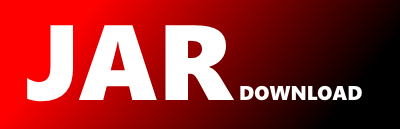
info.archinnov.achilles.entity.manager.ThriftEntityManagerFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of achilles-thrift Show documentation
Show all versions of achilles-thrift Show documentation
Thrift implementation for Achilles using Hector library
package info.archinnov.achilles.entity.manager;
import info.archinnov.achilles.configuration.ArgumentExtractor;
import info.archinnov.achilles.configuration.ThriftArgumentExtractor;
import info.archinnov.achilles.consistency.AchillesConsistencyLevelPolicy;
import info.archinnov.achilles.consistency.ThriftConsistencyLevelPolicy;
import info.archinnov.achilles.context.ThriftDaoContext;
import info.archinnov.achilles.context.ThriftDaoContextBuilder;
import info.archinnov.achilles.table.ThriftTableCreator;
import info.archinnov.achilles.type.ConsistencyLevel;
import java.util.Collections;
import java.util.Map;
import me.prettyprint.hector.api.Cluster;
import me.prettyprint.hector.api.Keyspace;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* ThriftEntityManagerFactory
*
* @author DuyHai DOAN
*
*/
public class ThriftEntityManagerFactory extends AchillesEntityManagerFactory
{
private static final Logger log = LoggerFactory.getLogger(ThriftEntityManagerFactory.class);
private Cluster cluster;
private Keyspace keyspace;
private ThriftDaoContext thriftDaoContext;
/**
* Create a new ThriftEntityManagerFactoryImpl with a configuration map
*
* @param configurationMap
*
* The configurationMap accepts the following properties:
*
* Entity Packages
*
*
* - "achilles.entity.packages" (MANDATORY): list of java packages for entity scanning, separated by comma.
*
*   Example: "my.project.entity,another.project.entity"
*
*
* Cluster and Keyspace
*
*
* - "achilles.cassandra.host": hostname/port of the Cassandra cluster
*
*   Example: localhost:9160
*
*
* - "achilles.cassandra.cluster.name": Cassandra cluster name
* - "achilles.cassandra.keyspace.name": Cassandra keyspace name
*
*
* - "achilles.cassandra.cluster": instance of pre-configured me.prettyprint.hector.api.Cluster object from Hector API
* - "achilles.cassandra.keyspace": instance of pre-configured me.prettyprint.hector.api.Keyspace object from Hector API
*
*
* Either "achilles.cassandra.cluster" or "achilles.cassandra.host"/"achilles.cassandra.cluster.name" parameters should be provided
*
* Either "achilles.cassandra.keyspace" or "achilles.cassandra.keyspace.name" parameters should be provided
*
*
* DDL Parameter
*
*
* - "achilles.ddl.force.column.family.creation" (OPTIONAL): create missing column families for entities if they are not found. Default = 'false'.
*
* If "achilles.ddl.force.column.family.creation" = false and no column family is found for any entity, Achilles will raise an
* AchillesInvalidColumnFamilyException
*
*
*
*
* JSON Serialization
*
*
* - "achilles.json.object.mapper.factory" (OPTIONAL): an implementation of the info.archinnov.achilles.json.ObjectMapperFactory interface to build custom
* Jackson ObjectMapper based on entity class
*
*
* - achilles.json.object.mapper (OPTIONAL): default Jackson ObjectMapper to use for serializing entities
*
* If both "achilles.json.object.mapper.factory" and "achilles.json.object.mapper" parameters are provided, Achilles will ignore the "achilles.json.object.mapper" value and
* use the "achilles.json.object.mapper.factory"
*
* If none is provided, Achilles will use a default Jackson ObjectMapper with the following configuration:
*
*
* - SerializationInclusion = Inclusion.NON_NULL
* - DeserializationConfig.Feature.FAIL_ON_UNKNOWN_PROPERTIES = false
* - AnnotationIntrospector pair : primary = JacksonAnnotationIntrospector, secondary = JaxbAnnotationIntrospector
*
*
*
*
* Consistency Level
*
*
* - "achilles.consistency.read.default" (OPTIONAL): default read consistency level for all entities
*
*
* - "achilles.consistency.write.default" (OPTIONAL): default write consistency level for all entities
*
*
* - "achilles.consistency.read.map" (OPTIONAL): map(String,String) of read consistency levels for column families
*
* Example:
*
* "columnFamily1" -> "ONE"
* "columnFamily2" -> "QUORUM"
* ...
*
*
* - "achilles.consistency.write.map" (OPTIONAL): map(String,String) of write consistency levels for column families
*
* Example:
*
* "columnFamily1" -> "ALL"
* "columnFamily2" -> "EACH_QUORUM"
* ...
*
*
*
*
* Join consistency
*
*
* - "achilles.consistency.join.check" (OPTIONAL): whether check for join entity in Cassandra before inserting join primary key
*
* Warning: enable this option guarantees stronger data consistency but with the expense of read-before-write for each join entity insertion
*
*
*/
public ThriftEntityManagerFactory(Map configurationMap) {
super(configurationMap, new ThriftArgumentExtractor());
ThriftArgumentExtractor thriftArgumentExtractor = new ThriftArgumentExtractor();
cluster = thriftArgumentExtractor.initCluster(configurationMap);
keyspace = thriftArgumentExtractor.initKeyspace(cluster,
(ThriftConsistencyLevelPolicy) configContext.getConsistencyPolicy(),
configurationMap);
log
.info("Initializing Achilles ThriftEntityManagerFactory for cluster '{}' and keyspace '{}' ",
cluster.getName(), keyspace.getKeyspaceName());
boolean hasSimpleCounter = bootstrap();
new ThriftTableCreator(cluster, keyspace).validateOrCreateColumnFamilies(entityMetaMap,
configContext, hasSimpleCounter);
thriftDaoContext = new ThriftDaoContextBuilder().buildDao(cluster, keyspace, entityMetaMap,
configContext, hasSimpleCounter);
}
/**
* Create a new ThriftEntityManager
*
* @return ThriftEntityManager
*/
public ThriftEntityManager createEntityManager()
{
log.info("Create new Thrift-based Entity Manager ");
return new ThriftEntityManager(this, Collections.unmodifiableMap(entityMetaMap), //
thriftDaoContext, configContext);
}
@Override
protected AchillesConsistencyLevelPolicy initConsistencyLevelPolicy(
Map configurationMap, ArgumentExtractor argumentExtractor)
{
log.info("Initializing new Achilles Configurable Consistency Level Policy from arguments ");
ConsistencyLevel defaultReadConsistencyLevel = argumentExtractor
.initDefaultReadConsistencyLevel(configurationMap);
ConsistencyLevel defaultWriteConsistencyLevel = argumentExtractor
.initDefaultWriteConsistencyLevel(configurationMap);
Map readConsistencyMap = argumentExtractor
.initReadConsistencyMap(configurationMap);
Map writeConsistencyMap = argumentExtractor
.initWriteConsistencyMap(configurationMap);
return new ThriftConsistencyLevelPolicy(defaultReadConsistencyLevel,
defaultWriteConsistencyLevel, readConsistencyMap, writeConsistencyMap);
}
protected void setThriftDaoContext(ThriftDaoContext thriftDaoContext)
{
this.thriftDaoContext = thriftDaoContext;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy