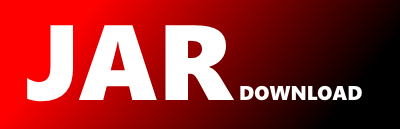
info.archinnov.achilles.annotations.Index Maven / Gradle / Ivy
/*
* Copyright (C) 2012-2016 DuyHai DOAN
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package info.archinnov.achilles.annotations;
import java.lang.annotation.*;
/**
* Annotation for secondary index. Example
*
* {@literal @}Table(table = "my_entity")
* public class MyEntity {
* //Simple index
* {@literal @}Column
* {@literal @}Index
* private String countryCode;
* //Simple index with custom name
* {@literal @}Column("custom_name")
* {@literal @}Index(name = "country_code_idx")
* private String customName;
* //Index on collection
* {@literal @}Column
* {@literal @}Index
* private List<String> indexedList;
* //Full index on collection because of the usage of {@literal @}Frozen
* {@literal @}Column
* {@literal @}Frozen
* {@literal @}Index
* private List<String> indexedFullList;
* //Index on map key
* {@literal @}Column("indexed_map_key")
* private Map<{@literal @}Index String, Long> indexOnMapKey;
* //Index on map entry
* {@literal @}Column("index_map_entry")
* {@literal @}Index
* private Map<String, Long> indexOnMapEntry;
* //Custom index
* {@literal @}Column
* {@literal @}Index(indexClassName = "com.myproject.SecondaryIndex", indexOptions = "{'key1': 'value1', 'key2': 'value2'}")
* private String custom;
* ...
* }
*
* The above code would produce the following CQL script for index creation:
*
* CREATE INDEX IF NOT EXISTS ON my_entity(countryCode);
* CREATE INDEX country_code_idx IF NOT EXISTS ON my_entity(custom_name);
* CREATE INDEX IF NOT EXISTS ON my_entity(indexedlist);
* CREATE INDEX IF NOT EXISTS ON my_entity(FULL(indexedfulllist));
* CREATE INDEX IF NOT EXISTS ON my_entity(KEYS(indexed_map_key));
* CREATE INDEX IF NOT EXISTS ON my_entity(ENTRY(index_map_entry));
* CREATE CUSTOM my_entity_custom_index INDEX IF NOT EXISTS
* ON my_entity(custom)
* USING com.myproject.SecondaryIndex
* WITH OPTIONS = {'key1': 'value1', 'key2': 'value2'};
*
* /
*
* @see @Index
*/
@Retention(RetentionPolicy.RUNTIME)
@Target({ElementType.FIELD, ElementType.TYPE_USE})
@Documented
public @interface Index {
/**
* Optional.
* Define the name of the secondary index. If not set, defaults to table name_field name_index
*
* {@literal @}Table
* public class User {
* ...
* {@literal @}Index(name = "country_code_index")
* {@literal @}Column
* private String countryCode;
* ...
* }
*
*
* If the index name was not set above, it would default to user_countrycode_index
*/
String name() default "";
/**
* Optional. The class name of your custom secondary index implementation
*/
String indexClassName() default "";
/**
* Optional. The options for your custom secondary index.
* The indexOptions should be provided using the JSON map style. Example:
*
* {'key1': 'property1', 'key2': 'property2', ...}
*/
String indexOptions() default "";
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy