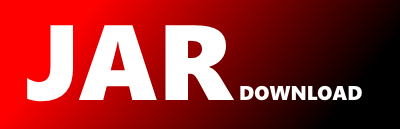
in.s8.rsa.controller.DecryptController Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of RSA-Encryption-Decryption Show documentation
Show all versions of RSA-Encryption-Decryption Show documentation
Project to RSA-encrypt-decrypt
The newest version!
package in.s8.rsa.controller;
import in.s8.rsa.Service.DecryptionService;
import in.s8.rsa.constant.S8Constant;
import in.s8.rsa.impl.DecryptImpl;
import in.s8.rsa.implService.DecryptService;
import org.springframework.stereotype.Component;
import javax.crypto.BadPaddingException;
import javax.crypto.IllegalBlockSizeException;
import javax.crypto.NoSuchPaddingException;
import javax.xml.bind.DatatypeConverter;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.ObjectInputStream;
import java.security.InvalidKeyException;
import java.security.PrivateKey;
/**
* Utility to decrypt the Data.
* Created by Sourabh_Sethi on 4/26/2016.
*
* @Author - Sourabh Sethi
*/
@Component("decryptionService")
public class DecryptController implements DecryptionService {
DecryptService decryptService = new DecryptImpl();
public String decryption(String encryptedTxt) {
ObjectInputStream inputStream = null;
String plainText = null;
try {
inputStream = new ObjectInputStream(new FileInputStream(S8Constant.PRIVATE_KEY_FILE));
final PrivateKey privateKey = (PrivateKey) inputStream.readObject();
String cipherText = encryptedTxt;
plainText = decryptService.decrypt(DatatypeConverter.parseHexBinary(cipherText), privateKey);
} catch (FileNotFoundException e) {
System.out.println("Please check the public key and Private Key Path");
System.out.println("Path Should be ./private.key");
} catch (Exception e) {
e.printStackTrace();
}
return plainText;
}
@Deprecated
public String decryptionSetup(String encryptedTxt, String privateKeyLocation) {
ObjectInputStream inputStream = null;
String plainText = null;
try {
inputStream = new ObjectInputStream(new FileInputStream(privateKeyLocation));
final PrivateKey privateKey = (PrivateKey) inputStream.readObject();
String cipherText = encryptedTxt;
plainText = decryptService.decrypt(DatatypeConverter.parseHexBinary(cipherText), privateKey);
} catch (FileNotFoundException e) {
System.out.println("Please check the public key and Private Key Path");
System.out.println("please provide the private key path");
} catch (Exception e) {
e.printStackTrace();
}
return plainText;
}
/**
* @param encryptedString
* @param privateKey
* @return decrypted String.
* @desc Decryption Service - used to Decrypted the string
*/
public String decryption(String encryptedString, PrivateKey privateKey) throws NoSuchPaddingException, BadPaddingException {
String plainText = null;
try {
plainText = decryptService.decrypt(DatatypeConverter.parseHexBinary(encryptedString), privateKey);
} catch (NoSuchPaddingException e) {
System.out.println("No such Padding !! Warning Please check the It may be Malicious Attempt to decrypt");
System.out.println("or please provide valid private key");
} catch (BadPaddingException e) {
System.out.println("Incorrect Padding !! Warning Please check the It may be Malicious Attempt to decrypt");
System.out.println("or please provide valid private key");
} catch (InvalidKeyException e) {
System.out.println("or please provide valid private key");
} catch (IllegalBlockSizeException e) {
System.out.println("Please check the block Size of Encrypted String and provided private Key");
} catch (Exception e) {
e.printStackTrace();
}
return plainText;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy