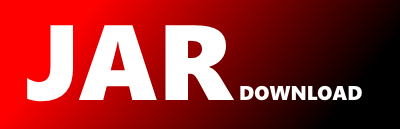
info.archinnov.achilles.composite.ThriftCompositeTransformer Maven / Gradle / Ivy
package info.archinnov.achilles.composite;
import info.archinnov.achilles.compound.ThriftCompoundKeyMapper;
import info.archinnov.achilles.context.ThriftPersistenceContext;
import info.archinnov.achilles.entity.ThriftEntityMapper;
import info.archinnov.achilles.entity.metadata.PropertyMeta;
import info.archinnov.achilles.iterator.ThriftHColumn;
import java.util.List;
import java.util.Map;
import me.prettyprint.hector.api.beans.AbstractComposite.Component;
import me.prettyprint.hector.api.beans.Composite;
import me.prettyprint.hector.api.beans.HColumn;
import me.prettyprint.hector.api.beans.HCounterColumn;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.google.common.base.Function;
/**
* ThriftCompositeTransformer
*
* @author DuyHai DOAN
*
*/
public class ThriftCompositeTransformer
{
private static final Logger log = LoggerFactory.getLogger(ThriftCompositeTransformer.class);
private ThriftCompoundKeyMapper compoundKeyMapper = new ThriftCompoundKeyMapper();
private ThriftEntityMapper mapper = new ThriftEntityMapper();
public Function, ?> buildRawValueTransformer()
{
return new Function, Object>()
{
@Override
public Object apply(HColumn hColumn)
{
return hColumn.getValue();
}
};
}
// //////////////////// Clustered Entities
public Function, T> buildClusteredEntityTransformer(
final Class entityClass,
final ThriftPersistenceContext context)
{
return new Function, T>()
{
@Override
public T apply(HColumn hColumn)
{
return buildClusteredEntity(entityClass, context, hColumn);
}
};
}
public Function, T> buildJoinClusteredEntityTransformer(
final Class entityClass, final ThriftPersistenceContext context,
final Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy