCompiler();
ArrayList args = new ArrayList();
args.add("-d");
args.add(file.getParentFile().getAbsolutePath());
args.add("-s");
args.add(file.getParentFile().getAbsolutePath());
args.add(file.getAbsolutePath());
ByteArrayOutputStream err = new ByteArrayOutputStream();
if(compiler.run(null, null, err, args.toArray(new String[args.size()]))==0)
return null;
return err.toString();
}
private DebuggableNBParser parser;
private int inputIndex;
private char[] inputText;
private int inputPosition;
private void start(){
try{
showMessage("");
clearGuardedBlock();
String parserName = "UntitledParser";
File file = new File("temp/"+parserName+".java").getAbsoluteFile();
FileUtil.mkdirs(file.getParentFile());
JavaCodeGenerator codeGenerator = new JavaCodeGenerator(scene.getSyntax());
codeGenerator.properties.put(JavaCodeGenerator.PARSER_CLASS_NAME, parserName);
codeGenerator.setDebuggable();
Printer printer = new Printer(new PrintWriter(new FileWriter(file)));
codeGenerator.generateParser(printer);
printer.close();
String error = compile(file);
if(error!=null){
JOptionPane.showMessageDialog(this, error);
return;
}
currentRule = scene.getRule();
URLClassLoader classLoader = new URLClassLoader(new URL[]{FileUtil.toURL(file.getParentFile())});
Class clazz = classLoader.loadClass(parserName);
parser = (DebuggableNBParser)clazz.getConstructor(getClass(), int.class).newInstance(this, scene.getRule().id);
inputText = input.getText(0, input.getDocument().getLength()).toCharArray();
showMessage("Executing...");
}catch(Exception ex){
ex.printStackTrace();
JOptionPane.showMessageDialog(this, ex.getMessage());
}
}
private void step(){
try{
if(inputIndexinputIndex)
throw new ImpossibleException("consumed="+consumed+" inputIndex="+inputIndex);
if(consumed>0)
input.getHighlighter().addHighlight(0, consumed, consumedHighlightPainter);
if(inputIndex!=consumed)
input.getHighlighter().addHighlight(consumed, inputIndex, lookAheadHighlightPainter);
input.repaint();
}
private void clearGuardedBlock(){
input.getHighlighter().removeAllHighlights();
input.repaint();
}
/*-------------------------------------------------[ Actions ]---------------------------------------------------*/
private void updateActions(){
if(scene.getSyntax()!=null)
ruleStackList.setPrototypeCellValue(scene.getSyntax().ruleProtypeWidth());
JScrollPane scroll = (JScrollPane)ruleStackList.getParent().getParent();
scroll.setVisible(parser!=null);
DefaultListModel model = (DefaultListModel)ruleStackList.getModel();
model.clear();
if(parser!=null){
Rule rules[] = scene.getSyntax().rules.values().toArray(new Rule[scene.getSyntax().rules.values().size()]);
for(int i=0; i=0; i--){
if(model.getElementAt(i)==rule){
ruleIndex = i;
break;
}
}
if(ruleIndex==-1)
ruleStackList.clearSelection();
else{
ruleStackList.setSelectedIndex(ruleIndex);
ArrayList states = new ArrayList();
for(int i=1; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy