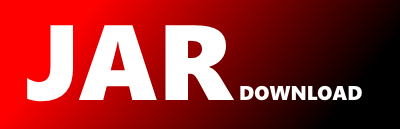
info.archinnov.achilles.proxy.wrapper.ThriftCounterWideMapWrapper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of achilles-thrift Show documentation
Show all versions of achilles-thrift Show documentation
Thrift implementation for Achilles using Hector library
package info.archinnov.achilles.proxy.wrapper;
import static info.archinnov.achilles.helper.ThriftLoggerHelper.format;
import static me.prettyprint.hector.api.beans.AbstractComposite.ComponentEquality.EQUAL;
import info.archinnov.achilles.composite.ThriftCompositeFactory;
import info.archinnov.achilles.context.execution.SafeExecutionContext;
import info.archinnov.achilles.dao.ThriftGenericWideRowDao;
import info.archinnov.achilles.entity.metadata.PropertyMeta;
import info.archinnov.achilles.helper.ThriftPropertyHelper;
import info.archinnov.achilles.iterator.ThriftCounterSliceIterator;
import info.archinnov.achilles.iterator.factory.ThriftIteratorFactory;
import info.archinnov.achilles.iterator.factory.ThriftKeyValueFactory;
import info.archinnov.achilles.proxy.wrapper.builder.ThriftCounterWrapperBuilder;
import info.archinnov.achilles.type.ConsistencyLevel;
import info.archinnov.achilles.type.Counter;
import info.archinnov.achilles.type.KeyValue;
import info.archinnov.achilles.type.KeyValueIterator;
import java.util.List;
import me.prettyprint.hector.api.beans.Composite;
import me.prettyprint.hector.api.beans.HCounterColumn;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* ThriftCounterWideMapWrapper
*
* @author DuyHai DOAN
*
*/
public class ThriftCounterWideMapWrapper extends ThriftAbstractWideMapWrapper
{
private static Logger log = LoggerFactory.getLogger(ThriftCounterWideMapWrapper.class);
private Object id;
private ThriftGenericWideRowDao wideMapCounterDao;
private PropertyMeta propertyMeta;
private ThriftPropertyHelper thriftPropertyHelper;
private ThriftKeyValueFactory thriftKeyValueFactory;
private ThriftIteratorFactory thriftIteratorFactory;
private ThriftCompositeFactory thriftCompositeFactory;
@Override
public Counter get(K key)
{
log.trace("Get counter value having key {}", key);
final Composite comp = thriftCompositeFactory.createForQuery(propertyMeta, key, EQUAL);
Long counterValue = context.executeWithReadConsistencyLevel(
new SafeExecutionContext()
{
@Override
public Long execute()
{
return wideMapCounterDao.getCounterValue(id, comp);
}
}, propertyMeta.getReadConsistencyLevel());
if (counterValue != null)
{
return ThriftCounterWrapperBuilder.builder(context) //
.columnName(comp)
.counterDao(wideMapCounterDao)
.key(id)
.readLevel(propertyMeta.getReadConsistencyLevel())
.writeLevel(propertyMeta.getWriteConsistencyLevel())
.build();
}
else
{
return null;
}
}
@Override
public void insert(K key, Counter value, int ttl)
{
throw new UnsupportedOperationException("Cannot insert counter value with ttl");
}
@Override
public void insert(K key, final Counter value)
{
log.trace("Insert counter value {} with key {}", value, key);
final Composite comp = thriftCompositeFactory.createBaseComposite(propertyMeta, key);
context.executeWithWriteConsistencyLevel(new SafeExecutionContext()
{
@Override
public Void execute()
{
wideMapCounterDao.incrementCounter(id, comp, value.get());
return null;
}
}, propertyMeta.getWriteConsistencyLevel());
}
@Override
public List> find(K start, K end, final int count, BoundingMode bounds,
final OrderingMode ordering)
{
thriftPropertyHelper.checkBounds(propertyMeta, start, end, ordering, false);
final Composite[] queryComps = thriftCompositeFactory.createForQuery(propertyMeta, start,
end, bounds, ordering);
if (log.isTraceEnabled())
{
log.trace("Find key/value pairs in range {} / {} with bounding {} and ordering {}",
format(queryComps[0]), format(queryComps[1]), bounds.name(), ordering.name());
}
List> hColumns = context.executeWithReadConsistencyLevel(
new SafeExecutionContext>>()
{
@Override
public List> execute()
{
return wideMapCounterDao.findCounterColumnsRange(id, queryComps[0],
queryComps[1], count, ordering.isReverse());
}
}, propertyMeta.getReadConsistencyLevel());
return thriftKeyValueFactory.createCounterKeyValueList(context, propertyMeta, hColumns);
}
@Override
public List findValues(K start, K end, final int count, BoundingMode bounds,
final OrderingMode ordering)
{
thriftPropertyHelper.checkBounds(propertyMeta, start, end, ordering, false);
final Composite[] queryComps = thriftCompositeFactory.createForQuery( //
propertyMeta, start, end, bounds, ordering);
if (log.isTraceEnabled())
{
log.trace("Find value in range {} / {} with bounding {} and ordering {}",
format(queryComps[0]), format(queryComps[1]), bounds.name(), ordering.name());
}
List> hColumns = context.executeWithReadConsistencyLevel(
new SafeExecutionContext>>()
{
@Override
public List> execute()
{
return wideMapCounterDao.findCounterColumnsRange(id, queryComps[0],
queryComps[1], count, ordering.isReverse());
}
}, propertyMeta.getReadConsistencyLevel());
return thriftKeyValueFactory.createCounterValueList(context, propertyMeta, hColumns);
}
@Override
public List findKeys(K start, K end, final int count, BoundingMode bounds,
final OrderingMode ordering)
{
thriftPropertyHelper.checkBounds(propertyMeta, start, end, ordering, false);
final Composite[] queryComps = thriftCompositeFactory.createForQuery( //
propertyMeta, start, end, bounds, ordering);
if (log.isTraceEnabled())
{
log.trace("Find keys in range {} / {} with bounding {} and ordering {}",
format(queryComps[0]), format(queryComps[1]), bounds.name(), ordering.name());
}
List> hColumns = context.executeWithReadConsistencyLevel(
new SafeExecutionContext>>()
{
@Override
public List> execute()
{
return wideMapCounterDao.findCounterColumnsRange(id, queryComps[0],
queryComps[1], count, ordering.isReverse());
}
}, propertyMeta.getReadConsistencyLevel());
return thriftKeyValueFactory.createCounterKeyList(propertyMeta, hColumns);
}
@Override
public KeyValueIterator iterator(K start, K end, final int count,
BoundingMode bounds, final OrderingMode ordering)
{
final Composite[] queryComps = thriftCompositeFactory.createForQuery( //
propertyMeta, start, end, bounds, ordering);
if (log.isTraceEnabled())
{
log
.trace("Iterate in range {} / {} with bounding {} and ordering {} and batch of {} elements",
format(queryComps[0]), format(queryComps[1]), bounds.name(),
ordering.name(), count);
}
ThriftCounterSliceIterator> columnSliceIterator = context
.executeWithReadConsistencyLevel(
new SafeExecutionContext>()
{
@Override
public ThriftCounterSliceIterator> execute()
{
return wideMapCounterDao.getCounterColumnsIterator(id,
queryComps[0], queryComps[1], ordering.isReverse(), count);
}
}, propertyMeta.getReadConsistencyLevel());
return thriftIteratorFactory.createCounterKeyValueIterator(context, columnSliceIterator,
propertyMeta);
}
@Override
public void remove(K key)
{
throw new UnsupportedOperationException("Cannot remove counter value");
}
@Override
public void remove(K start, K end, BoundingMode bounds)
{
throw new UnsupportedOperationException("Cannot remove counter value");
}
@Override
public void removeFirst(int count)
{
throw new UnsupportedOperationException("Cannot remove counter value");
}
@Override
public void removeLast(int count)
{
throw new UnsupportedOperationException("Cannot remove counter value");
}
@Override
public Counter get(K key, ConsistencyLevel readLevel)
{
return get(key);
}
@Override
public void insert(K key, Counter value, ConsistencyLevel writeLevel)
{
throw new UnsupportedOperationException(
"Please set runtime consistency level at Counter level instead of at WideMap level");
}
@Override
public void insert(K key, Counter value, int ttl, ConsistencyLevel writeLevel)
{
throw new UnsupportedOperationException("Cannot insert counter value with ttl");
}
@Override
public List> find(K start, K end, int count, ConsistencyLevel readLevel)
{
throw new UnsupportedOperationException(
"Please set runtime consistency level at Counter level instead of at WideMap level");
}
@Override
public List> find(K start, K end, int count, BoundingMode bounds,
OrderingMode ordering, ConsistencyLevel readLevel)
{
throw new UnsupportedOperationException(
"Please set runtime consistency level at Counter level instead of at WideMap level");
}
@Override
public List> findBoundsExclusive(K start, K end, int count,
ConsistencyLevel readLevel)
{
throw new UnsupportedOperationException(
"Please set runtime consistency level at Counter level instead of at WideMap level");
}
@Override
public List> findReverse(K start, K end, int count,
ConsistencyLevel readLevel)
{
throw new UnsupportedOperationException(
"Please set runtime consistency level at Counter level instead of at WideMap level");
}
@Override
public List> findReverseBoundsExclusive(K start, K end, int count,
ConsistencyLevel readLevel)
{
throw new UnsupportedOperationException(
"Please set runtime consistency level at Counter level instead of at WideMap level");
}
@Override
public KeyValue findFirst(ConsistencyLevel readLevel)
{
throw new UnsupportedOperationException(
"Please set runtime consistency level at Counter level instead of at WideMap level");
}
@Override
public List> findFirst(int count, ConsistencyLevel readLevel)
{
throw new UnsupportedOperationException(
"Please set runtime consistency level at Counter level instead of at WideMap level");
}
@Override
public KeyValue findLast(ConsistencyLevel readLevel)
{
throw new UnsupportedOperationException(
"Please set runtime consistency level at Counter level instead of at WideMap level");
}
@Override
public List> findLast(int count, ConsistencyLevel readLevel)
{
throw new UnsupportedOperationException(
"Please set runtime consistency level at Counter level instead of at WideMap level");
}
@Override
public List findValues(K start, K end, int count, ConsistencyLevel readLevel)
{
throw new UnsupportedOperationException(
"Please set runtime consistency level at Counter level instead of at WideMap level");
}
@Override
public List findValues(K start, K end, int count, BoundingMode bounds,
OrderingMode ordering, ConsistencyLevel readLevel)
{
throw new UnsupportedOperationException(
"Please set runtime consistency level at Counter level instead of at WideMap level");
}
@Override
public List findBoundsExclusiveValues(K start, K end, int count,
ConsistencyLevel readLevel)
{
throw new UnsupportedOperationException(
"Please set runtime consistency level at Counter level instead of at WideMap level");
}
@Override
public List findReverseValues(K start, K end, int count, ConsistencyLevel readLevel)
{
throw new UnsupportedOperationException(
"Please set runtime consistency level at Counter level instead of at WideMap level");
}
@Override
public List findReverseBoundsExclusiveValues(K start, K end, int count,
ConsistencyLevel readLevel)
{
throw new UnsupportedOperationException(
"Please set runtime consistency level at Counter level instead of at WideMap level");
}
@Override
public Counter findFirstValue(ConsistencyLevel readLevel)
{
throw new UnsupportedOperationException(
"Please set runtime consistency level at Counter level instead of at WideMap level");
}
@Override
public List findFirstValues(int count, ConsistencyLevel readLevel)
{
throw new UnsupportedOperationException(
"Please set runtime consistency level at Counter level instead of at WideMap level");
}
@Override
public Counter findLastValue(ConsistencyLevel readLevel)
{
throw new UnsupportedOperationException(
"Please set runtime consistency level at Counter level instead of at WideMap level");
}
@Override
public List findLastValues(int count, ConsistencyLevel readLevel)
{
throw new UnsupportedOperationException(
"Please set runtime consistency level at Counter level instead of at WideMap level");
}
@Override
public List findKeys(K start, K end, int count, BoundingMode bounds, OrderingMode ordering,
ConsistencyLevel readLevel)
{
throw new UnsupportedOperationException(
"Please set runtime consistency level at Counter level instead of at WideMap level");
}
@Override
public List findKeys(K start, K end, int count, ConsistencyLevel readLevel)
{
throw new UnsupportedOperationException(
"Please set runtime consistency level at Counter level instead of at WideMap level");
}
@Override
public List findBoundsExclusiveKeys(K start, K end, int count, ConsistencyLevel readLevel)
{
throw new UnsupportedOperationException(
"Please set runtime consistency level at Counter level instead of at WideMap level");
}
@Override
public List findReverseKeys(K start, K end, int count, ConsistencyLevel readLevel)
{
throw new UnsupportedOperationException(
"Please set runtime consistency level at Counter level instead of at WideMap level");
}
@Override
public List findReverseBoundsExclusiveKeys(K start, K end, int count,
ConsistencyLevel readLevel)
{
throw new UnsupportedOperationException(
"Please set runtime consistency level at Counter level instead of at WideMap level");
}
@Override
public K findFirstKey(ConsistencyLevel readLevel)
{
throw new UnsupportedOperationException(
"Please set runtime consistency level at Counter level instead of at WideMap level");
}
@Override
public List findFirstKeys(int count, ConsistencyLevel readLevel)
{
throw new UnsupportedOperationException(
"Please set runtime consistency level at Counter level instead of at WideMap level");
}
@Override
public K findLastKey(ConsistencyLevel readLevel)
{
throw new UnsupportedOperationException(
"Please set runtime consistency level at Counter level instead of at WideMap level");
}
@Override
public List findLastKeys(int count, ConsistencyLevel readLevel)
{
throw new UnsupportedOperationException(
"Please set runtime consistency level at Counter level instead of at WideMap level");
}
@Override
public KeyValueIterator iterator(ConsistencyLevel readLevel)
{
throw new UnsupportedOperationException(
"Please set runtime consistency level at Counter level instead of at WideMap level");
}
@Override
public KeyValueIterator iterator(K start, K end, int count, BoundingMode bounds,
OrderingMode ordering, ConsistencyLevel readLevel)
{
throw new UnsupportedOperationException(
"Please set runtime consistency level at Counter level instead of at WideMap level");
}
@Override
public KeyValueIterator iterator(int count, ConsistencyLevel readLevel)
{
throw new UnsupportedOperationException(
"Please set runtime consistency level at Counter level instead of at WideMap level");
}
@Override
public KeyValueIterator iterator(K start, K end, int count,
ConsistencyLevel readLevel)
{
throw new UnsupportedOperationException(
"Please set runtime consistency level at Counter level instead of at WideMap level");
}
@Override
public KeyValueIterator iteratorBoundsExclusive(K start, K end, int count,
ConsistencyLevel readLevel)
{
throw new UnsupportedOperationException(
"Please set runtime consistency level at Counter level instead of at WideMap level");
}
@Override
public KeyValueIterator iteratorReverse(ConsistencyLevel readLevel)
{
throw new UnsupportedOperationException(
"Please set runtime consistency level at Counter level instead of at WideMap level");
}
@Override
public KeyValueIterator iteratorReverse(int count, ConsistencyLevel readLevel)
{
throw new UnsupportedOperationException(
"Please set runtime consistency level at Counter level instead of at WideMap level");
}
@Override
public KeyValueIterator iteratorReverse(K start, K end, int count,
ConsistencyLevel readLevel)
{
throw new UnsupportedOperationException(
"Please set runtime consistency level at Counter level instead of at WideMap level");
}
@Override
public KeyValueIterator iteratorReverseBoundsExclusive(K start, K end, int count,
ConsistencyLevel readLevel)
{
throw new UnsupportedOperationException(
"Please set runtime consistency level at Counter level instead of at WideMap level");
}
@Override
public void remove(K key, ConsistencyLevel writeLevel)
{
throw new UnsupportedOperationException("Cannot remove counter value");
}
@Override
public void remove(K start, K end, ConsistencyLevel writeLevel)
{
throw new UnsupportedOperationException("Cannot remove counter value");
}
@Override
public void remove(K start, K end, BoundingMode bounds, ConsistencyLevel writeLevel)
{
throw new UnsupportedOperationException("Cannot remove counter value");
}
@Override
public void removeBoundsExclusive(K start, K end, ConsistencyLevel writeLevel)
{
throw new UnsupportedOperationException("Cannot remove counter value");
}
@Override
public void removeFirst(ConsistencyLevel writeLevel)
{
throw new UnsupportedOperationException("Cannot remove counter value");
}
@Override
public void removeFirst(int count, ConsistencyLevel writeLevel)
{
throw new UnsupportedOperationException("Cannot remove counter value");
}
@Override
public void removeLast(ConsistencyLevel writeLevel)
{
throw new UnsupportedOperationException("Cannot remove counter value");
}
@Override
public void removeLast(int count, ConsistencyLevel writeLevel)
{
throw new UnsupportedOperationException("Cannot remove counter value");
}
public void setId(Object id)
{
this.id = id;
}
public void setPropertyMeta(PropertyMeta propertyMeta)
{
this.propertyMeta = propertyMeta;
}
public void setCompositeHelper(ThriftPropertyHelper thriftPropertyHelper)
{
this.thriftPropertyHelper = thriftPropertyHelper;
}
public void setKeyValueFactory(ThriftKeyValueFactory thriftKeyValueFactory)
{
this.thriftKeyValueFactory = thriftKeyValueFactory;
}
public void setIteratorFactory(ThriftIteratorFactory thriftIteratorFactory)
{
this.thriftIteratorFactory = thriftIteratorFactory;
}
public void setCompositeKeyFactory(ThriftCompositeFactory thriftCompositeFactory)
{
this.thriftCompositeFactory = thriftCompositeFactory;
}
public void setWideMapCounterDao(ThriftGenericWideRowDao wideMapCounterDao)
{
this.wideMapCounterDao = wideMapCounterDao;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy