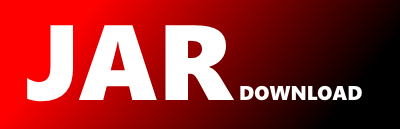
info.archinnov.achilles.iterator.ThriftHColumn Maven / Gradle / Ivy
package info.archinnov.achilles.iterator;
import java.nio.ByteBuffer;
import me.prettyprint.hector.api.Serializer;
import me.prettyprint.hector.api.beans.HColumn;
/**
* ThriftHColumn
*
* @author DuyHai DOAN
*
*/
public class ThriftHColumn implements HColumn
{
private N name;
private V value;
private int ttl;
public ThriftHColumn() {}
public ThriftHColumn(N name, V value) {
this.name = name;
this.value = value;
}
@Override
public HColumn setName(N name)
{
this.name = name;
return this;
}
@Override
public HColumn setValue(V value)
{
this.value = value;
return this;
}
@Override
public N getName()
{
return this.name;
}
@Override
public V getValue()
{
return this.value;
}
@Override
public ByteBuffer getValueBytes()
{
return null;
}
@Override
public ByteBuffer getNameBytes()
{
return null;
}
@Override
public long getClock()
{
return 0;
}
@Override
public HColumn setClock(long clock)
{
return this;
}
@Override
public int getTtl()
{
return ttl;
}
@Override
public HColumn setTtl(int ttl)
{
this.ttl = ttl;
return this;
}
@Override
public HColumn clear()
{
this.name = null;
this.value = null;
this.ttl = 0;
return this;
}
@Override
public HColumn apply(V value, long clock, int ttl)
{
this.value = value;
this.ttl = ttl;
return this;
}
@Override
public Serializer getNameSerializer()
{
return null;
}
@Override
public Serializer getValueSerializer()
{
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy