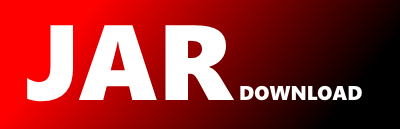
info.archinnov.achilles.annotations.Codec Maven / Gradle / Ivy
/*
* Copyright (C) 2012-2016 DuyHai DOAN
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package info.archinnov.achilles.annotations;
import java.lang.annotation.*;
/**
* Transform a custom Java type into one of native types supported by the Java driver
*
* The Codec class provided should implement the {@link info.archinnov.achilles.type.codec.Codec} interface.
*
*
*
* Let's consider the following codec transforming a Long to a String
*
* public class LongToString implements Codec<Long,String> {
* {@literal @}Override
* public Class sourceType() {
* return Long.class;
* }
* {@literal @}Override
* public Class targetType() {
* return String.class;
* }
* {@literal @}Override
* public String encode(Long fromJava) throws AchillesTranscodingException {
* return fromJava.toString();
* }
* {@literal @}Override
* public Long decode(String fromCassandra) throws AchillesTranscodingException {
* return Long.parseLong(fromCassandra);
* }
* }
*
*
* Example of simple Long type to String type transformation
*
* {@literal @}Column
* {@literal @}Codec(LongToString.class)
* private Long longToString;
*
*
* Example of List<Long> to List<String> transformation
*
* {@literal @}Column
* private List<{@literal @}Codec(LongToString.class) Long> listOfLongToString;
*
*
* Example of Set<Long> to Set<String> transformation
*
* {@literal @}Column
* private Set<{@literal @}Codec(LongToString.class) Long> setOfLongToString;
*
*
* Example of key Map transformation: Map<Long, Double> to Map<String, Double>
*
* {@literal @}Column
* private Map<{@literal @}Codec(LongToString.class) Long, Double> mapKeyTransformation;
*
*
* Example of value Map transformation: Map<Integer, Long> to Map<Integer, String>
*
* {@literal @}Column
* private Map<Integer,{@literal @}Codec(LongToString.class)Long > mapValueTransformation;
*
*
* Example of key/value Map transformation: Map<Long, Long> to Map<String, String>
*
* {@literal @}Column
* private Map<{@literal @}Codec(LongToString.class) Long, {@literal @}Codec(LongToString.class) Long > mapKeyValueTransformation;
*
*
* You can also have nested usage of {@literal @}Codec. The nesting level can be arbitrary and does not matter
*
* 2-levels nesting
*
* {@literal @}Column
* private Tuple2<String, Map<Integer, {@literal @}Codec(LongToString.class) Long>> nested1Level
*
*
* 3-levels nesting
*
* {@literal @}Column
* private Tuple2<String, Map<Integer, List<{@literal @}Codec(LongToString.class) Long>>> nested2Level
*
*
*/
@Retention(RetentionPolicy.RUNTIME)
@Target({ElementType.FIELD, ElementType.TYPE_USE, ElementType.TYPE_PARAMETER})
@Documented
public @interface Codec {
/**
* Codec Implementation class. The provided Codec class should implement the {@link info.archinnov.achilles.type.codec.Codec} interface.
*/
Class extends info.archinnov.achilles.type.codec.Codec> value();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy