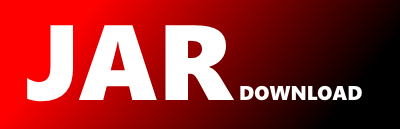
info.archinnov.achilles.generated.dsl.EntityWithNativeCollections_Update Maven / Gradle / Ivy
The newest version!
package info.archinnov.achilles.generated.dsl;
import com.datastax.driver.core.querybuilder.NonEscapingSetAssignment;
import com.datastax.driver.core.querybuilder.NotEq;
import com.datastax.driver.core.querybuilder.QueryBuilder;
import com.datastax.driver.core.querybuilder.Update;
import info.archinnov.achilles.generated.meta.entity.EntityWithNativeCollections_AchillesMeta;
import info.archinnov.achilles.internals.dsl.query.update.AbstractUpdate;
import info.archinnov.achilles.internals.dsl.query.update.AbstractUpdateColumns;
import info.archinnov.achilles.internals.dsl.query.update.AbstractUpdateEnd;
import info.archinnov.achilles.internals.dsl.query.update.AbstractUpdateFrom;
import info.archinnov.achilles.internals.dsl.query.update.AbstractUpdateWhere;
import info.archinnov.achilles.internals.entities.EntityWithNativeCollections;
import info.archinnov.achilles.internals.metamodel.AbstractEntityProperty;
import info.archinnov.achilles.internals.options.CassandraOptions;
import info.archinnov.achilles.internals.runtime.RuntimeEngine;
import info.archinnov.achilles.type.SchemaNameProvider;
import info.archinnov.achilles.type.tuples.Tuple2;
import info.archinnov.achilles.validation.Validator;
import java.lang.Class;
import java.lang.Double;
import java.lang.Integer;
import java.lang.Long;
import java.lang.Object;
import java.lang.Override;
import java.lang.SuppressWarnings;
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.stream.Collectors;
import org.apache.commons.lang3.ArrayUtils;
public final class EntityWithNativeCollections_Update extends AbstractUpdate {
protected final EntityWithNativeCollections_AchillesMeta meta;
protected final Class entityClass = EntityWithNativeCollections.class;
public EntityWithNativeCollections_Update(RuntimeEngine rte, EntityWithNativeCollections_AchillesMeta meta) {
super(rte);
this.meta = meta;
}
/**
* Generate an UPDATE FROM ... */
public final EntityWithNativeCollections_Update.F fromBaseTable() {
final String currentKeyspace = meta.getKeyspace().orElse("unknown_keyspace_for_" + meta.entityClass.getCanonicalName());
final Update.Where where = QueryBuilder.update(currentKeyspace, meta.getTableOrViewName()).where();
return new EntityWithNativeCollections_Update.F(where, new CassandraOptions());
}
/**
* Generate an UPDATE FROM ... using the given SchemaNameProvider */
public final EntityWithNativeCollections_Update.F from(final SchemaNameProvider schemaNameProvider) {
final String currentKeyspace = lookupKeyspace(schemaNameProvider, meta.entityClass);
final String currentTable = lookupTable(schemaNameProvider, meta.entityClass);
final Update.Where where = QueryBuilder.update(currentKeyspace, currentTable).where();
return new EntityWithNativeCollections_Update.F(where, CassandraOptions.withSchemaNameProvider(schemaNameProvider));
}
public class Cols extends AbstractUpdateColumns {
Cols(Update.Where where, CassandraOptions cassandraOptions) {
super(where, cassandraOptions);
}
public final Cols.LongList longList() {
return new Cols.LongList();
}
public final Cols.DoubleList doubleList() {
return new Cols.DoubleList();
}
public final Cols.MapIntLong mapIntLong() {
return new Cols.MapIntLong();
}
public final Cols.Tuple2 tuple2() {
return new Cols.Tuple2();
}
public final EntityWithNativeCollections_Update.W_Id where() {
return new EntityWithNativeCollections_Update.W_Id(where, cassandraOptions);
}
public final class LongList {
/**
* Generate an UPDATE FROM ... SET longlist = longlist + [?] */
@SuppressWarnings("static-access")
public final EntityWithNativeCollections_Update.Cols AppendTo(final Long longList_element) {
where.with(QueryBuilder.appendAll("longlist", QueryBuilder.bindMarker("longlist")));
boundValues.add(Arrays.asList(longList_element));
encodedValues.add(meta.longList.encodeFromJava(Arrays.asList(longList_element), Optional.of(cassandraOptions)));
return EntityWithNativeCollections_Update.Cols.this;
}
/**
* Generate an UPDATE FROM ... SET longList = longList + ? */
@SuppressWarnings("static-access")
public final EntityWithNativeCollections_Update.Cols AppendAllTo(final List longList_element) {
where.with(QueryBuilder.appendAll("longlist", QueryBuilder.bindMarker("longlist")));
boundValues.add(longList_element);
encodedValues.add(meta.longList.encodeFromJava(longList_element, Optional.of(cassandraOptions)));
return EntityWithNativeCollections_Update.Cols.this;
}
/**
* Generate an UPDATE FROM ... SET longList = [?] + longList */
@SuppressWarnings("static-access")
public final EntityWithNativeCollections_Update.Cols PrependTo(final Long longList_element) {
where.with(QueryBuilder.prependAll("longlist", QueryBuilder.bindMarker("longlist")));
boundValues.add(Arrays.asList(longList_element));
encodedValues.add(meta.longList.encodeFromJava(Arrays.asList(longList_element), Optional.of(cassandraOptions)));
return EntityWithNativeCollections_Update.Cols.this;
}
/**
* Generate an UPDATE FROM ... SET longList = ? + longList */
@SuppressWarnings("static-access")
public final EntityWithNativeCollections_Update.Cols PrependAllTo(final List longList_element) {
where.with(QueryBuilder.prependAll("longlist", QueryBuilder.bindMarker("longlist")));
boundValues.add(longList_element);
encodedValues.add(meta.longList.encodeFromJava(longList_element, Optional.of(cassandraOptions)));
return EntityWithNativeCollections_Update.Cols.this;
}
/**
* Generate an UPDATE FROM ... SET longList[index] = ? */
public final EntityWithNativeCollections_Update.Cols SetAtIndex(final int index, final Long longList_element) {
where.with(QueryBuilder.setIdx("longlist", index, QueryBuilder.bindMarker("longlist")));
boundValues.add(longList_element);
encodedValues.add(meta.longList.valueProperty.encodeFromJava(longList_element, Optional.of(cassandraOptions)));
return EntityWithNativeCollections_Update.Cols.this;
}
/**
* Generate an UPDATE FROM ... SET longList[index] = null */
public final EntityWithNativeCollections_Update.Cols RemoveAtIndex(final int index) {
where.with(QueryBuilder.setIdx("longlist", index, QueryBuilder.bindMarker("longlist")));
boundValues.add(null);
encodedValues.add(null);
return EntityWithNativeCollections_Update.Cols.this;
}
/**
* Generate an UPDATE FROM ... SET longList = longList - [?] */
@SuppressWarnings("static-access")
public final EntityWithNativeCollections_Update.Cols RemoveFrom(final Long longList_element) {
where.with(QueryBuilder.discardAll("longlist", QueryBuilder.bindMarker("longlist")));
boundValues.add(Arrays.asList(longList_element));
encodedValues.add(meta.longList.encodeFromJava(Arrays.asList(longList_element), Optional.of(cassandraOptions)));
return EntityWithNativeCollections_Update.Cols.this;
}
/**
* Generate an UPDATE FROM ... SET longList = longList - ? */
@SuppressWarnings("static-access")
public final EntityWithNativeCollections_Update.Cols RemoveAllFrom(final List longList_element) {
where.with(QueryBuilder.discardAll("longlist", QueryBuilder.bindMarker("longlist")));
boundValues.add(longList_element);
encodedValues.add(meta.longList.encodeFromJava(longList_element, Optional.of(cassandraOptions)));
return EntityWithNativeCollections_Update.Cols.this;
}
/**
* Generate an UPDATE FROM ... SET longList = ? */
@SuppressWarnings("static-access")
public final EntityWithNativeCollections_Update.Cols Set(final List longList_element) {
where.with(NonEscapingSetAssignment.of("longlist", QueryBuilder.bindMarker("longlist")));
boundValues.add(longList_element);
encodedValues.add(meta.longList.encodeFromJava(longList_element, Optional.of(cassandraOptions)));
return EntityWithNativeCollections_Update.Cols.this;
}
}
public final class DoubleList {
/**
* Generate an UPDATE FROM ... SET doublelist = doublelist + [?] */
@SuppressWarnings("static-access")
public final EntityWithNativeCollections_Update.Cols AppendTo(final Double doubleList_element) {
where.with(QueryBuilder.appendAll("doublelist", QueryBuilder.bindMarker("doublelist")));
boundValues.add(Arrays.asList(doubleList_element));
encodedValues.add(meta.doubleList.encodeFromJava(Arrays.asList(doubleList_element), Optional.of(cassandraOptions)));
return EntityWithNativeCollections_Update.Cols.this;
}
/**
* Generate an UPDATE FROM ... SET doubleList = doubleList + ? */
@SuppressWarnings("static-access")
public final EntityWithNativeCollections_Update.Cols AppendAllTo(final List doubleList_element) {
where.with(QueryBuilder.appendAll("doublelist", QueryBuilder.bindMarker("doublelist")));
boundValues.add(doubleList_element);
encodedValues.add(meta.doubleList.encodeFromJava(doubleList_element, Optional.of(cassandraOptions)));
return EntityWithNativeCollections_Update.Cols.this;
}
/**
* Generate an UPDATE FROM ... SET doubleList = [?] + doubleList */
@SuppressWarnings("static-access")
public final EntityWithNativeCollections_Update.Cols PrependTo(final Double doubleList_element) {
where.with(QueryBuilder.prependAll("doublelist", QueryBuilder.bindMarker("doublelist")));
boundValues.add(Arrays.asList(doubleList_element));
encodedValues.add(meta.doubleList.encodeFromJava(Arrays.asList(doubleList_element), Optional.of(cassandraOptions)));
return EntityWithNativeCollections_Update.Cols.this;
}
/**
* Generate an UPDATE FROM ... SET doubleList = ? + doubleList */
@SuppressWarnings("static-access")
public final EntityWithNativeCollections_Update.Cols PrependAllTo(final List doubleList_element) {
where.with(QueryBuilder.prependAll("doublelist", QueryBuilder.bindMarker("doublelist")));
boundValues.add(doubleList_element);
encodedValues.add(meta.doubleList.encodeFromJava(doubleList_element, Optional.of(cassandraOptions)));
return EntityWithNativeCollections_Update.Cols.this;
}
/**
* Generate an UPDATE FROM ... SET doubleList[index] = ? */
public final EntityWithNativeCollections_Update.Cols SetAtIndex(final int index, final Double doubleList_element) {
where.with(QueryBuilder.setIdx("doublelist", index, QueryBuilder.bindMarker("doublelist")));
boundValues.add(doubleList_element);
encodedValues.add(meta.doubleList.valueProperty.encodeFromJava(doubleList_element, Optional.of(cassandraOptions)));
return EntityWithNativeCollections_Update.Cols.this;
}
/**
* Generate an UPDATE FROM ... SET doubleList[index] = null */
public final EntityWithNativeCollections_Update.Cols RemoveAtIndex(final int index) {
where.with(QueryBuilder.setIdx("doublelist", index, QueryBuilder.bindMarker("doublelist")));
boundValues.add(null);
encodedValues.add(null);
return EntityWithNativeCollections_Update.Cols.this;
}
/**
* Generate an UPDATE FROM ... SET doubleList = doubleList - [?] */
@SuppressWarnings("static-access")
public final EntityWithNativeCollections_Update.Cols RemoveFrom(final Double doubleList_element) {
where.with(QueryBuilder.discardAll("doublelist", QueryBuilder.bindMarker("doublelist")));
boundValues.add(Arrays.asList(doubleList_element));
encodedValues.add(meta.doubleList.encodeFromJava(Arrays.asList(doubleList_element), Optional.of(cassandraOptions)));
return EntityWithNativeCollections_Update.Cols.this;
}
/**
* Generate an UPDATE FROM ... SET doubleList = doubleList - ? */
@SuppressWarnings("static-access")
public final EntityWithNativeCollections_Update.Cols RemoveAllFrom(final List doubleList_element) {
where.with(QueryBuilder.discardAll("doublelist", QueryBuilder.bindMarker("doublelist")));
boundValues.add(doubleList_element);
encodedValues.add(meta.doubleList.encodeFromJava(doubleList_element, Optional.of(cassandraOptions)));
return EntityWithNativeCollections_Update.Cols.this;
}
/**
* Generate an UPDATE FROM ... SET doubleList = ? */
@SuppressWarnings("static-access")
public final EntityWithNativeCollections_Update.Cols Set(final List doubleList_element) {
where.with(NonEscapingSetAssignment.of("doublelist", QueryBuilder.bindMarker("doublelist")));
boundValues.add(doubleList_element);
encodedValues.add(meta.doubleList.encodeFromJava(doubleList_element, Optional.of(cassandraOptions)));
return EntityWithNativeCollections_Update.Cols.this;
}
}
public final class MapIntLong {
/**
* Generate an UPDATE FROM ... SET mapintlong[?] = ? */
public final EntityWithNativeCollections_Update.Cols PutTo(final Integer mapIntLong_key, final Long mapIntLong_value) {
where.with(QueryBuilder.put("mapintlong", QueryBuilder.bindMarker("mapIntLong_key"), QueryBuilder.bindMarker("mapIntLong_value")));
boundValues.add(mapIntLong_key);
boundValues.add(mapIntLong_value);
encodedValues.add(meta.mapIntLong.keyProperty.encodeFromJava(mapIntLong_key, Optional.of(cassandraOptions)));
encodedValues.add(meta.mapIntLong.valueProperty.encodeFromJava(mapIntLong_value, Optional.of(cassandraOptions)));
return EntityWithNativeCollections_Update.Cols.this;
}
/**
* Generate an UPDATE FROM ... SET mapIntLong = mapIntLong + ? */
@SuppressWarnings("static-access")
public final EntityWithNativeCollections_Update.Cols AddAllTo(final Map mapIntLong) {
where.with(QueryBuilder.addAll("mapintlong", QueryBuilder.bindMarker("mapintlong")));
boundValues.add(mapIntLong);
encodedValues.add(meta.mapIntLong.encodeFromJava(mapIntLong, Optional.of(cassandraOptions)));
return EntityWithNativeCollections_Update.Cols.this;
}
/**
* Generate an UPDATE FROM ... SET mapIntLong[?] = null */
@SuppressWarnings("static-access")
public final EntityWithNativeCollections_Update.Cols RemoveByKey(final Integer mapIntLong_key) {
where.with(QueryBuilder.put("mapintlong", QueryBuilder.bindMarker("mapIntLong_key"), QueryBuilder.bindMarker("mapIntLong_value")));
boundValues.add(mapIntLong_key);
boundValues.add(null);
encodedValues.add(meta.mapIntLong.keyProperty.encodeFromJava(mapIntLong_key, Optional.of(cassandraOptions)));
encodedValues.add(null);
return EntityWithNativeCollections_Update.Cols.this;
}
/**
* Generate an UPDATE FROM ... SET mapIntLong = ? */
@SuppressWarnings("static-access")
public final EntityWithNativeCollections_Update.Cols Set(final Map mapIntLong) {
where.with(NonEscapingSetAssignment.of("mapintlong", QueryBuilder.bindMarker("mapintlong")));
boundValues.add(mapIntLong);
encodedValues.add(meta.mapIntLong.encodeFromJava(mapIntLong, Optional.of(cassandraOptions)));
return EntityWithNativeCollections_Update.Cols.this;
}
}
public final class Tuple2 {
/**
* Generate an UPDATE FROM ... SET tuple2 = ? */
@SuppressWarnings("static-access")
public final EntityWithNativeCollections_Update.Cols Set(final info.archinnov.achilles.type.tuples.Tuple2, List> tuple2) {
where.with(NonEscapingSetAssignment.of("tuple2", QueryBuilder.bindMarker("tuple2")));
boundValues.add(tuple2);
encodedValues.add(meta.tuple2.encodeFromJava(tuple2, Optional.of(cassandraOptions)));
return EntityWithNativeCollections_Update.Cols.this;
}
}
}
public class F extends AbstractUpdateFrom {
F(Update.Where where, CassandraOptions cassandraOptions) {
super(where, cassandraOptions);
}
public final F.LongList longList() {
return new F.LongList();
}
public final F.DoubleList doubleList() {
return new F.DoubleList();
}
public final F.MapIntLong mapIntLong() {
return new F.MapIntLong();
}
public final F.Tuple2 tuple2() {
return new F.Tuple2();
}
public final class LongList {
/**
* Generate an UPDATE FROM ... SET longlist = longlist + [?] */
@SuppressWarnings("static-access")
public final EntityWithNativeCollections_Update.Cols AppendTo(final Long longList_element) {
where.with(QueryBuilder.appendAll("longlist", QueryBuilder.bindMarker("longlist")));
boundValues.add(Arrays.asList(longList_element));
encodedValues.add(meta.longList.encodeFromJava(Arrays.asList(longList_element), Optional.of(cassandraOptions)));
return new EntityWithNativeCollections_Update.Cols(where, cassandraOptions);
}
/**
* Generate an UPDATE FROM ... SET longList = longList + ? */
@SuppressWarnings("static-access")
public final EntityWithNativeCollections_Update.Cols AppendAllTo(final List longList_element) {
where.with(QueryBuilder.appendAll("longlist", QueryBuilder.bindMarker("longlist")));
boundValues.add(longList_element);
encodedValues.add(meta.longList.encodeFromJava(longList_element, Optional.of(cassandraOptions)));
return new EntityWithNativeCollections_Update.Cols(where, cassandraOptions);
}
/**
* Generate an UPDATE FROM ... SET longList = [?] + longList */
@SuppressWarnings("static-access")
public final EntityWithNativeCollections_Update.Cols PrependTo(final Long longList_element) {
where.with(QueryBuilder.prependAll("longlist", QueryBuilder.bindMarker("longlist")));
boundValues.add(Arrays.asList(longList_element));
encodedValues.add(meta.longList.encodeFromJava(Arrays.asList(longList_element), Optional.of(cassandraOptions)));
return new EntityWithNativeCollections_Update.Cols(where, cassandraOptions);
}
/**
* Generate an UPDATE FROM ... SET longList = ? + longList */
@SuppressWarnings("static-access")
public final EntityWithNativeCollections_Update.Cols PrependAllTo(final List longList_element) {
where.with(QueryBuilder.prependAll("longlist", QueryBuilder.bindMarker("longlist")));
boundValues.add(longList_element);
encodedValues.add(meta.longList.encodeFromJava(longList_element, Optional.of(cassandraOptions)));
return new EntityWithNativeCollections_Update.Cols(where, cassandraOptions);
}
/**
* Generate an UPDATE FROM ... SET longList[index] = ? */
public final EntityWithNativeCollections_Update.Cols SetAtIndex(final int index, final Long longList_element) {
where.with(QueryBuilder.setIdx("longlist", index, QueryBuilder.bindMarker("longlist")));
boundValues.add(longList_element);
encodedValues.add(meta.longList.valueProperty.encodeFromJava(longList_element, Optional.of(cassandraOptions)));
return new EntityWithNativeCollections_Update.Cols(where, cassandraOptions);
}
/**
* Generate an UPDATE FROM ... SET longList[index] = null */
public final EntityWithNativeCollections_Update.Cols RemoveAtIndex(final int index) {
where.with(QueryBuilder.setIdx("longlist", index, QueryBuilder.bindMarker("longlist")));
boundValues.add(null);
encodedValues.add(null);
return new EntityWithNativeCollections_Update.Cols(where, cassandraOptions);
}
/**
* Generate an UPDATE FROM ... SET longList = longList - [?] */
@SuppressWarnings("static-access")
public final EntityWithNativeCollections_Update.Cols RemoveFrom(final Long longList_element) {
where.with(QueryBuilder.discardAll("longlist", QueryBuilder.bindMarker("longlist")));
boundValues.add(Arrays.asList(longList_element));
encodedValues.add(meta.longList.encodeFromJava(Arrays.asList(longList_element), Optional.of(cassandraOptions)));
return new EntityWithNativeCollections_Update.Cols(where, cassandraOptions);
}
/**
* Generate an UPDATE FROM ... SET longList = longList - ? */
@SuppressWarnings("static-access")
public final EntityWithNativeCollections_Update.Cols RemoveAllFrom(final List longList_element) {
where.with(QueryBuilder.discardAll("longlist", QueryBuilder.bindMarker("longlist")));
boundValues.add(longList_element);
encodedValues.add(meta.longList.encodeFromJava(longList_element, Optional.of(cassandraOptions)));
return new EntityWithNativeCollections_Update.Cols(where, cassandraOptions);
}
/**
* Generate an UPDATE FROM ... SET longList = ? */
@SuppressWarnings("static-access")
public final EntityWithNativeCollections_Update.Cols Set(final List longList_element) {
where.with(NonEscapingSetAssignment.of("longlist", QueryBuilder.bindMarker("longlist")));
boundValues.add(longList_element);
encodedValues.add(meta.longList.encodeFromJava(longList_element, Optional.of(cassandraOptions)));
return new EntityWithNativeCollections_Update.Cols(where, cassandraOptions);
}
}
public final class DoubleList {
/**
* Generate an UPDATE FROM ... SET doublelist = doublelist + [?] */
@SuppressWarnings("static-access")
public final EntityWithNativeCollections_Update.Cols AppendTo(final Double doubleList_element) {
where.with(QueryBuilder.appendAll("doublelist", QueryBuilder.bindMarker("doublelist")));
boundValues.add(Arrays.asList(doubleList_element));
encodedValues.add(meta.doubleList.encodeFromJava(Arrays.asList(doubleList_element), Optional.of(cassandraOptions)));
return new EntityWithNativeCollections_Update.Cols(where, cassandraOptions);
}
/**
* Generate an UPDATE FROM ... SET doubleList = doubleList + ? */
@SuppressWarnings("static-access")
public final EntityWithNativeCollections_Update.Cols AppendAllTo(final List doubleList_element) {
where.with(QueryBuilder.appendAll("doublelist", QueryBuilder.bindMarker("doublelist")));
boundValues.add(doubleList_element);
encodedValues.add(meta.doubleList.encodeFromJava(doubleList_element, Optional.of(cassandraOptions)));
return new EntityWithNativeCollections_Update.Cols(where, cassandraOptions);
}
/**
* Generate an UPDATE FROM ... SET doubleList = [?] + doubleList */
@SuppressWarnings("static-access")
public final EntityWithNativeCollections_Update.Cols PrependTo(final Double doubleList_element) {
where.with(QueryBuilder.prependAll("doublelist", QueryBuilder.bindMarker("doublelist")));
boundValues.add(Arrays.asList(doubleList_element));
encodedValues.add(meta.doubleList.encodeFromJava(Arrays.asList(doubleList_element), Optional.of(cassandraOptions)));
return new EntityWithNativeCollections_Update.Cols(where, cassandraOptions);
}
/**
* Generate an UPDATE FROM ... SET doubleList = ? + doubleList */
@SuppressWarnings("static-access")
public final EntityWithNativeCollections_Update.Cols PrependAllTo(final List doubleList_element) {
where.with(QueryBuilder.prependAll("doublelist", QueryBuilder.bindMarker("doublelist")));
boundValues.add(doubleList_element);
encodedValues.add(meta.doubleList.encodeFromJava(doubleList_element, Optional.of(cassandraOptions)));
return new EntityWithNativeCollections_Update.Cols(where, cassandraOptions);
}
/**
* Generate an UPDATE FROM ... SET doubleList[index] = ? */
public final EntityWithNativeCollections_Update.Cols SetAtIndex(final int index, final Double doubleList_element) {
where.with(QueryBuilder.setIdx("doublelist", index, QueryBuilder.bindMarker("doublelist")));
boundValues.add(doubleList_element);
encodedValues.add(meta.doubleList.valueProperty.encodeFromJava(doubleList_element, Optional.of(cassandraOptions)));
return new EntityWithNativeCollections_Update.Cols(where, cassandraOptions);
}
/**
* Generate an UPDATE FROM ... SET doubleList[index] = null */
public final EntityWithNativeCollections_Update.Cols RemoveAtIndex(final int index) {
where.with(QueryBuilder.setIdx("doublelist", index, QueryBuilder.bindMarker("doublelist")));
boundValues.add(null);
encodedValues.add(null);
return new EntityWithNativeCollections_Update.Cols(where, cassandraOptions);
}
/**
* Generate an UPDATE FROM ... SET doubleList = doubleList - [?] */
@SuppressWarnings("static-access")
public final EntityWithNativeCollections_Update.Cols RemoveFrom(final Double doubleList_element) {
where.with(QueryBuilder.discardAll("doublelist", QueryBuilder.bindMarker("doublelist")));
boundValues.add(Arrays.asList(doubleList_element));
encodedValues.add(meta.doubleList.encodeFromJava(Arrays.asList(doubleList_element), Optional.of(cassandraOptions)));
return new EntityWithNativeCollections_Update.Cols(where, cassandraOptions);
}
/**
* Generate an UPDATE FROM ... SET doubleList = doubleList - ? */
@SuppressWarnings("static-access")
public final EntityWithNativeCollections_Update.Cols RemoveAllFrom(final List doubleList_element) {
where.with(QueryBuilder.discardAll("doublelist", QueryBuilder.bindMarker("doublelist")));
boundValues.add(doubleList_element);
encodedValues.add(meta.doubleList.encodeFromJava(doubleList_element, Optional.of(cassandraOptions)));
return new EntityWithNativeCollections_Update.Cols(where, cassandraOptions);
}
/**
* Generate an UPDATE FROM ... SET doubleList = ? */
@SuppressWarnings("static-access")
public final EntityWithNativeCollections_Update.Cols Set(final List doubleList_element) {
where.with(NonEscapingSetAssignment.of("doublelist", QueryBuilder.bindMarker("doublelist")));
boundValues.add(doubleList_element);
encodedValues.add(meta.doubleList.encodeFromJava(doubleList_element, Optional.of(cassandraOptions)));
return new EntityWithNativeCollections_Update.Cols(where, cassandraOptions);
}
}
public final class MapIntLong {
/**
* Generate an UPDATE FROM ... SET mapintlong[?] = ? */
public final EntityWithNativeCollections_Update.Cols PutTo(final Integer mapIntLong_key, final Long mapIntLong_value) {
where.with(QueryBuilder.put("mapintlong", QueryBuilder.bindMarker("mapIntLong_key"), QueryBuilder.bindMarker("mapIntLong_value")));
boundValues.add(mapIntLong_key);
boundValues.add(mapIntLong_value);
encodedValues.add(meta.mapIntLong.keyProperty.encodeFromJava(mapIntLong_key, Optional.of(cassandraOptions)));
encodedValues.add(meta.mapIntLong.valueProperty.encodeFromJava(mapIntLong_value, Optional.of(cassandraOptions)));
return new EntityWithNativeCollections_Update.Cols(where, cassandraOptions);
}
/**
* Generate an UPDATE FROM ... SET mapIntLong = mapIntLong + ? */
@SuppressWarnings("static-access")
public final EntityWithNativeCollections_Update.Cols AddAllTo(final Map mapIntLong) {
where.with(QueryBuilder.addAll("mapintlong", QueryBuilder.bindMarker("mapintlong")));
boundValues.add(mapIntLong);
encodedValues.add(meta.mapIntLong.encodeFromJava(mapIntLong, Optional.of(cassandraOptions)));
return new EntityWithNativeCollections_Update.Cols(where, cassandraOptions);
}
/**
* Generate an UPDATE FROM ... SET mapIntLong[?] = null */
@SuppressWarnings("static-access")
public final EntityWithNativeCollections_Update.Cols RemoveByKey(final Integer mapIntLong_key) {
where.with(QueryBuilder.put("mapintlong", QueryBuilder.bindMarker("mapIntLong_key"), QueryBuilder.bindMarker("mapIntLong_value")));
boundValues.add(mapIntLong_key);
boundValues.add(null);
encodedValues.add(meta.mapIntLong.keyProperty.encodeFromJava(mapIntLong_key, Optional.of(cassandraOptions)));
encodedValues.add(null);
return new EntityWithNativeCollections_Update.Cols(where, cassandraOptions);
}
/**
* Generate an UPDATE FROM ... SET mapIntLong = ? */
@SuppressWarnings("static-access")
public final EntityWithNativeCollections_Update.Cols Set(final Map mapIntLong) {
where.with(NonEscapingSetAssignment.of("mapintlong", QueryBuilder.bindMarker("mapintlong")));
boundValues.add(mapIntLong);
encodedValues.add(meta.mapIntLong.encodeFromJava(mapIntLong, Optional.of(cassandraOptions)));
return new EntityWithNativeCollections_Update.Cols(where, cassandraOptions);
}
}
public final class Tuple2 {
/**
* Generate an UPDATE FROM ... SET tuple2 = ? */
@SuppressWarnings("static-access")
public final EntityWithNativeCollections_Update.Cols Set(final info.archinnov.achilles.type.tuples.Tuple2, List> tuple2) {
where.with(NonEscapingSetAssignment.of("tuple2", QueryBuilder.bindMarker("tuple2")));
boundValues.add(tuple2);
encodedValues.add(meta.tuple2.encodeFromJava(tuple2, Optional.of(cassandraOptions)));
return new EntityWithNativeCollections_Update.Cols(where, cassandraOptions);
}
}
}
public final class W_Id extends AbstractUpdateWhere {
public W_Id(Update.Where where, CassandraOptions cassandraOptions) {
super(where, cassandraOptions);
}
public final EntityWithNativeCollections_Update.W_Id.Relation id() {
return new EntityWithNativeCollections_Update.W_Id.Relation();
}
public final class Relation {
/**
* Generate a SELECT ... FROM ... WHERE ... id = ? */
@SuppressWarnings("static-access")
public final EntityWithNativeCollections_Update.E Eq(Long id) {
where.and(QueryBuilder.eq("id", QueryBuilder.bindMarker("id")));
boundValues.add(id);
encodedValues.add(meta.id.encodeFromJava(id, Optional.of(cassandraOptions)));
return new EntityWithNativeCollections_Update.E(where, cassandraOptions);
}
/**
* Generate a SELECT ... FROM ... WHERE ... id IN ? */
@SuppressWarnings("static-access")
public final EntityWithNativeCollections_Update.E IN(Long... id) {
Validator.validateTrue(ArrayUtils.isNotEmpty(id), "Varargs for field '%s' should not be null/empty", "id");
where.and(QueryBuilder.in("id",QueryBuilder.bindMarker("id")));
final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy