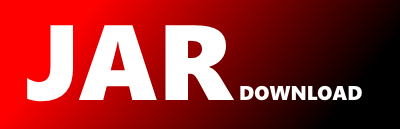
info.archinnov.achilles.generated.dsl.EntityWithUDTForDynamicKeyspace_Update Maven / Gradle / Ivy
The newest version!
package info.archinnov.achilles.generated.dsl;
import com.datastax.driver.core.querybuilder.NonEscapingSetAssignment;
import com.datastax.driver.core.querybuilder.NotEq;
import com.datastax.driver.core.querybuilder.QueryBuilder;
import com.datastax.driver.core.querybuilder.Update;
import com.google.common.collect.Sets;
import info.archinnov.achilles.generated.meta.entity.EntityWithUDTForDynamicKeyspace_AchillesMeta;
import info.archinnov.achilles.internals.dsl.query.update.AbstractUpdate;
import info.archinnov.achilles.internals.dsl.query.update.AbstractUpdateColumns;
import info.archinnov.achilles.internals.dsl.query.update.AbstractUpdateEnd;
import info.archinnov.achilles.internals.dsl.query.update.AbstractUpdateFrom;
import info.archinnov.achilles.internals.dsl.query.update.AbstractUpdateWhere;
import info.archinnov.achilles.internals.entities.EntityWithUDTForDynamicKeyspace;
import info.archinnov.achilles.internals.entities.UDTWithNoKeyspace;
import info.archinnov.achilles.internals.metamodel.AbstractEntityProperty;
import info.archinnov.achilles.internals.options.CassandraOptions;
import info.archinnov.achilles.internals.runtime.RuntimeEngine;
import info.archinnov.achilles.type.SchemaNameProvider;
import info.archinnov.achilles.validation.Validator;
import java.lang.Class;
import java.lang.Integer;
import java.lang.Long;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.SuppressWarnings;
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.Set;
import java.util.stream.Collectors;
import org.apache.commons.lang3.ArrayUtils;
public final class EntityWithUDTForDynamicKeyspace_Update extends AbstractUpdate {
protected final EntityWithUDTForDynamicKeyspace_AchillesMeta meta;
protected final Class entityClass = EntityWithUDTForDynamicKeyspace.class;
public EntityWithUDTForDynamicKeyspace_Update(RuntimeEngine rte, EntityWithUDTForDynamicKeyspace_AchillesMeta meta) {
super(rte);
this.meta = meta;
}
/**
* Generate an UPDATE FROM ... */
public final EntityWithUDTForDynamicKeyspace_Update.F fromBaseTable() {
final String currentKeyspace = meta.getKeyspace().orElse("unknown_keyspace_for_" + meta.entityClass.getCanonicalName());
final Update.Where where = QueryBuilder.update(currentKeyspace, meta.getTableOrViewName()).where();
return new EntityWithUDTForDynamicKeyspace_Update.F(where, new CassandraOptions());
}
/**
* Generate an UPDATE FROM ... using the given SchemaNameProvider */
public final EntityWithUDTForDynamicKeyspace_Update.F from(final SchemaNameProvider schemaNameProvider) {
final String currentKeyspace = lookupKeyspace(schemaNameProvider, meta.entityClass);
final String currentTable = lookupTable(schemaNameProvider, meta.entityClass);
final Update.Where where = QueryBuilder.update(currentKeyspace, currentTable).where();
return new EntityWithUDTForDynamicKeyspace_Update.F(where, CassandraOptions.withSchemaNameProvider(schemaNameProvider));
}
public class Cols extends AbstractUpdateColumns {
Cols(Update.Where where, CassandraOptions cassandraOptions) {
super(where, cassandraOptions);
}
public final Cols.Udt udt() {
return new Cols.Udt();
}
public final Cols.UdtList udtList() {
return new Cols.UdtList();
}
public final Cols.UdtSet udtSet() {
return new Cols.UdtSet();
}
public final Cols.UdtMapKey udtMapKey() {
return new Cols.UdtMapKey();
}
public final Cols.UdtMapValue udtMapValue() {
return new Cols.UdtMapValue();
}
public final EntityWithUDTForDynamicKeyspace_Update.W_Id where() {
return new EntityWithUDTForDynamicKeyspace_Update.W_Id(where, cassandraOptions);
}
public final class Udt {
/**
* Generate an UPDATE FROM ... SET udt = ? */
@SuppressWarnings("static-access")
public final EntityWithUDTForDynamicKeyspace_Update.Cols Set(final UDTWithNoKeyspace udt) {
where.with(NonEscapingSetAssignment.of("udt", QueryBuilder.bindMarker("udt")));
boundValues.add(udt);
encodedValues.add(meta.udt.encodeFromJava(udt, Optional.of(cassandraOptions)));
return EntityWithUDTForDynamicKeyspace_Update.Cols.this;
}
}
public final class UdtList {
/**
* Generate an UPDATE FROM ... SET udtlist = udtlist + [?] */
@SuppressWarnings("static-access")
public final EntityWithUDTForDynamicKeyspace_Update.Cols AppendTo(final UDTWithNoKeyspace udtList_element) {
where.with(QueryBuilder.appendAll("udtlist", QueryBuilder.bindMarker("udtlist")));
boundValues.add(Arrays.asList(udtList_element));
encodedValues.add(meta.udtList.encodeFromJava(Arrays.asList(udtList_element), Optional.of(cassandraOptions)));
return EntityWithUDTForDynamicKeyspace_Update.Cols.this;
}
/**
* Generate an UPDATE FROM ... SET udtList = udtList + ? */
@SuppressWarnings("static-access")
public final EntityWithUDTForDynamicKeyspace_Update.Cols AppendAllTo(final List udtList_element) {
where.with(QueryBuilder.appendAll("udtlist", QueryBuilder.bindMarker("udtlist")));
boundValues.add(udtList_element);
encodedValues.add(meta.udtList.encodeFromJava(udtList_element, Optional.of(cassandraOptions)));
return EntityWithUDTForDynamicKeyspace_Update.Cols.this;
}
/**
* Generate an UPDATE FROM ... SET udtList = [?] + udtList */
@SuppressWarnings("static-access")
public final EntityWithUDTForDynamicKeyspace_Update.Cols PrependTo(final UDTWithNoKeyspace udtList_element) {
where.with(QueryBuilder.prependAll("udtlist", QueryBuilder.bindMarker("udtlist")));
boundValues.add(Arrays.asList(udtList_element));
encodedValues.add(meta.udtList.encodeFromJava(Arrays.asList(udtList_element), Optional.of(cassandraOptions)));
return EntityWithUDTForDynamicKeyspace_Update.Cols.this;
}
/**
* Generate an UPDATE FROM ... SET udtList = ? + udtList */
@SuppressWarnings("static-access")
public final EntityWithUDTForDynamicKeyspace_Update.Cols PrependAllTo(final List udtList_element) {
where.with(QueryBuilder.prependAll("udtlist", QueryBuilder.bindMarker("udtlist")));
boundValues.add(udtList_element);
encodedValues.add(meta.udtList.encodeFromJava(udtList_element, Optional.of(cassandraOptions)));
return EntityWithUDTForDynamicKeyspace_Update.Cols.this;
}
/**
* Generate an UPDATE FROM ... SET udtList[index] = ? */
public final EntityWithUDTForDynamicKeyspace_Update.Cols SetAtIndex(final int index, final UDTWithNoKeyspace udtList_element) {
where.with(QueryBuilder.setIdx("udtlist", index, QueryBuilder.bindMarker("udtlist")));
boundValues.add(udtList_element);
encodedValues.add(meta.udtList.valueProperty.encodeFromJava(udtList_element, Optional.of(cassandraOptions)));
return EntityWithUDTForDynamicKeyspace_Update.Cols.this;
}
/**
* Generate an UPDATE FROM ... SET udtList[index] = null */
public final EntityWithUDTForDynamicKeyspace_Update.Cols RemoveAtIndex(final int index) {
where.with(QueryBuilder.setIdx("udtlist", index, QueryBuilder.bindMarker("udtlist")));
boundValues.add(null);
encodedValues.add(null);
return EntityWithUDTForDynamicKeyspace_Update.Cols.this;
}
/**
* Generate an UPDATE FROM ... SET udtList = udtList - [?] */
@SuppressWarnings("static-access")
public final EntityWithUDTForDynamicKeyspace_Update.Cols RemoveFrom(final UDTWithNoKeyspace udtList_element) {
where.with(QueryBuilder.discardAll("udtlist", QueryBuilder.bindMarker("udtlist")));
boundValues.add(Arrays.asList(udtList_element));
encodedValues.add(meta.udtList.encodeFromJava(Arrays.asList(udtList_element), Optional.of(cassandraOptions)));
return EntityWithUDTForDynamicKeyspace_Update.Cols.this;
}
/**
* Generate an UPDATE FROM ... SET udtList = udtList - ? */
@SuppressWarnings("static-access")
public final EntityWithUDTForDynamicKeyspace_Update.Cols RemoveAllFrom(final List udtList_element) {
where.with(QueryBuilder.discardAll("udtlist", QueryBuilder.bindMarker("udtlist")));
boundValues.add(udtList_element);
encodedValues.add(meta.udtList.encodeFromJava(udtList_element, Optional.of(cassandraOptions)));
return EntityWithUDTForDynamicKeyspace_Update.Cols.this;
}
/**
* Generate an UPDATE FROM ... SET udtList = ? */
@SuppressWarnings("static-access")
public final EntityWithUDTForDynamicKeyspace_Update.Cols Set(final List udtList_element) {
where.with(NonEscapingSetAssignment.of("udtlist", QueryBuilder.bindMarker("udtlist")));
boundValues.add(udtList_element);
encodedValues.add(meta.udtList.encodeFromJava(udtList_element, Optional.of(cassandraOptions)));
return EntityWithUDTForDynamicKeyspace_Update.Cols.this;
}
}
public final class UdtSet {
/**
* Generate an UPDATE FROM ... SET udtset = udtset + {?} */
@SuppressWarnings("static-access")
public final EntityWithUDTForDynamicKeyspace_Update.Cols AddTo(final UDTWithNoKeyspace udtSet_element) {
where.with(QueryBuilder.addAll("udtset", QueryBuilder.bindMarker("udtset")));
boundValues.add(Sets.newHashSet(udtSet_element));
encodedValues.add(meta.udtSet.encodeFromJava(Sets.newHashSet(udtSet_element), Optional.of(cassandraOptions)));
return EntityWithUDTForDynamicKeyspace_Update.Cols.this;
}
/**
* Generate an UPDATE FROM ... SET udtSet = udtSet + ? */
@SuppressWarnings("static-access")
public final EntityWithUDTForDynamicKeyspace_Update.Cols AddAllTo(final Set udtSet_element) {
where.with(QueryBuilder.addAll("udtset", QueryBuilder.bindMarker("udtset")));
boundValues.add(udtSet_element);
encodedValues.add(meta.udtSet.encodeFromJava(udtSet_element, Optional.of(cassandraOptions)));
return EntityWithUDTForDynamicKeyspace_Update.Cols.this;
}
/**
* Generate an UPDATE FROM ... SET udtSet = udtSet - {?} */
@SuppressWarnings("static-access")
public final EntityWithUDTForDynamicKeyspace_Update.Cols RemoveFrom(final UDTWithNoKeyspace udtSet_element) {
where.with(QueryBuilder.removeAll("udtset", QueryBuilder.bindMarker("udtset")));
boundValues.add(Sets.newHashSet(udtSet_element));
encodedValues.add(meta.udtSet.encodeFromJava(Sets.newHashSet(udtSet_element), Optional.of(cassandraOptions)));
return EntityWithUDTForDynamicKeyspace_Update.Cols.this;
}
/**
* Generate an UPDATE FROM ... SET udtSet = udtSet - ? */
@SuppressWarnings("static-access")
public final EntityWithUDTForDynamicKeyspace_Update.Cols RemoveAllFrom(final Set udtSet_element) {
where.with(QueryBuilder.removeAll("udtset", QueryBuilder.bindMarker("udtset")));
boundValues.add(udtSet_element);
encodedValues.add(meta.udtSet.encodeFromJava(udtSet_element, Optional.of(cassandraOptions)));
return EntityWithUDTForDynamicKeyspace_Update.Cols.this;
}
/**
* Generate an UPDATE FROM ... SET udtSet = ? */
@SuppressWarnings("static-access")
public final EntityWithUDTForDynamicKeyspace_Update.Cols Set(final Set udtSet_element) {
where.with(NonEscapingSetAssignment.of("udtset", QueryBuilder.bindMarker("udtset")));
boundValues.add(udtSet_element);
encodedValues.add(meta.udtSet.encodeFromJava(udtSet_element, Optional.of(cassandraOptions)));
return EntityWithUDTForDynamicKeyspace_Update.Cols.this;
}
}
public final class UdtMapKey {
/**
* Generate an UPDATE FROM ... SET udtmapkey[?] = ? */
public final EntityWithUDTForDynamicKeyspace_Update.Cols PutTo(final UDTWithNoKeyspace udtMapKey_key, final String udtMapKey_value) {
where.with(QueryBuilder.put("udtmapkey", QueryBuilder.bindMarker("udtMapKey_key"), QueryBuilder.bindMarker("udtMapKey_value")));
boundValues.add(udtMapKey_key);
boundValues.add(udtMapKey_value);
encodedValues.add(meta.udtMapKey.keyProperty.encodeFromJava(udtMapKey_key, Optional.of(cassandraOptions)));
encodedValues.add(meta.udtMapKey.valueProperty.encodeFromJava(udtMapKey_value, Optional.of(cassandraOptions)));
return EntityWithUDTForDynamicKeyspace_Update.Cols.this;
}
/**
* Generate an UPDATE FROM ... SET udtMapKey = udtMapKey + ? */
@SuppressWarnings("static-access")
public final EntityWithUDTForDynamicKeyspace_Update.Cols AddAllTo(final Map udtMapKey) {
where.with(QueryBuilder.addAll("udtmapkey", QueryBuilder.bindMarker("udtmapkey")));
boundValues.add(udtMapKey);
encodedValues.add(meta.udtMapKey.encodeFromJava(udtMapKey, Optional.of(cassandraOptions)));
return EntityWithUDTForDynamicKeyspace_Update.Cols.this;
}
/**
* Generate an UPDATE FROM ... SET udtMapKey[?] = null */
@SuppressWarnings("static-access")
public final EntityWithUDTForDynamicKeyspace_Update.Cols RemoveByKey(final UDTWithNoKeyspace udtMapKey_key) {
where.with(QueryBuilder.put("udtmapkey", QueryBuilder.bindMarker("udtMapKey_key"), QueryBuilder.bindMarker("udtMapKey_value")));
boundValues.add(udtMapKey_key);
boundValues.add(null);
encodedValues.add(meta.udtMapKey.keyProperty.encodeFromJava(udtMapKey_key, Optional.of(cassandraOptions)));
encodedValues.add(null);
return EntityWithUDTForDynamicKeyspace_Update.Cols.this;
}
/**
* Generate an UPDATE FROM ... SET udtMapKey = ? */
@SuppressWarnings("static-access")
public final EntityWithUDTForDynamicKeyspace_Update.Cols Set(final Map udtMapKey) {
where.with(NonEscapingSetAssignment.of("udtmapkey", QueryBuilder.bindMarker("udtmapkey")));
boundValues.add(udtMapKey);
encodedValues.add(meta.udtMapKey.encodeFromJava(udtMapKey, Optional.of(cassandraOptions)));
return EntityWithUDTForDynamicKeyspace_Update.Cols.this;
}
}
public final class UdtMapValue {
/**
* Generate an UPDATE FROM ... SET udtmapvalue[?] = ? */
public final EntityWithUDTForDynamicKeyspace_Update.Cols PutTo(final Integer udtMapValue_key, final UDTWithNoKeyspace udtMapValue_value) {
where.with(QueryBuilder.put("udtmapvalue", QueryBuilder.bindMarker("udtMapValue_key"), QueryBuilder.bindMarker("udtMapValue_value")));
boundValues.add(udtMapValue_key);
boundValues.add(udtMapValue_value);
encodedValues.add(meta.udtMapValue.keyProperty.encodeFromJava(udtMapValue_key, Optional.of(cassandraOptions)));
encodedValues.add(meta.udtMapValue.valueProperty.encodeFromJava(udtMapValue_value, Optional.of(cassandraOptions)));
return EntityWithUDTForDynamicKeyspace_Update.Cols.this;
}
/**
* Generate an UPDATE FROM ... SET udtMapValue = udtMapValue + ? */
@SuppressWarnings("static-access")
public final EntityWithUDTForDynamicKeyspace_Update.Cols AddAllTo(final Map udtMapValue) {
where.with(QueryBuilder.addAll("udtmapvalue", QueryBuilder.bindMarker("udtmapvalue")));
boundValues.add(udtMapValue);
encodedValues.add(meta.udtMapValue.encodeFromJava(udtMapValue, Optional.of(cassandraOptions)));
return EntityWithUDTForDynamicKeyspace_Update.Cols.this;
}
/**
* Generate an UPDATE FROM ... SET udtMapValue[?] = null */
@SuppressWarnings("static-access")
public final EntityWithUDTForDynamicKeyspace_Update.Cols RemoveByKey(final Integer udtMapValue_key) {
where.with(QueryBuilder.put("udtmapvalue", QueryBuilder.bindMarker("udtMapValue_key"), QueryBuilder.bindMarker("udtMapValue_value")));
boundValues.add(udtMapValue_key);
boundValues.add(null);
encodedValues.add(meta.udtMapValue.keyProperty.encodeFromJava(udtMapValue_key, Optional.of(cassandraOptions)));
encodedValues.add(null);
return EntityWithUDTForDynamicKeyspace_Update.Cols.this;
}
/**
* Generate an UPDATE FROM ... SET udtMapValue = ? */
@SuppressWarnings("static-access")
public final EntityWithUDTForDynamicKeyspace_Update.Cols Set(final Map udtMapValue) {
where.with(NonEscapingSetAssignment.of("udtmapvalue", QueryBuilder.bindMarker("udtmapvalue")));
boundValues.add(udtMapValue);
encodedValues.add(meta.udtMapValue.encodeFromJava(udtMapValue, Optional.of(cassandraOptions)));
return EntityWithUDTForDynamicKeyspace_Update.Cols.this;
}
}
}
public class F extends AbstractUpdateFrom {
F(Update.Where where, CassandraOptions cassandraOptions) {
super(where, cassandraOptions);
}
public final F.Udt udt() {
return new F.Udt();
}
public final F.UdtList udtList() {
return new F.UdtList();
}
public final F.UdtSet udtSet() {
return new F.UdtSet();
}
public final F.UdtMapKey udtMapKey() {
return new F.UdtMapKey();
}
public final F.UdtMapValue udtMapValue() {
return new F.UdtMapValue();
}
public final class Udt {
/**
* Generate an UPDATE FROM ... SET udt = ? */
@SuppressWarnings("static-access")
public final EntityWithUDTForDynamicKeyspace_Update.Cols Set(final UDTWithNoKeyspace udt) {
where.with(NonEscapingSetAssignment.of("udt", QueryBuilder.bindMarker("udt")));
boundValues.add(udt);
encodedValues.add(meta.udt.encodeFromJava(udt, Optional.of(cassandraOptions)));
return new EntityWithUDTForDynamicKeyspace_Update.Cols(where, cassandraOptions);
}
}
public final class UdtList {
/**
* Generate an UPDATE FROM ... SET udtlist = udtlist + [?] */
@SuppressWarnings("static-access")
public final EntityWithUDTForDynamicKeyspace_Update.Cols AppendTo(final UDTWithNoKeyspace udtList_element) {
where.with(QueryBuilder.appendAll("udtlist", QueryBuilder.bindMarker("udtlist")));
boundValues.add(Arrays.asList(udtList_element));
encodedValues.add(meta.udtList.encodeFromJava(Arrays.asList(udtList_element), Optional.of(cassandraOptions)));
return new EntityWithUDTForDynamicKeyspace_Update.Cols(where, cassandraOptions);
}
/**
* Generate an UPDATE FROM ... SET udtList = udtList + ? */
@SuppressWarnings("static-access")
public final EntityWithUDTForDynamicKeyspace_Update.Cols AppendAllTo(final List udtList_element) {
where.with(QueryBuilder.appendAll("udtlist", QueryBuilder.bindMarker("udtlist")));
boundValues.add(udtList_element);
encodedValues.add(meta.udtList.encodeFromJava(udtList_element, Optional.of(cassandraOptions)));
return new EntityWithUDTForDynamicKeyspace_Update.Cols(where, cassandraOptions);
}
/**
* Generate an UPDATE FROM ... SET udtList = [?] + udtList */
@SuppressWarnings("static-access")
public final EntityWithUDTForDynamicKeyspace_Update.Cols PrependTo(final UDTWithNoKeyspace udtList_element) {
where.with(QueryBuilder.prependAll("udtlist", QueryBuilder.bindMarker("udtlist")));
boundValues.add(Arrays.asList(udtList_element));
encodedValues.add(meta.udtList.encodeFromJava(Arrays.asList(udtList_element), Optional.of(cassandraOptions)));
return new EntityWithUDTForDynamicKeyspace_Update.Cols(where, cassandraOptions);
}
/**
* Generate an UPDATE FROM ... SET udtList = ? + udtList */
@SuppressWarnings("static-access")
public final EntityWithUDTForDynamicKeyspace_Update.Cols PrependAllTo(final List udtList_element) {
where.with(QueryBuilder.prependAll("udtlist", QueryBuilder.bindMarker("udtlist")));
boundValues.add(udtList_element);
encodedValues.add(meta.udtList.encodeFromJava(udtList_element, Optional.of(cassandraOptions)));
return new EntityWithUDTForDynamicKeyspace_Update.Cols(where, cassandraOptions);
}
/**
* Generate an UPDATE FROM ... SET udtList[index] = ? */
public final EntityWithUDTForDynamicKeyspace_Update.Cols SetAtIndex(final int index, final UDTWithNoKeyspace udtList_element) {
where.with(QueryBuilder.setIdx("udtlist", index, QueryBuilder.bindMarker("udtlist")));
boundValues.add(udtList_element);
encodedValues.add(meta.udtList.valueProperty.encodeFromJava(udtList_element, Optional.of(cassandraOptions)));
return new EntityWithUDTForDynamicKeyspace_Update.Cols(where, cassandraOptions);
}
/**
* Generate an UPDATE FROM ... SET udtList[index] = null */
public final EntityWithUDTForDynamicKeyspace_Update.Cols RemoveAtIndex(final int index) {
where.with(QueryBuilder.setIdx("udtlist", index, QueryBuilder.bindMarker("udtlist")));
boundValues.add(null);
encodedValues.add(null);
return new EntityWithUDTForDynamicKeyspace_Update.Cols(where, cassandraOptions);
}
/**
* Generate an UPDATE FROM ... SET udtList = udtList - [?] */
@SuppressWarnings("static-access")
public final EntityWithUDTForDynamicKeyspace_Update.Cols RemoveFrom(final UDTWithNoKeyspace udtList_element) {
where.with(QueryBuilder.discardAll("udtlist", QueryBuilder.bindMarker("udtlist")));
boundValues.add(Arrays.asList(udtList_element));
encodedValues.add(meta.udtList.encodeFromJava(Arrays.asList(udtList_element), Optional.of(cassandraOptions)));
return new EntityWithUDTForDynamicKeyspace_Update.Cols(where, cassandraOptions);
}
/**
* Generate an UPDATE FROM ... SET udtList = udtList - ? */
@SuppressWarnings("static-access")
public final EntityWithUDTForDynamicKeyspace_Update.Cols RemoveAllFrom(final List udtList_element) {
where.with(QueryBuilder.discardAll("udtlist", QueryBuilder.bindMarker("udtlist")));
boundValues.add(udtList_element);
encodedValues.add(meta.udtList.encodeFromJava(udtList_element, Optional.of(cassandraOptions)));
return new EntityWithUDTForDynamicKeyspace_Update.Cols(where, cassandraOptions);
}
/**
* Generate an UPDATE FROM ... SET udtList = ? */
@SuppressWarnings("static-access")
public final EntityWithUDTForDynamicKeyspace_Update.Cols Set(final List udtList_element) {
where.with(NonEscapingSetAssignment.of("udtlist", QueryBuilder.bindMarker("udtlist")));
boundValues.add(udtList_element);
encodedValues.add(meta.udtList.encodeFromJava(udtList_element, Optional.of(cassandraOptions)));
return new EntityWithUDTForDynamicKeyspace_Update.Cols(where, cassandraOptions);
}
}
public final class UdtSet {
/**
* Generate an UPDATE FROM ... SET udtset = udtset + {?} */
@SuppressWarnings("static-access")
public final EntityWithUDTForDynamicKeyspace_Update.Cols AddTo(final UDTWithNoKeyspace udtSet_element) {
where.with(QueryBuilder.addAll("udtset", QueryBuilder.bindMarker("udtset")));
boundValues.add(Sets.newHashSet(udtSet_element));
encodedValues.add(meta.udtSet.encodeFromJava(Sets.newHashSet(udtSet_element), Optional.of(cassandraOptions)));
return new EntityWithUDTForDynamicKeyspace_Update.Cols(where, cassandraOptions);
}
/**
* Generate an UPDATE FROM ... SET udtSet = udtSet + ? */
@SuppressWarnings("static-access")
public final EntityWithUDTForDynamicKeyspace_Update.Cols AddAllTo(final Set udtSet_element) {
where.with(QueryBuilder.addAll("udtset", QueryBuilder.bindMarker("udtset")));
boundValues.add(udtSet_element);
encodedValues.add(meta.udtSet.encodeFromJava(udtSet_element, Optional.of(cassandraOptions)));
return new EntityWithUDTForDynamicKeyspace_Update.Cols(where, cassandraOptions);
}
/**
* Generate an UPDATE FROM ... SET udtSet = udtSet - {?} */
@SuppressWarnings("static-access")
public final EntityWithUDTForDynamicKeyspace_Update.Cols RemoveFrom(final UDTWithNoKeyspace udtSet_element) {
where.with(QueryBuilder.removeAll("udtset", QueryBuilder.bindMarker("udtset")));
boundValues.add(Sets.newHashSet(udtSet_element));
encodedValues.add(meta.udtSet.encodeFromJava(Sets.newHashSet(udtSet_element), Optional.of(cassandraOptions)));
return new EntityWithUDTForDynamicKeyspace_Update.Cols(where, cassandraOptions);
}
/**
* Generate an UPDATE FROM ... SET udtSet = udtSet - ? */
@SuppressWarnings("static-access")
public final EntityWithUDTForDynamicKeyspace_Update.Cols RemoveAllFrom(final Set udtSet_element) {
where.with(QueryBuilder.removeAll("udtset", QueryBuilder.bindMarker("udtset")));
boundValues.add(udtSet_element);
encodedValues.add(meta.udtSet.encodeFromJava(udtSet_element, Optional.of(cassandraOptions)));
return new EntityWithUDTForDynamicKeyspace_Update.Cols(where, cassandraOptions);
}
/**
* Generate an UPDATE FROM ... SET udtSet = ? */
@SuppressWarnings("static-access")
public final EntityWithUDTForDynamicKeyspace_Update.Cols Set(final Set udtSet_element) {
where.with(NonEscapingSetAssignment.of("udtset", QueryBuilder.bindMarker("udtset")));
boundValues.add(udtSet_element);
encodedValues.add(meta.udtSet.encodeFromJava(udtSet_element, Optional.of(cassandraOptions)));
return new EntityWithUDTForDynamicKeyspace_Update.Cols(where, cassandraOptions);
}
}
public final class UdtMapKey {
/**
* Generate an UPDATE FROM ... SET udtmapkey[?] = ? */
public final EntityWithUDTForDynamicKeyspace_Update.Cols PutTo(final UDTWithNoKeyspace udtMapKey_key, final String udtMapKey_value) {
where.with(QueryBuilder.put("udtmapkey", QueryBuilder.bindMarker("udtMapKey_key"), QueryBuilder.bindMarker("udtMapKey_value")));
boundValues.add(udtMapKey_key);
boundValues.add(udtMapKey_value);
encodedValues.add(meta.udtMapKey.keyProperty.encodeFromJava(udtMapKey_key, Optional.of(cassandraOptions)));
encodedValues.add(meta.udtMapKey.valueProperty.encodeFromJava(udtMapKey_value, Optional.of(cassandraOptions)));
return new EntityWithUDTForDynamicKeyspace_Update.Cols(where, cassandraOptions);
}
/**
* Generate an UPDATE FROM ... SET udtMapKey = udtMapKey + ? */
@SuppressWarnings("static-access")
public final EntityWithUDTForDynamicKeyspace_Update.Cols AddAllTo(final Map udtMapKey) {
where.with(QueryBuilder.addAll("udtmapkey", QueryBuilder.bindMarker("udtmapkey")));
boundValues.add(udtMapKey);
encodedValues.add(meta.udtMapKey.encodeFromJava(udtMapKey, Optional.of(cassandraOptions)));
return new EntityWithUDTForDynamicKeyspace_Update.Cols(where, cassandraOptions);
}
/**
* Generate an UPDATE FROM ... SET udtMapKey[?] = null */
@SuppressWarnings("static-access")
public final EntityWithUDTForDynamicKeyspace_Update.Cols RemoveByKey(final UDTWithNoKeyspace udtMapKey_key) {
where.with(QueryBuilder.put("udtmapkey", QueryBuilder.bindMarker("udtMapKey_key"), QueryBuilder.bindMarker("udtMapKey_value")));
boundValues.add(udtMapKey_key);
boundValues.add(null);
encodedValues.add(meta.udtMapKey.keyProperty.encodeFromJava(udtMapKey_key, Optional.of(cassandraOptions)));
encodedValues.add(null);
return new EntityWithUDTForDynamicKeyspace_Update.Cols(where, cassandraOptions);
}
/**
* Generate an UPDATE FROM ... SET udtMapKey = ? */
@SuppressWarnings("static-access")
public final EntityWithUDTForDynamicKeyspace_Update.Cols Set(final Map udtMapKey) {
where.with(NonEscapingSetAssignment.of("udtmapkey", QueryBuilder.bindMarker("udtmapkey")));
boundValues.add(udtMapKey);
encodedValues.add(meta.udtMapKey.encodeFromJava(udtMapKey, Optional.of(cassandraOptions)));
return new EntityWithUDTForDynamicKeyspace_Update.Cols(where, cassandraOptions);
}
}
public final class UdtMapValue {
/**
* Generate an UPDATE FROM ... SET udtmapvalue[?] = ? */
public final EntityWithUDTForDynamicKeyspace_Update.Cols PutTo(final Integer udtMapValue_key, final UDTWithNoKeyspace udtMapValue_value) {
where.with(QueryBuilder.put("udtmapvalue", QueryBuilder.bindMarker("udtMapValue_key"), QueryBuilder.bindMarker("udtMapValue_value")));
boundValues.add(udtMapValue_key);
boundValues.add(udtMapValue_value);
encodedValues.add(meta.udtMapValue.keyProperty.encodeFromJava(udtMapValue_key, Optional.of(cassandraOptions)));
encodedValues.add(meta.udtMapValue.valueProperty.encodeFromJava(udtMapValue_value, Optional.of(cassandraOptions)));
return new EntityWithUDTForDynamicKeyspace_Update.Cols(where, cassandraOptions);
}
/**
* Generate an UPDATE FROM ... SET udtMapValue = udtMapValue + ? */
@SuppressWarnings("static-access")
public final EntityWithUDTForDynamicKeyspace_Update.Cols AddAllTo(final Map udtMapValue) {
where.with(QueryBuilder.addAll("udtmapvalue", QueryBuilder.bindMarker("udtmapvalue")));
boundValues.add(udtMapValue);
encodedValues.add(meta.udtMapValue.encodeFromJava(udtMapValue, Optional.of(cassandraOptions)));
return new EntityWithUDTForDynamicKeyspace_Update.Cols(where, cassandraOptions);
}
/**
* Generate an UPDATE FROM ... SET udtMapValue[?] = null */
@SuppressWarnings("static-access")
public final EntityWithUDTForDynamicKeyspace_Update.Cols RemoveByKey(final Integer udtMapValue_key) {
where.with(QueryBuilder.put("udtmapvalue", QueryBuilder.bindMarker("udtMapValue_key"), QueryBuilder.bindMarker("udtMapValue_value")));
boundValues.add(udtMapValue_key);
boundValues.add(null);
encodedValues.add(meta.udtMapValue.keyProperty.encodeFromJava(udtMapValue_key, Optional.of(cassandraOptions)));
encodedValues.add(null);
return new EntityWithUDTForDynamicKeyspace_Update.Cols(where, cassandraOptions);
}
/**
* Generate an UPDATE FROM ... SET udtMapValue = ? */
@SuppressWarnings("static-access")
public final EntityWithUDTForDynamicKeyspace_Update.Cols Set(final Map udtMapValue) {
where.with(NonEscapingSetAssignment.of("udtmapvalue", QueryBuilder.bindMarker("udtmapvalue")));
boundValues.add(udtMapValue);
encodedValues.add(meta.udtMapValue.encodeFromJava(udtMapValue, Optional.of(cassandraOptions)));
return new EntityWithUDTForDynamicKeyspace_Update.Cols(where, cassandraOptions);
}
}
}
public final class W_Id extends AbstractUpdateWhere {
public W_Id(Update.Where where, CassandraOptions cassandraOptions) {
super(where, cassandraOptions);
}
public final EntityWithUDTForDynamicKeyspace_Update.W_Id.Relation id() {
return new EntityWithUDTForDynamicKeyspace_Update.W_Id.Relation();
}
public final class Relation {
/**
* Generate a SELECT ... FROM ... WHERE ... id = ? */
@SuppressWarnings("static-access")
public final EntityWithUDTForDynamicKeyspace_Update.W_Clust Eq(Long id) {
where.and(QueryBuilder.eq("id", QueryBuilder.bindMarker("id")));
boundValues.add(id);
encodedValues.add(meta.id.encodeFromJava(id, Optional.of(cassandraOptions)));
return new EntityWithUDTForDynamicKeyspace_Update.W_Clust(where, cassandraOptions);
}
/**
* Generate a SELECT ... FROM ... WHERE ... id IN ? */
@SuppressWarnings("static-access")
public final EntityWithUDTForDynamicKeyspace_Update.W_Clust IN(Long... id) {
Validator.validateTrue(ArrayUtils.isNotEmpty(id), "Varargs for field '%s' should not be null/empty", "id");
where.and(QueryBuilder.in("id",QueryBuilder.bindMarker("id")));
final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy