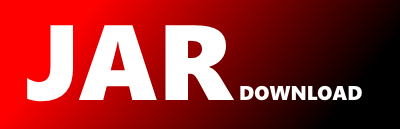
info.archinnov.achilles.query.cql.AbstractNativeQuery Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of achilles-core Show documentation
Show all versions of achilles-core Show documentation
CQL implementation for Achilles using Datastax Java driver
package info.archinnov.achilles.query.cql;
import com.datastax.driver.core.PagingState;
import com.datastax.driver.core.ResultSet;
import com.datastax.driver.core.Row;
import com.datastax.driver.core.Statement;
import com.google.common.base.Function;
import com.google.common.base.Optional;
import com.google.common.util.concurrent.FutureCallback;
import com.google.common.util.concurrent.ListenableFuture;
import info.archinnov.achilles.async.AchillesFuture;
import info.archinnov.achilles.internal.async.AsyncUtils;
import info.archinnov.achilles.internal.async.RowsWithExecutionInfo;
import info.archinnov.achilles.internal.context.ConfigurationContext;
import info.archinnov.achilles.internal.context.DaoContext;
import info.archinnov.achilles.internal.persistence.operations.NativeQueryMapper;
import info.archinnov.achilles.internal.persistence.operations.TypedMapIterator;
import info.archinnov.achilles.internal.statement.wrapper.NativeQueryLog;
import info.archinnov.achilles.internal.statement.wrapper.NativeStatementWrapper;
import info.archinnov.achilles.type.Empty;
import info.archinnov.achilles.options.Options;
import info.archinnov.achilles.type.TypedMap;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.Iterator;
import java.util.List;
import java.util.concurrent.ExecutorService;
import static info.archinnov.achilles.internal.async.AsyncUtils.RESULTSET_TO_ROWS;
import static info.archinnov.achilles.internal.async.AsyncUtils.RESULTSET_TO_ROWS_WITH_EXECUTION_INFO;
public abstract class AbstractNativeQuery> {
private static final Logger log = LoggerFactory.getLogger(AbstractNativeQuery.class);
protected NativeStatementWrapper nativeStatementWrapper;
protected DaoContext daoContext;
protected AsyncUtils asyncUtils = AsyncUtils.Singleton.INSTANCE.get();
protected NativeQueryMapper mapper = NativeQueryMapper.Singleton.INSTANCE.get();
protected Optional pagingStateO = Optional.absent();
protected ExecutorService executorService;
protected AbstractNativeQuery(DaoContext daoContext, ConfigurationContext configContext, Statement statement, Options options, Object... boundValues) {
this.daoContext = daoContext;
this.nativeStatementWrapper = new NativeStatementWrapper(NativeQueryLog.class, statement, boundValues, options.getLWTResultListener());
this.executorService = configContext.getExecutorService();
}
protected abstract T getThis();
protected ListenableFuture asyncGetInternal(FutureCallback
© 2015 - 2025 Weber Informatics LLC | Privacy Policy