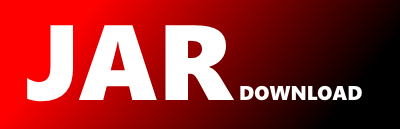
info.archinnov.achilles.query.cql.AsyncNativeQuery Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of achilles-core Show documentation
Show all versions of achilles-core Show documentation
CQL implementation for Achilles using Datastax Java driver
/*
* Copyright (C) 2012-2014 DuyHai DOAN
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package info.archinnov.achilles.query.cql;
import com.datastax.driver.core.Statement;
import com.google.common.base.Function;
import com.google.common.base.Optional;
import com.google.common.util.concurrent.FutureCallback;
import com.google.common.util.concurrent.ListenableFuture;
import info.archinnov.achilles.async.AchillesFuture;
import info.archinnov.achilles.internal.context.ConfigurationContext;
import info.archinnov.achilles.internal.context.DaoContext;
import info.archinnov.achilles.type.Empty;
import info.archinnov.achilles.options.Options;
import info.archinnov.achilles.type.TypedMap;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import javax.annotation.Nullable;
import java.util.Iterator;
import java.util.List;
/**
* Class to wrap CQL native query asynchronously
*
*
*
* Statement statement = new SimpleStatement("SELECT name,age_in_years FROM UserEntity WHERE id IN(?,?)");
* AchillesFuture> future = manager.nativeQuery(statement,10L,11L).get();
*
*
*
* @see Native query
*/
public class AsyncNativeQuery extends AbstractNativeQuery {
private static final Logger log = LoggerFactory.getLogger(AsyncNativeQuery.class);
public AsyncNativeQuery(DaoContext daoContext, ConfigurationContext configContext, Statement statement, Options options, Object... boundValues) {
super(daoContext, configContext, statement, options, boundValues);
}
@Override
protected AsyncNativeQuery getThis() {
return this;
}
/**
* Return found rows asynchronously. The list represents the number of returned rows The
* map contains the (column name, column value) of each row. The map is
* backed by a LinkedHashMap and thus preserves the columns order as they
* were declared in the native query
*
* @return AchillesFuture<List<TypedMap>>
*/
public AchillesFuture> get(FutureCallback
© 2015 - 2025 Weber Informatics LLC | Privacy Policy