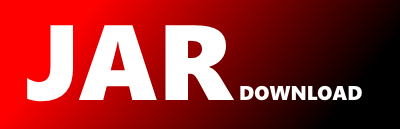
info.archinnov.achilles.persistence.AsyncBatch Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of achilles-core Show documentation
Show all versions of achilles-core Show documentation
CQL implementation for Achilles using Datastax Java driver
/*
* Copyright (C) 2012-2014 DuyHai DOAN
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package info.archinnov.achilles.persistence;
import com.datastax.driver.core.BatchStatement;
import com.google.common.util.concurrent.FutureCallback;
import info.archinnov.achilles.async.AchillesFuture;
import info.archinnov.achilles.internal.async.AsyncUtils;
import info.archinnov.achilles.internal.context.ConfigurationContext;
import info.archinnov.achilles.internal.context.DaoContext;
import info.archinnov.achilles.internal.context.PersistenceContextFactory;
import info.archinnov.achilles.internal.metadata.holder.EntityMeta;
import info.archinnov.achilles.type.Empty;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.Map;
import java.util.concurrent.ExecutorService;
/**
*
* Create an asynchronous Batch session to perform
*
* -
* insert()
*
* -
* update()
*
* -
* remove()
*
* -
* removeById()
*
*
*
* This AsyncBatch is a state-full object that stacks up all operations. They will be flushed upon call to
* asyncEndBatch()
*
*
*
*
* There are 2 types of batch: ordered and unordered. Ordered batches will automatically add
* increasing generated timestamp for each statement so that their ordering is guaranteed.
*
*
*
* AsyncBatch asyncBatch = asyncManager.createBatch();
*
* asyncBatch.insert(new User(10L, "John","LENNNON")); // nothing happens here
*
* Future emptyFuture = asyncBatch.asyncEndBatch(); // send the INSERT statement to Cassandra
*
*
*
* @see Batch Mode
*
*/
public class AsyncBatch extends CommonBatch {
private static final Logger log = LoggerFactory.getLogger(AsyncBatch.class);
private AsyncUtils asyncUtils = AsyncUtils.Singleton.INSTANCE.get();
AsyncBatch(Map, EntityMeta> entityMetaMap, PersistenceContextFactory contextFactory,
DaoContext daoContext, ConfigurationContext configContext, BatchStatement.Type batchType, boolean orderedBatch) {
super(entityMetaMap, contextFactory, daoContext, configContext, batchType, orderedBatch);
}
/**
* End an existing batch and flush all the pending statements asynchronously.
*
* Do nothing if there is no pending statement
*
* @return Future an empty future
*
*/
public AchillesFuture asyncEndBatch() {
log.debug("Flushing batch asynchronously");
try {
return flushContext.flushBatch();
} finally {
flushContext = flushContext.duplicateWithNoData(defaultConsistencyLevel);
}
}
/**
* End an existing batch and flush all the pending statements.
*
* Do nothing if there is no pending statement
*
*/
public AchillesFuture asyncEndBatch(FutureCallback
© 2015 - 2025 Weber Informatics LLC | Privacy Policy