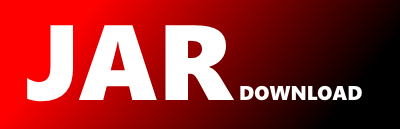
info.archinnov.achilles.iterator.ThriftCounterKeyValueIteratorImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of achilles-thrift Show documentation
Show all versions of achilles-thrift Show documentation
Thrift implementation for Achilles using Hector library
package info.archinnov.achilles.iterator;
import info.archinnov.achilles.context.ThriftPersistenceContext;
import info.archinnov.achilles.entity.metadata.PropertyMeta;
import info.archinnov.achilles.iterator.factory.ThriftKeyValueFactory;
import info.archinnov.achilles.type.Counter;
import info.archinnov.achilles.type.KeyValue;
import info.archinnov.achilles.type.KeyValueIterator;
import java.util.NoSuchElementException;
import me.prettyprint.hector.api.beans.Composite;
import me.prettyprint.hector.api.beans.HCounterColumn;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* ThriftCounterKeyValueIteratorImpl
*
* @author DuyHai DOAN
*
*/
public class ThriftCounterKeyValueIteratorImpl implements KeyValueIterator
{
private static final Logger log = LoggerFactory
.getLogger(ThriftCounterKeyValueIteratorImpl.class);
private ThriftKeyValueFactory factory = new ThriftKeyValueFactory();
private ThriftPersistenceContext context;
private ThriftAbstractSliceIterator> achillesSliceIterator;
private PropertyMeta propertyMeta;
public ThriftCounterKeyValueIteratorImpl(ThriftPersistenceContext context,
ThriftAbstractSliceIterator> columnSliceIterator,
PropertyMeta propertyMeta)
{
this.context = context;
this.achillesSliceIterator = columnSliceIterator;
this.propertyMeta = propertyMeta;
}
@Override
public boolean hasNext()
{
log.trace("Does the {} has next value ? ", achillesSliceIterator.type());
return this.achillesSliceIterator.hasNext();
}
@Override
public KeyValue next()
{
log.trace("Get next key/counter value from the {} ", achillesSliceIterator.type());
if (this.achillesSliceIterator.hasNext())
{
HCounterColumn column = this.achillesSliceIterator.next();
return factory.createCounterKeyValue(context, propertyMeta, column);
}
else
{
throw new NoSuchElementException();
}
}
@Override
public K nextKey()
{
log.trace("Get next key from the {} ", achillesSliceIterator.type());
if (this.achillesSliceIterator.hasNext())
{
HCounterColumn column = this.achillesSliceIterator.next();
return factory.createCounterKey(propertyMeta, column);
}
else
{
throw new NoSuchElementException();
}
}
@Override
public Counter nextValue()
{
log.trace("Get next counter value from the {} ", achillesSliceIterator.type());
if (this.achillesSliceIterator.hasNext())
{
HCounterColumn column = this.achillesSliceIterator.next();
return factory.createCounterValue(context, propertyMeta, column);
}
else
{
throw new NoSuchElementException();
}
}
@Override
public Integer nextTtl()
{
throw new UnsupportedOperationException("Ttl does not exist for counter type");
}
@Override
public void remove()
{
throw new UnsupportedOperationException(
"Cannot remove counter value. Please set a its value to 0 instead of removing it");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy