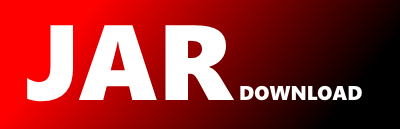
info.archinnov.achilles.iterator.factory.ThriftCompositeTransformer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of achilles-thrift Show documentation
Show all versions of achilles-thrift Show documentation
Thrift implementation for Achilles using Hector library
package info.archinnov.achilles.iterator.factory;
import static info.archinnov.achilles.helper.ThriftLoggerHelper.format;
import info.archinnov.achilles.context.ThriftPersistenceContext;
import info.archinnov.achilles.entity.metadata.PropertyMeta;
import info.archinnov.achilles.entity.operations.ThriftEntityProxifier;
import info.archinnov.achilles.helper.ThriftPropertyHelper;
import info.archinnov.achilles.proxy.wrapper.builder.ThriftCounterWrapperBuilder;
import info.archinnov.achilles.type.Counter;
import info.archinnov.achilles.type.KeyValue;
import me.prettyprint.cassandra.serializers.SerializerTypeInferer;
import me.prettyprint.hector.api.Serializer;
import me.prettyprint.hector.api.beans.Composite;
import me.prettyprint.hector.api.beans.HColumn;
import me.prettyprint.hector.api.beans.HCounterColumn;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.google.common.base.Function;
/**
* ThriftCompositeTransformer
*
* @author DuyHai DOAN
*
*/
public class ThriftCompositeTransformer
{
private static final Logger log = LoggerFactory.getLogger(ThriftCompositeTransformer.class);
private ThriftPropertyHelper helper = new ThriftPropertyHelper();
private ThriftEntityProxifier proxifier = new ThriftEntityProxifier();
public Function, K> buildKeyTransformer(
final PropertyMeta propertyMeta)
{
return new Function, K>()
{
public K apply(HColumn hColumn)
{
return buildKey(propertyMeta, hColumn);
}
};
}
public Function, V> buildValueTransformer(
final PropertyMeta propertyMeta)
{
return new Function, V>()
{
public V apply(HColumn hColumn)
{
return propertyMeta.castValue(hColumn.getValue());
}
};
}
public Function, ?> buildRawValueTransformer()
{
return new Function, Object>()
{
public Object apply(HColumn hColumn)
{
return hColumn.getValue();
}
};
}
public Function, Integer> buildTtlTransformer()
{
return new Function, Integer>()
{
public Integer apply(HColumn hColumn)
{
return hColumn.getTtl();
}
};
}
public Function, KeyValue> buildKeyValueTransformer(
final ThriftPersistenceContext context, final PropertyMeta propertyMeta)
{
return new Function, KeyValue>()
{
public KeyValue apply(HColumn hColumn)
{
return buildKeyValue(context, propertyMeta, hColumn);
}
};
}
public KeyValue buildKeyValue(ThriftPersistenceContext context,
PropertyMeta propertyMeta, HColumn hColumn)
{
K key = buildKey(propertyMeta, hColumn);
V value = this.buildValue(context, propertyMeta, hColumn);
int ttl = hColumn.getTtl();
KeyValue keyValue = new KeyValue(key, value, ttl);
if (log.isTraceEnabled())
{
log.trace("Built key/value from {} = {}",
format(hColumn.getName()) + ":" + hColumn.getValue(), keyValue);
}
return keyValue;
}
public V buildValue(ThriftPersistenceContext context, PropertyMeta propertyMeta,
HColumn hColumn)
{
V value = hColumn.getValue();
if (propertyMeta.isJoin())
{
ThriftPersistenceContext joinContext = context.newPersistenceContext(
propertyMeta.joinMeta(), value);
value = proxifier.buildProxy((V) hColumn.getValue(), joinContext);
}
else
{
value = propertyMeta.castValue(hColumn.getValue());
}
if (log.isTraceEnabled())
{
log.trace("Built value from {} = {}",
format(hColumn.getName()) + ":" + hColumn.getValue(), value);
}
return value;
}
public K buildKey(PropertyMeta propertyMeta, HColumn hColumn)
{
K key;
Serializer keySerializer = SerializerTypeInferer.getSerializer(propertyMeta
.getKeyClass());
if (propertyMeta.isSingleKey())
{
key = (K) hColumn.getName().get(0, keySerializer);
}
else
{
key = helper
.buildMultiKeyFromComposite(propertyMeta, hColumn.getName().getComponents());
}
if (log.isTraceEnabled())
{
log.trace("Built key from {} = {}", format(hColumn.getName()), key);
}
return key;
}
public Function, KeyValue> buildCounterKeyValueTransformer(
final ThriftPersistenceContext context, final PropertyMeta propertyMeta)
{
return new Function, KeyValue>()
{
@Override
public KeyValue apply(HCounterColumn hColumn)
{
return buildCounterKeyValue(context, propertyMeta, hColumn);
}
};
}
public KeyValue buildCounterKeyValue(ThriftPersistenceContext context,
PropertyMeta propertyMeta, HCounterColumn hColumn)
{
K key = buildCounterKey(propertyMeta, hColumn);
Counter value = buildCounterValue(context, propertyMeta, hColumn);
return new KeyValue(key, value, 0);
}
public K buildCounterKey(PropertyMeta propertyMeta, HCounterColumn hColumn)
{
K key;
Serializer keySerializer = SerializerTypeInferer.getSerializer(propertyMeta
.getKeyClass());
if (propertyMeta.isSingleKey())
{
key = hColumn.getName().get(0, keySerializer);
}
else
{
key = helper
.buildMultiKeyFromComposite(propertyMeta, hColumn.getName().getComponents());
}
if (log.isTraceEnabled())
{
log.trace("Built counter key from {} = {}", format(hColumn.getName()), key);
}
return key;
}
public Counter buildCounterValue(ThriftPersistenceContext context,
PropertyMeta propertyMeta, HCounterColumn hColumn)
{
return ThriftCounterWrapperBuilder.builder(context) //
.columnName(hColumn.getName())
//
.counterDao(context.findWideRowDao(propertyMeta.getExternalTableName()))
//
.readLevel(propertyMeta.getReadConsistencyLevel())
//
.writeLevel(propertyMeta.getWriteConsistencyLevel())
//
.key(context.getPrimaryKey())
//
.build();
}
public Function, Counter> buildCounterValueTransformer(
final ThriftPersistenceContext context, final PropertyMeta propertyMeta)
{
return new Function, Counter>()
{
@Override
public Counter apply(HCounterColumn hColumn)
{
return buildCounterValue(context, propertyMeta, hColumn);
}
};
}
public Function, K> buildCounterKeyTransformer(
final PropertyMeta propertyMeta)
{
return new Function, K>()
{
@Override
public K apply(HCounterColumn hColumn)
{
return buildCounterKey(propertyMeta, hColumn);
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy