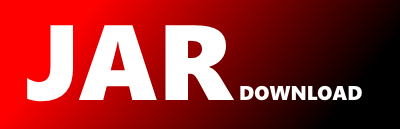
info.archinnov.achilles.context.ThriftConsistencyContext Maven / Gradle / Ivy
package info.archinnov.achilles.context;
import info.archinnov.achilles.consistency.AchillesConsistencyLevelPolicy;
import info.archinnov.achilles.context.execution.SafeExecutionContext;
import info.archinnov.achilles.type.ConsistencyLevel;
import com.google.common.base.Optional;
/**
* ThriftConsistencyContext
*
* @author DuyHai DOAN
*
*/
public class ThriftConsistencyContext implements ConsistencyContext
{
private final AchillesConsistencyLevelPolicy policy;
private Optional readLevelO;
private Optional writeLevelO;
public ThriftConsistencyContext(AchillesConsistencyLevelPolicy policy,
Optional readLevelO, Optional writeLevelO)
{
this.policy = policy;
this.readLevelO = readLevelO;
this.writeLevelO = writeLevelO;
}
public T executeWithReadConsistencyLevel(SafeExecutionContext context)
{
if (readLevelO.isPresent())
{
policy.setCurrentReadLevel(readLevelO.get());
return reinitConsistencyLevels(context);
}
else
{
return context.execute();
}
}
public T executeWithReadConsistencyLevel(SafeExecutionContext context,
ConsistencyLevel readLevel)
{
if (readLevel != null)
{
policy.setCurrentReadLevel(readLevel);
return reinitConsistencyLevels(context);
}
else
{
return context.execute();
}
}
public T executeWithWriteConsistencyLevel(SafeExecutionContext context)
{
if (writeLevelO.isPresent())
{
policy.setCurrentWriteLevel(writeLevelO.get());
return reinitConsistencyLevels(context);
}
else
{
return context.execute();
}
}
public T executeWithWriteConsistencyLevel(SafeExecutionContext context,
ConsistencyLevel writeLevel)
{
if (writeLevel != null)
{
policy.setCurrentWriteLevel(writeLevel);
return reinitConsistencyLevels(context);
}
else
{
return context.execute();
}
}
@Override
public void setReadConsistencyLevel(Optional readLevelO)
{
if (readLevelO.isPresent())
{
this.readLevelO = readLevelO;
policy.setCurrentReadLevel(readLevelO.get());
}
}
public void setReadConsistencyLevel()
{
if (readLevelO.isPresent())
{
policy.setCurrentReadLevel(readLevelO.get());
}
}
@Override
public void setWriteConsistencyLevel(Optional writeLevelO)
{
if (writeLevelO.isPresent())
{
this.writeLevelO = writeLevelO;
policy.setCurrentWriteLevel(writeLevelO.get());
}
}
public void setWriteConsistencyLevel()
{
if (writeLevelO.isPresent())
{
policy.setCurrentWriteLevel(writeLevelO.get());
}
}
@Override
public void reinitConsistencyLevels()
{
policy.reinitCurrentConsistencyLevels();
policy.reinitDefaultConsistencyLevels();
}
public Optional getReadLevelO()
{
return readLevelO;
}
public Optional getWriteLevelO()
{
return writeLevelO;
}
private T reinitConsistencyLevels(SafeExecutionContext context)
{
try
{
return context.execute();
} finally
{
policy.reinitCurrentConsistencyLevels();
policy.reinitDefaultConsistencyLevels();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy