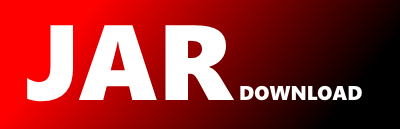
info.archinnov.achilles.annotations.FunctionRegistry Maven / Gradle / Ivy
/*
* Copyright (C) 2012-2016 DuyHai DOAN
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package info.archinnov.achilles.annotations;
import java.lang.annotation.*;
/**
*
* Marks a class as a function registry and let Achilles manage it
*
* {@literal @}FunctionRegistry
* public interface MyFunctions {
*
* Integer sumOf(int val1, int val2);
*
* Long toLong(Date javaDate);
* }
*
*
* Note: it is possible to declare several function registries in your source code,
* just annotate them with {@literal @}FunctionRegistry
*
* Warning: it is not possible to declare 2 different functions with the same name and signature in the same keyspace
* Achilles will raise a compilation error when encountering such case. Ex:
*
*
*
* {@literal @}FunctionRegistry
* public interface MyFunctionRegistry {
*
*
* String toString(long value);
*
* String toString(int value); // OK because parameter type is different
*
* String toString(long value); // KO because same signature as the first function
* }
*
*
* Remark 1: functions return types cannot be primitive, use boxed types instead
*
*
* Remark 2: Achilles' codec system also applies for function parameters and return type
*
*
*
* {@literal @}FunctionRegistry
* public interface FunctionsWithCodecSystemRegistry {
*
* // CQL function signature = listtojson(consistencylevels list<text>), returns text
* String listToJson(List<@Enumerated ConsistencyLevel> consistencyLevels);
*
* // CQL function signature = getinvalue(input text), returns text
* {@literal @}Codec(IntToString.class) String getIntValue(String input);
*
* }
*
* Remark 3: functions name and parameters' name are lower-cased by Cassandra automatically
*
*
* @see Function Registry
*/
@Retention(RetentionPolicy.RUNTIME)
@Target({ElementType.TYPE})
@Documented
public @interface FunctionRegistry {
/**
* (Optional) The name of the keyspace in which the declared functions belong to.
* If not set explicitly, Achilles will use the current
* keyspace of the java driver Session object.
*
*
* {@literal @}FunctionRegistry(keyspace="production")
* public class MyFunctions {...}
*
*/
String keyspace() default "";
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy