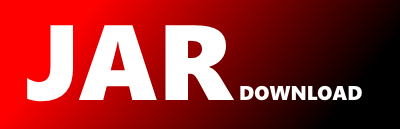
info.archinnov.achilles.configuration.ConfigurationParameters Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of achilles-core Show documentation
Show all versions of achilles-core Show documentation
CQL implementation for Achilles using Datastax Java driver
/*
* Copyright (C) 2012-2016 DuyHai DOAN
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package info.archinnov.achilles.configuration;
/**
* Enum listing all configuration parameters
*
*
* Entity Management
*
* -
* MANAGED_ENTITIES (OPTIONAL): list of entities to be managed by Achilles.
*
Example: my.project.entity,another.project.entity
*
*
*
*
* DDL
*
* -
* FORCE_SCHEMA_GENERATION (OPTIONAL): create missing column families for entities if they are not found. Default = 'false'
If set to false and no column family is found for any entity, Achilles will raise an AchillesInvalidColumnFamilyException
*
*
*
*
* JSON Serialization
*
* -
* JACKSON_MAPPER_FACTORY (OPTIONAL): an implementation of the info.archinnov.achilles.json.JacksonMapperFactory interface to build custom Jackson ObjectMapper based on entity class
*
* -
* JACKSON_MAPPER (OPTIONAL): default Jackson ObjectMapper to use for serializing entities
*
*
If both JACKSON_MAPPER_FACTORY and JACKSON_MAPPER parameters are provided, Achilles will ignore the JACKSON_MAPPER parameter and use JACKSON_MAPPER_FACTORY
If none is provided, Achilles will use a default Jackson ObjectMapper with the following configuration:
*
* - MapperFeature.SORT_PROPERTIES_ALPHABETICALLY = true
* - SerializationInclusion = JsonInclude.Include.NON_NULL
* - DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES = false
* - AnnotationIntrospector pair : primary = JacksonAnnotationIntrospector, secondary = JaxbAnnotationIntrospector
*
*
*
* Consistency Level
*
* - CONSISTENCY_LEVEL_READ_DEFAULT (OPTIONAL): default read consistency level for all entities
* - CONSISTENCY_LEVEL_WRITE_DEFAULT (OPTIONAL): default write consistency level for all entities
* - CONSISTENCY_LEVEL_SERIAL_DEFAULT (OPTIONAL): default serial consistency level for all entities
* - CONSISTENCY_LEVEL_READ_MAP (OPTIONAL): map(String,String) of read consistency levels for column families/tables
*
*
* Example:
"columnFamily1" -> "ONE"
* "columnFamily2" -> "QUORUM"
* ...
*
*
* -
* CONSISTENCY_LEVEL_WRITE_MAP (OPTIONAL): map(String,String) of write consistency levels for column families
* Example:
* "columnFamily1" -> "ALL"
* "columnFamily2" -> "EACH_QUORUM"
* ...
*
*
* -
* CONSISTENCY_LEVEL_SERIAL_MAP (OPTIONAL): map(String,String) of write consistency levels for column families
* Example:
* "columnFamily1" -> "SERIAL"
* "columnFamily2" -> "LOCAL_SERIAL"
* ...
*
*
*
*
*
* Events Interceptors
*
* - EVENT_INTERCEPTORS (OPTIONAL): list of events interceptors.
*
*
*
* Bean Validation
*
* - BEAN_VALIDATION_ENABLE (OPTIONAL): whether to enable Bean Validation for PRE_INSERT/PRE_UPDATE events.
* -
* POST_LOAD_BEAN_VALIDATION_ENABLE (OPTIONAL): whether to enable Bean Validation for POST_LOAD event.
* Please note that this flag is taken into account only if BEAN_VALIDATION_ENABLE is true
*
* - BEAN_VALIDATION_VALIDATOR (OPTIONAL): custom validator to be used.
If no validator is provided, Achilles will get the default validator provided by the default Validation provider.
* If Bean Validation is enabled at runtime but no default Validation provider can be found, an exception will be raised and the bootstrap is aborted
*
*
*
*
* Prepared Statements Cache
*
* - PREPARED_STATEMENTS_CACHE_SIZE (OPTIONAL): define the LRU cache size for prepared statements cache.
By default, common operations like
insert
, find
and delete
are prepared before-hand for each entity class. For update
and all operations with timestamp, since the updated fields and timestamp value are only known at runtime, Achilless will prepare the statements only on the fly and save them into a Guava LRU cache.
The default size is 10000
entries. Once the limit is reached, oldest prepared statements are evicted, causing Achilles to re-prepare them and get warnings from the Java Driver.
You can get details on the LRU cache state by putting the logger info.archinnov.achilles.internal.statement.cache.CacheManager
on DEBUG
*
* -
* STATEMENTS_CACHE (OPTIONAL): provide an instance of the class {@link info.archinnov.achilles.internals.cache.StatementsCache}
* to store all prepared statements. This option is useful for unit testing to avoid re-preparing many times the same prepared statements
*
*
* Remark: if your provide the statement cache object yourself, the parameter PREPARED_STATEMENTS_CACHE_SIZE will be ignored
*
*
*
*
*
* Strategies
*
* -
* GLOBAL_INSERT_STRATEGY (OPTIONAL): choose between
InsertStrategy.ALL_FIELDS
and InsertStrategy.NOT_NULL_FIELDS
.
Default value is ConfigurationParameters.InsertStrategy.ALL_FIELDS
.
For more details, please check Insert Strategy
*
* -
* GLOBAL_NAMING_STRATEGY (OPTIONAL): choose between
NamingStrategy.LOWER_CASE
, NamingStrategy.SNAKE_CASE
and NamingStrategy.CASE_SENSITIVE
.
Default value is NamingStrategy.LOWER_CASE
.
For more details, please check Naming Strategy
*
*
*
*
* Bean Factory
*
*
*
* -
* DEFAULT_BEAN_FACTORY (OPTIONAL): inject the default bean factory to instantiate new entities and UDT classes.
* The implementation class should implement the interface {@link info.archinnov.achilles.type.factory.BeanFactory}
* The default implementation is straightforward
*
* {@literal @}Override
* public T newInstance(Class clazz) {
* try{
* return clazz.newInstance();
* } catch (InstantiationException | IllegalAccessException e) {
* ....
* }
* }
*
*
*
*
*
* Schema Name Provider
*
* -
* SCHEMA_NAME_PROVIDER (OPTIONAL): define a schema name provider to bind dynamically
* an entity to a keyspace/table name at runtime. This feature is useful mostly in a multi-tenant context
*
*
*
*
* Asynchronous Operations
*
* - EXECUTOR_SERVICE (OPTIONAL): define the executor service (thread pool) to be used by Achilles for its internal asynchronous operations.
* By default, the thread pool is configured as follow:
*
* new ThreadPoolExecutor(5, 20, 60, TimeUnit.SECONDS,
* new LinkedBlockingQueue(1000),
* new DefaultExecutorThreadFactory())
*
*
* -
* DEFAULT_EXECUTOR_SERVICE_MIN_THREAD (OPTIONAL): define the minimum thread count for the executor service used by Achilles for its internal asynchronous operations.
* The thread pool will configured as follow:
*
* new ThreadPoolExecutor(DEFAULT_EXECUTOR_SERVICE_MIN_THREAD, 20, 60, TimeUnit.SECONDS,
* new LinkedBlockingQueue(1000),
* new DefaultExecutorThreadFactory())
*
*
* -
* DEFAULT_EXECUTOR_SERVICE_MAX_THREAD (OPTIONAL): define the maximum thread count for the executor service used by Achilles for its internal asynchronous operations.
* The thread pool will configured as follow:
*
* new ThreadPoolExecutor(5, DEFAULT_EXECUTOR_SERVICE_MAX_THREAD, 60, TimeUnit.SECONDS,
* new LinkedBlockingQueue(1000),
* new DefaultExecutorThreadFactory())
*
*
* -
* DEFAULT_EXECUTOR_SERVICE_THREAD_KEEPALIVE (OPTIONAL): define the duration in seconds during which a thread is kept alive before being destroyed, on the executor service used by Achilles for its internal asynchronous operations.
* The thread pool will configured as follow:
*
* new ThreadPoolExecutor(5, 20, DEFAULT_EXECUTOR_SERVICE_THREAD_KEEPALIVE, TimeUnit.SECONDS,
* new LinkedBlockingQueue(1000),
* new DefaultExecutorThreadFactory())
*
*
* -
* DEFAULT_EXECUTOR_SERVICE_QUEUE_SIZE (OPTIONAL): define the size of the LinkedBlockingQueue used by the executor service used by Achilles for its internal asynchronous operations.
* The thread pool will configured as follow:
*
* new ThreadPoolExecutor(5, 20, 60, TimeUnit.SECONDS,
* new LinkedBlockingQueue(DEFAULT_EXECUTOR_SERVICE_QUEUE_SIZE),
* new DefaultExecutorThreadFactory())
*
*
* -
* DEFAULT_EXECUTOR_SERVICE_THREAD_FACTORY (OPTIONAL): define the thread factory used by Achilles for its internal asynchronous operations.
* The thread pool will configured as follow:
*
* new ThreadPoolExecutor(5, 20, 60, TimeUnit.SECONDS,
* new LinkedBlockingQueue(1000),
* DEFAULT_EXECUTOR_SERVICE_THREAD_FACTORY)
*
*
*
For more details, please check Asynchronous Operations
*/
public enum ConfigurationParameters {
NATIVE_SESSION("achilles.cassandra.native.session"),
KEYSPACE_NAME("achilles.cassandra.keyspace.name"),
JACKSON_MAPPER_FACTORY("achilles.json.jackson.mapper.factory"),
JACKSON_MAPPER("achilles.json.jackson.mapper"),
CONSISTENCY_LEVEL_READ_DEFAULT("achilles.consistency.read.default"),
CONSISTENCY_LEVEL_WRITE_DEFAULT("achilles.consistency.write.default"),
CONSISTENCY_LEVEL_SERIAL_DEFAULT("achilles.consistency.serial.default"),
CONSISTENCY_LEVEL_READ_MAP("achilles.consistency.read.map"),
CONSISTENCY_LEVEL_WRITE_MAP("achilles.consistency.write.map"),
CONSISTENCY_LEVEL_SERIAL_MAP("achilles.consistency.serial.map"),
EVENT_INTERCEPTORS("achilles.event.interceptors"),
FORCE_SCHEMA_GENERATION("achilles.ddl.force.schema.generation"),
MANAGED_ENTITIES("achilles.managed.entities"),
BEAN_VALIDATION_ENABLE("achilles.bean.validation.enable"),
POST_LOAD_BEAN_VALIDATION_ENABLE("achilles.post.load.bean.validation.enable"),
BEAN_VALIDATION_VALIDATOR("achilles.bean.validation.validator"),
PREPARED_STATEMENTS_CACHE_SIZE("achilles.prepared.statements.cache.size"),
DEFAULT_BEAN_FACTORY("achilles.bean.factory"),
GLOBAL_INSERT_STRATEGY("achilles.global.insert.strategy"),
GLOBAL_NAMING_STRATEGY("achilles.global.naming.strategy"),
SCHEMA_NAME_PROVIDER("achilles.schema.name.provider"),
EXECUTOR_SERVICE("achilles.executor.service"),
STATEMENTS_CACHE("achilles.statements.cache"),
RUNTIME_CODECS("achilles.runtime.codecs"),
DEFAULT_EXECUTOR_SERVICE_MIN_THREAD("achilles.executor.service.default.thread.min"),
DEFAULT_EXECUTOR_SERVICE_MAX_THREAD("achilles.executor.service.default.thread.max"),
DEFAULT_EXECUTOR_SERVICE_THREAD_KEEPALIVE("achilles.executor.service.default.thread.keepalive"),
DEFAULT_EXECUTOR_SERVICE_QUEUE_SIZE("achilles.executor.service.default.queue.size"),
DEFAULT_EXECUTOR_SERVICE_THREAD_FACTORY("achilles.executor.service.thread.factory");
private String label;
ConfigurationParameters(String label) {
this.label = label;
}
/**
* Small utility method that resolves a configuration based on its label.
*
* @param label the configuration label that would be populated in the map.
* @return the label in ConfigurationParameters format, or null if no match found.
*/
public static ConfigurationParameters fromLabel(String label) {
for (ConfigurationParameters param : ConfigurationParameters.values()) {
if (param.label.equals(label)) {
return param;
}
}
return null;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy