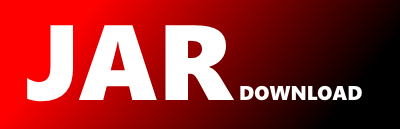
in.s8.rsa.impl.DecryptImpl Maven / Gradle / Ivy
package in.s8.rsa.impl;
import in.s8.rsa.constant.S8Constant;
import in.s8.rsa.implService.DecryptService;
import org.springframework.stereotype.Component;
import javax.crypto.BadPaddingException;
import javax.crypto.Cipher;
import javax.crypto.IllegalBlockSizeException;
import javax.crypto.NoSuchPaddingException;
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
import java.security.PrivateKey;
/**
* Decryption Implementation
* Created by Sourabh_Sethi on 5/17/2016.
*/
@Component("decryptImpl")
public class DecryptImpl implements DecryptService {
/**
* decrypt the data Using Private Key.
*
* @param text
* @param key
* @return
* @throws NoSuchPaddingException
* @throws NoSuchAlgorithmException
* @throws InvalidKeyException
* @throws BadPaddingException
* @throws IllegalBlockSizeException
*/
public String decrypt(byte[] text, PrivateKey key) throws NoSuchPaddingException, NoSuchAlgorithmException,
InvalidKeyException, BadPaddingException, IllegalBlockSizeException {
byte[] dectyptedText = null;
// get an RSA cipher object and print the provider
final Cipher cipher = Cipher.getInstance(S8Constant.ALGORITHM);
// decrypt the text using the private key
cipher.init(Cipher.DECRYPT_MODE, key);
dectyptedText = cipher.doFinal(text);
return new String(dectyptedText);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy