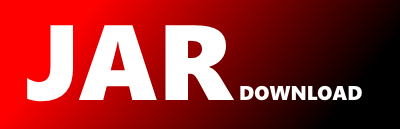
info.archinnov.achilles.dao.ThriftGenericEntityDao Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of achilles-thrift Show documentation
Show all versions of achilles-thrift Show documentation
Thrift implementation for Achilles using Hector library
package info.archinnov.achilles.dao;
import static info.archinnov.achilles.serializer.ThriftSerializerUtils.*;
import info.archinnov.achilles.consistency.AchillesConsistencyLevelPolicy;
import info.archinnov.achilles.type.Pair;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import me.prettyprint.hector.api.Cluster;
import me.prettyprint.hector.api.Keyspace;
import me.prettyprint.hector.api.beans.AbstractComposite.ComponentEquality;
import me.prettyprint.hector.api.beans.Composite;
import me.prettyprint.hector.api.beans.HColumn;
import me.prettyprint.hector.api.beans.Row;
import me.prettyprint.hector.api.beans.Rows;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* ThriftGenericEntityDao
*
* @author DuyHai DOAN
*
*/
public class ThriftGenericEntityDao extends ThriftAbstractDao
{
private static final Logger log = LoggerFactory.getLogger(ThriftGenericEntityDao.class);
protected static final byte[] START_EAGER = new byte[]
{
0
};
protected static final byte[] END_EAGER = new byte[]
{
20
};
private Composite startCompositeForEagerFetch;
private Composite endCompositeForEagerFetch;
protected ThriftGenericEntityDao() {
this.initComposites();
}
protected ThriftGenericEntityDao(Pair rowkeyAndValueClasses) {
this.initComposites();
super.rowkeyAndValueClasses = rowkeyAndValueClasses;
}
public ThriftGenericEntityDao(Cluster cluster, Keyspace keyspace, String cf,
AchillesConsistencyLevelPolicy consistencyPolicy, Pair rowkeyAndValueClasses)
{
super(cluster, keyspace, cf, consistencyPolicy, rowkeyAndValueClasses);
this.initComposites();
columnNameSerializer = COMPOSITE_SRZ;
log
.debug("Initializing GenericEntityDao for key serializer '{}', composite comparator and value serializer '{}'",
this.rowSrz().getComparatorType().getTypeName(), STRING_SRZ
.getComparatorType()
.getTypeName());
}
public List> eagerFetchEntity(K key)
{
log.trace("Eager fetching properties for column family {} ", columnFamily);
return this.findColumnsRange(key, startCompositeForEagerFetch, endCompositeForEagerFetch,
false, Integer.MAX_VALUE);
}
public Map>> eagerFetchEntities(List keys)
{
log.trace("Eager fetching properties for multiple entities in column family {} ",
columnFamily);
Map>> map = new HashMap>>();
Rows rows = this.multiGetSliceRange(keys,
startCompositeForEagerFetch, endCompositeForEagerFetch, false, Integer.MAX_VALUE);
for (Row row : rows)
{
List> columns = new ArrayList>();
for (HColumn column : row.getColumnSlice().getColumns())
{
columns.add(new Pair(column.getName(), column.getValue()));
}
map.put(row.getKey(), columns);
}
return map;
}
private void initComposites()
{
startCompositeForEagerFetch = new Composite();
startCompositeForEagerFetch.addComponent(0, START_EAGER, ComponentEquality.EQUAL);
endCompositeForEagerFetch = new Composite();
endCompositeForEagerFetch.addComponent(0, END_EAGER, ComponentEquality.GREATER_THAN_EQUAL);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy