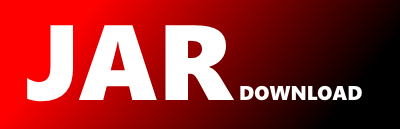
info.archinnov.achilles.query.slice.IteratePartitionRoot Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of achilles-core Show documentation
Show all versions of achilles-core Show documentation
CQL implementation for Achilles using Datastax Java driver
/*
* Copyright (C) 2012-2014 DuyHai DOAN
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package info.archinnov.achilles.query.slice;
import static info.archinnov.achilles.query.slice.BoundingMode.EXCLUSIVE_BOUNDS;
import static info.archinnov.achilles.query.slice.BoundingMode.INCLUSIVE_BOUNDS;
import static info.archinnov.achilles.query.slice.BoundingMode.INCLUSIVE_END_BOUND_ONLY;
import static info.archinnov.achilles.query.slice.BoundingMode.INCLUSIVE_START_BOUND_ONLY;
import static info.archinnov.achilles.query.slice.OrderingMode.ASCENDING;
import static info.archinnov.achilles.query.slice.OrderingMode.DESCENDING;
import java.util.Iterator;
import com.google.common.util.concurrent.FutureCallback;
import info.archinnov.achilles.internal.metadata.holder.EntityMeta;
import info.archinnov.achilles.internal.persistence.operations.SliceQueryExecutor;
import info.archinnov.achilles.type.ConsistencyLevel;
public abstract class IteratePartitionRoot> extends SliceQueryRootExtended {
protected IteratePartitionRoot(SliceQueryExecutor sliceQueryExecutor, Class entityClass, EntityMeta meta, SliceQueryProperties.SliceType sliceType) {
super(sliceQueryExecutor, entityClass, meta, sliceType);
}
/**
*
* Iterate over selected entities without filtering clustering keys. If no limit has been set, the default LIMIT 100 applies
*
*
*
* manager.sliceQuery(ArticleRating.class)
* .forIterate()
* .withPartitionComponents(articleId)
* .iterator();
*
*
*
* Generated CQL query:
*
*
* SELECT * FROM article_rating WHERE article_id=... ORDER BY rating ASC LIMIT 100
*
* @return slice DSL
*/
public Iterator iterator() {
return super.iteratorInternal();
}
/**
*
* Iterate over selected entities without filtering clustering keys using provided fetch size
*
*
*
* manager.sliceQuery(ArticleRating.class)
* .forIterate()
* .withPartitionComponents(articleId)
* .iterator(23);
*
*
*
* Generated CQL query:
*
*
* SELECT * FROM article_rating WHERE article_id=... ORDER BY rating ASC ASC LIMIT 100
*
*
*
* Note: if the fetch size is set, then you won't be able to use IN clause for partition components together ORDER BY.
* In this case, Achilles will remove automatically the ORDER BY clause
*
*
* @return slice DSL
*/
public Iterator iterator(int batchSize) {
super.properties.fetchSize(batchSize);
return super.iteratorInternal();
}
/**
*
* Iterate over entities with matching clustering keys
*
*
*
* manager.sliceQuery(ArticleRating.class)
* .forIterate()
* .withPartitionComponents(articleId)
* .iteratorWithMatching(2);
*
*
*
* Generated CQL query:
*
*
* SELECT * FROM article_rating WHERE article_id=... AND rating=2 ORDER BY rating ASC LIMIT 100
*
* @return slice DSL
*/
public Iterator iteratorWithMatching(Object... clusterings) {
super.withClusteringsInternal(clusterings);
return super.iteratorInternal();
}
/**
*
* Iterate over entities with matching clustering keys and fetch size
*
*
*
* manager.sliceQuery(ArticleRating.class)
* .forIterate()
* .withPartitionComponents(articleId)
* .iteratorWithMatchingAndBatchSize(10,2);
*
*
*
* Generated CQL query:
*
*
* SELECT * FROM article_rating WHERE article_id=... AND rating=2 ORDER BY rating ASC LIMIT 100
*
*
* Note: if the fetch size is set, then you won't be able to use IN clause for partition components together ORDER BY.
* In this case, Achilles will remove automatically the ORDER BY clause
*
*
* @return slice DSL
*/
public Iterator iteratorWithMatchingAndBatchSize(int batchSize, Object... clusterings) {
super.properties.fetchSize(batchSize);
super.withClusteringsInternal(clusterings);
return super.iteratorInternal();
}
/**
*
* Filter with lower bound clustering key(s)
*
*
*
* manager.sliceQuery(ArticleRating.class)
* .forIterate()
* .withPartitionComponents(articleId)
* .fromClusterings(2,now)
* .iterator();
*
*
*
* Generated CQL query:
*
*
* SELECT * FROM article_rating WHERE article_id=... AND (rating,date)>=(2,now) ORDER BY rating ASC LIMIT 100
*
* @return slice DSL
*/
public IterateFromClusterings fromClusterings(Object... clusteringKeys) {
super.fromClusteringsInternal(clusteringKeys);
return new IterateFromClusterings<>();
}
/**
*
* Filter with upper bound clustering key(s)
*
*
*
* manager.sliceQuery(ArticleRating.class)
* .forIterate()
* .withPartitionComponents(articleId)
* .toClusterings(3)
* .iterator();
*
*
*
* Generated CQL query:
*
*
* SELECT * FROM article_rating WHERE article_id=... AND rating<=3 ORDER BY rating ASC LIMIT 100
*
* @return slice DSL
*/
public IterateEnd toClusterings(Object... clusteringKeys) {
super.toClusteringsInternal(clusteringKeys);
return new IterateEnd<>();
}
/**
*
* Filter with matching clustering key(s)
*
*
*
* manager.sliceQuery(ArticleRating.class)
* .forIterate()
* .withPartitionComponents(articleId)
* .withClusterings(3)
* .iterator();
*
*
*
* Generated CQL query:
*
*
* SELECT * FROM article_rating WHERE article_id=... AND rating=3 ORDER BY rating ASC LIMIT 100
*
* @return slice DSL
*/
public IterateWithClusterings withClusterings(Object... clusteringKeys) {
super.withClusteringsInternal(clusteringKeys);
return new IterateWithClusterings<>();
}
public abstract class IterateClusteringsRootWithLimitation> {
/**
*
* Use ascending order for the first clustering key. This is the default ordering
*
*
*
* manager.sliceQuery(ArticleRating.class)
* .forSelect()
* .withPartitionComponents(articleId)
* .fromClusterings(2, now)
* .toClusterings(4)
* .orderByAscending()
* .get(20);
*
*
*
* Generated CQL query:
*
*
* SELECT * FROM article_rating WHERE article_id=... AND (rating,date)>=(2,now) AND (rating)<=4 ORDER BY rating ASC LIMIT 20
* @return Slice DSL
*/
public T orderByAscending() {
IteratePartitionRoot.super.properties.ordering(ASCENDING);
return getThis();
}
/**
*
* Use descending order for the first clustering key
*
*
*
* manager.sliceQuery(ArticleRating.class)
* .forSelect()
* .withPartitionComponents(articleId)
* .fromClusterings(2, now)
* .toClusterings(4)
* .orderByDescending()
* .get(20);
*
*
*
* Generated CQL query:
*
*
* SELECT * FROM article_rating WHERE article_id=... AND (rating,date)>=(2,now) AND (rating)<=4 ORDER BY rating DESC LIMIT 20
* @return Slice DSL
*/
public T orderByDescending() {
IteratePartitionRoot.super.properties.ordering(DESCENDING);
return getThis();
}
/**
*
* Set a limit to the query. A default limit of 100 is always set to avoid OutOfMemoryException
* You can remove it at your own risk using noLimit()
*
*
*
* manager.sliceQuery(ArticleRating.class)
* .forSelect()
* .withPartitionComponents(articleId)
* .fromClusterings(2, now)
* .toClusterings(4)
* .limit(5)
* .get();
*
*
*
* Generated CQL query:
*
*
* SELECT * FROM article_rating WHERE article_id=... AND (rating,date)>=(2,now) AND (rating)<=4 ORDER BY rating ASC LIMIT 5
* @return Slice DSL
*/
public T limit(int limit) {
IteratePartitionRoot.super.properties.limit(limit);
return getThis();
}
/**
*
* Provide a consistency level for SELECT statement
*
* @param consistencyLevel
* @return Slice DSL
*/
public T withConsistency(ConsistencyLevel consistencyLevel) {
IteratePartitionRoot.super.properties.readConsistency(consistencyLevel);
return getThis();
}
public T withAsyncListeners(FutureCallback
© 2015 - 2025 Weber Informatics LLC | Privacy Policy