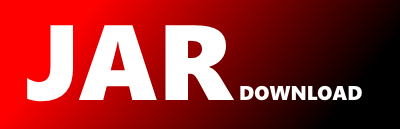
info.archinnov.achilles.persistence.CommonAsyncManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of achilles-core Show documentation
Show all versions of achilles-core Show documentation
CQL implementation for Achilles using Datastax Java driver
/*
* Copyright (C) 2012-2014 DuyHai DOAN
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package info.archinnov.achilles.persistence;
import info.archinnov.achilles.async.AchillesFuture;
import info.archinnov.achilles.internal.context.ConfigurationContext;
import info.archinnov.achilles.internal.context.DaoContext;
import info.archinnov.achilles.internal.context.PersistenceContextFactory;
import info.archinnov.achilles.internal.metadata.holder.EntityMeta;
import info.archinnov.achilles.type.Empty;
import info.archinnov.achilles.options.Options;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import javax.annotation.PreDestroy;
import java.util.Map;
import static info.archinnov.achilles.options.OptionsBuilder.noOptions;
public class CommonAsyncManager extends AbstractPersistenceManager {
private static final Logger log = LoggerFactory.getLogger(CommonAsyncManager.class);
protected CommonAsyncManager(Map, EntityMeta> entityMetaMap, PersistenceContextFactory contextFactory, DaoContext daoContext, ConfigurationContext configContext) {
super(entityMetaMap, contextFactory, daoContext, configContext);
}
/**
* Insert an entity asynchronously.
*
*
* // Insert
* Future managedEntityFuture = manager.insert(myEntity);
*
*
* @param entity
* Entity to be inserted
* @return future of managed entity
*
*/
public AchillesFuture insert(T entity) {
log.debug("Inserting asynchronously entity '{}'", entity);
return super.asyncInsert(entity, noOptions());
}
/**
* Insert an entity asynchronously with the given options.
*
*
* // Insert
* Future managedEntityFuture = asyncManager.insert(myEntity, OptionsBuilder.withTtl(3600));
*
*
* @param entity
* Entity to be inserted
* @param options
* options
* @return future of managed entity
*/
public AchillesFuture insert(final T entity, Options options) {
log.debug("Inserting asynchronously entity '{}' with options {} ", entity, options);
return super.asyncInsert(entity, options);
}
/**
* Update asynchronously a "managed" entity
*
*
* User managedUser = asyncManager.find(User.class,1L).get();
* user.setFirstname("DuyHai");
*
* Future userFuture = asyncManager.update(user);
*
*
* @param entity
* Managed entity to be updated
*
* @return future of managed entity
*/
public AchillesFuture update(T entity) {
log.debug("Updating asynchronously entity '{}'", proxifier.getRealObject(entity));
return super.asyncUpdate(entity, noOptions());
}
/**
* Update asynchronously a "managed" entity with options
*
*
* User managedUser = asyncManager.find(User.class,1L).get();
* user.setFirstname("DuyHai");
*
* Future userFuture = asyncManager.update(user, OptionsBuilder.withTtl(10));
*
*
* @param entity
* Managed entity to be updated
* @param options
* options
*
* @return future of managed entity
*/
public AchillesFuture update(T entity, Options options) {
log.debug("Updating asynchronously entity '{}' with options {} ", proxifier.getRealObject(entity), options);
return super.asyncUpdate(entity, options);
}
/**
* Insert a "transient" entity or update a "managed" entity asynchronously.
*
* Shorthand to insert() or update()
*
* @param entity
* Managed entity to be inserted/updated
*
* @return future of managed entity
*/
public AchillesFuture insertOrUpdate(T entity) {
log.debug("Inserting or updating asynchronously entity '{}'", proxifier.getRealObject(entity));
return this.asyncInsertOrUpdate(entity, noOptions());
}
/**
* Insert a "transient" entity or update a "managed" entity asynchronously with options.
*
* Shorthand to insert() or update()
*
* @param entity
* Managed entity to be inserted/updated
* @param options
* options
* @return future of managed entity
*/
public AchillesFuture insertOrUpdate(T entity, Options options) {
log.debug("Inserting or updating asynchronously entity '{}' with options {}", proxifier.getRealObject(entity), options);
return this.asyncInsertOrUpdate(entity,options);
}
/**
* Delete an entity asynchronously.
*
*
* // Simple deletion
* User managedUser = asyncManager.find(User.class,1L).get();
* Future userFuture = asyncManager.delete(managedUser);
*
*
* @param entity
* Entity to be deleted
*
* @return future of managed entity
*/
public AchillesFuture delete(T entity) {
log.debug("Removing asynchronously entity '{}'", proxifier.getRealObject(entity));
return super.asyncDelete(entity, noOptions());
}
/**
* Delete an entity asynchronously with the given options.
*
*
* // Deletion with option
* User managedUser = asyncManager.find(User.class,1L);
* Future userFuture = asyncManager.delete(managedUser, OptionsBuilder.withConsistency(QUORUM));
*
*
* @param entity
* Entity to be deleted
* @param options
* options for consistency level and timestamp
*
* @return future of managed entity
*/
public AchillesFuture delete(final T entity, Options options) {
log.debug("Removing asynchronously entity '{}' with options {}", proxifier.getRealObject(entity), options);
return super.asyncDelete(entity,options);
}
/**
* Delete asynchronously an entity by its id.
* The returned future will yield an {@code info.archinnov.achilles.type.Empty}.INSTANCE
*
*
* // Direct deletion without read-before-write
* Future emptyFuture = asyncManager.deleteById(User.class,1L);
*
*
* @param entityClass
* Entity class
*
* @param primaryKey
* Primary key
* @return future of {@code info.archinnov.achilles.type.Empty}.INSTANCE
*/
public AchillesFuture deleteById(Class> entityClass, Object primaryKey) {
log.debug("Deleting asynchronously entity of type '{}' by its id '{}'", entityClass, primaryKey);
return super.asyncDeleteById(entityClass, primaryKey, noOptions());
}
/**
* Delete asynchronously an entity by its id with the given options.
* The returned future will yield an {@code info.archinnov.achilles.type.Empty}.INSTANCE
*
*
* // Direct deletion without read-before-write
* Future emptyFuture = asyncManager.deleteById(User.class,1L,OptionsBuilder.withConsistency(QUORUM));
*
*
* @param entityClass
* Entity class
*
* @param primaryKey
* Primary key
*
* @return future of {@code info.archinnov.achilles.type.Empty}.INSTANCE
*/
public AchillesFuture deleteById(Class> entityClass, Object primaryKey, Options options) {
log.debug("Removing asynchronously entity of type '{}' by its id '{}'", entityClass, primaryKey);
return super.asyncDeleteById(entityClass, primaryKey, options);
}
/**
* Call shutdown on Achilles, especially shutdown the internal thread pool handling asynchronous tasks
*/
@PreDestroy
public void shutDown() {
configContext.getExecutorService().shutdown();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy