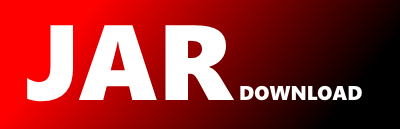
info.archinnov.achilles.generated.meta.entity.EntityWithComplexIndices_AchillesMeta Maven / Gradle / Ivy
The newest version!
package info.archinnov.achilles.generated.meta.entity;
import com.datastax.driver.core.ClusteringOrder;
import com.datastax.driver.core.ConsistencyLevel;
import com.datastax.driver.core.DataType;
import com.datastax.driver.core.Row;
import com.google.common.collect.BiMap;
import com.google.common.collect.HashBiMap;
import com.google.common.reflect.TypeToken;
import info.archinnov.achilles.generated.function.Integer_Type;
import info.archinnov.achilles.generated.function.List_String_Type;
import info.archinnov.achilles.generated.function.Long_Type;
import info.archinnov.achilles.generated.function.Map_Integer_String_Type;
import info.archinnov.achilles.generated.function.Map_String_String_Type;
import info.archinnov.achilles.generated.function.PartitionKeys_Type;
import info.archinnov.achilles.generated.function.Set_String_Type;
import info.archinnov.achilles.generated.function.String_Type;
import info.archinnov.achilles.internals.apt.annotations.AchillesMeta;
import info.archinnov.achilles.internals.codec.FallThroughCodec;
import info.archinnov.achilles.internals.entities.EntityWithComplexIndices;
import info.archinnov.achilles.internals.metamodel.AbstractEntityProperty;
import info.archinnov.achilles.internals.metamodel.AbstractProperty;
import info.archinnov.achilles.internals.metamodel.ListProperty;
import info.archinnov.achilles.internals.metamodel.MapProperty;
import info.archinnov.achilles.internals.metamodel.SetProperty;
import info.archinnov.achilles.internals.metamodel.SimpleProperty;
import info.archinnov.achilles.internals.metamodel.columns.ClusteringColumnInfo;
import info.archinnov.achilles.internals.metamodel.columns.ColumnInfo;
import info.archinnov.achilles.internals.metamodel.columns.ColumnType;
import info.archinnov.achilles.internals.metamodel.columns.FieldInfo;
import info.archinnov.achilles.internals.metamodel.columns.PartitionKeyInfo;
import info.archinnov.achilles.internals.metamodel.index.IndexInfo;
import info.archinnov.achilles.internals.metamodel.index.IndexType;
import info.archinnov.achilles.internals.strategy.naming.InternalNamingStrategy;
import info.archinnov.achilles.type.strategy.InsertStrategy;
import java.lang.Class;
import java.lang.Integer;
import java.lang.Long;
import java.lang.Override;
import java.lang.String;
import java.lang.SuppressWarnings;
import java.lang.UnsupportedOperationException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.Set;
/**
* Meta class of all entities of type EntityWithComplexIndices
* The meta class is responsible for
*
* - determining runtime consistency levels (read/write,serial)
* - determining runtime insert strategy
* - trigger event interceptors (if any)
* - map a Row back to an instance of EntityWithComplexIndices
* - determine runtime keyspace name using static annotations and runtime SchemaNameProvider (if any)
* - determine runtime table name using static annotations and runtime SchemaNameProvider (if any)
* - generate schema during bootstrap
* - validate schema during bootstrap
* - expose all property meta classes for encoding/decoding purpose on unitary columns
*
*/
@AchillesMeta
public final class EntityWithComplexIndices_AchillesMeta extends AbstractEntityProperty {
/**
* Meta class for 'id' property
* The meta class exposes some useful methods:
* - encodeFromJava: encode a property from raw Java to CQL java compatible type
* - encodeField: extract the current property value from the given EntityWithComplexIndices instance and encode to CQL java compatible type
* - decodeFromGettable: decode from a {@link com.datastax.driver.core.GettableData} instance (Row, UDTValue, TupleValue) the current property
*
*/
@SuppressWarnings({"serial", "unchecked"})
public static final SimpleProperty id = new SimpleProperty(new FieldInfo<>((EntityWithComplexIndices entity$) -> entity$.getId(), (EntityWithComplexIndices entity$, Long value$) -> entity$.setId(value$), "id", "id", ColumnType.PARTITION, new PartitionKeyInfo(1, false), IndexInfo.noIndex()), DataType.bigint(), gettableData$ -> gettableData$.get("id", java.lang.Long.class), (settableData$, value$) -> settableData$.set("id", value$, java.lang.Long.class), new TypeToken(){}, new TypeToken(){}, new FallThroughCodec<>(Long.class));
/**
* Meta class for 'clust1' property
* The meta class exposes some useful methods:
* - encodeFromJava: encode a property from raw Java to CQL java compatible type
* - encodeField: extract the current property value from the given EntityWithComplexIndices instance and encode to CQL java compatible type
* - decodeFromGettable: decode from a {@link com.datastax.driver.core.GettableData} instance (Row, UDTValue, TupleValue) the current property
*
*/
@SuppressWarnings({"serial", "unchecked"})
public static final SimpleProperty clust1 = new SimpleProperty(new FieldInfo<>((EntityWithComplexIndices entity$) -> entity$.getClust1(), (EntityWithComplexIndices entity$, Integer value$) -> entity$.setClust1(value$), "clust1", "clust1", ColumnType.CLUSTERING, new ClusteringColumnInfo(1, false, ClusteringOrder.ASC), IndexInfo.noIndex()), DataType.cint(), gettableData$ -> gettableData$.get("clust1", int.class), (settableData$, value$) -> settableData$.set("clust1", value$, int.class), new TypeToken(){}, new TypeToken(){}, new FallThroughCodec<>(Integer.class));
/**
* Meta class for 'clust2' property
* The meta class exposes some useful methods:
* - encodeFromJava: encode a property from raw Java to CQL java compatible type
* - encodeField: extract the current property value from the given EntityWithComplexIndices instance and encode to CQL java compatible type
* - decodeFromGettable: decode from a {@link com.datastax.driver.core.GettableData} instance (Row, UDTValue, TupleValue) the current property
*
*/
@SuppressWarnings({"serial", "unchecked"})
public static final SimpleProperty clust2 = new SimpleProperty(new FieldInfo<>((EntityWithComplexIndices entity$) -> entity$.getClust2(), (EntityWithComplexIndices entity$, Integer value$) -> entity$.setClust2(value$), "clust2", "clust2", ColumnType.CLUSTERING, new ClusteringColumnInfo(2, false, ClusteringOrder.ASC), IndexInfo.noIndex()), DataType.cint(), gettableData$ -> gettableData$.get("clust2", int.class), (settableData$, value$) -> settableData$.set("clust2", value$, int.class), new TypeToken(){}, new TypeToken(){}, new FallThroughCodec<>(Integer.class));
/**
* Meta class for 'clust3' property
* The meta class exposes some useful methods:
* - encodeFromJava: encode a property from raw Java to CQL java compatible type
* - encodeField: extract the current property value from the given EntityWithComplexIndices instance and encode to CQL java compatible type
* - decodeFromGettable: decode from a {@link com.datastax.driver.core.GettableData} instance (Row, UDTValue, TupleValue) the current property
*
*/
@SuppressWarnings({"serial", "unchecked"})
public static final SimpleProperty clust3 = new SimpleProperty(new FieldInfo<>((EntityWithComplexIndices entity$) -> entity$.getClust3(), (EntityWithComplexIndices entity$, String value$) -> entity$.setClust3(value$), "clust3", "clust3", ColumnType.CLUSTERING, new ClusteringColumnInfo(3, false, ClusteringOrder.ASC), IndexInfo.noIndex()), DataType.text(), gettableData$ -> gettableData$.get("clust3", java.lang.String.class), (settableData$, value$) -> settableData$.set("clust3", value$, java.lang.String.class), new TypeToken(){}, new TypeToken(){}, new FallThroughCodec<>(String.class));
/**
* Meta class for 'simpleIndex' property
* The meta class exposes some useful methods:
* - encodeFromJava: encode a property from raw Java to CQL java compatible type
* - encodeField: extract the current property value from the given EntityWithComplexIndices instance and encode to CQL java compatible type
* - decodeFromGettable: decode from a {@link com.datastax.driver.core.GettableData} instance (Row, UDTValue, TupleValue) the current property
*
*/
@SuppressWarnings({"serial", "unchecked"})
public static final SimpleProperty simpleIndex = new SimpleProperty(new FieldInfo<>((EntityWithComplexIndices entity$) -> entity$.getSimpleIndex(), (EntityWithComplexIndices entity$, String value$) -> entity$.setSimpleIndex(value$), "simpleIndex", "simpleindex", ColumnType.NORMAL, new ColumnInfo(false), IndexInfo.forNative(IndexType.NORMAL, "simpleindex_index", "", "")), DataType.text(), gettableData$ -> gettableData$.get("simpleindex", java.lang.String.class), (settableData$, value$) -> settableData$.set("simpleindex", value$, java.lang.String.class), new TypeToken(){}, new TypeToken(){}, new FallThroughCodec<>(String.class));
/**
* Meta class for 'collectionIndex' property
* The meta class exposes some useful methods:
* - encodeFromJava: encode a property from raw Java to CQL java compatible type
* - encodeField: extract the current property value from the given EntityWithComplexIndices instance and encode to CQL java compatible type
* - decodeFromGettable: decode from a {@link com.datastax.driver.core.GettableData} instance (Row, UDTValue, TupleValue) the current property
*
*/
@SuppressWarnings({"serial", "unchecked"})
public static final ListProperty collectionIndex = new ListProperty<>(new FieldInfo<>((EntityWithComplexIndices entity$) -> entity$.getCollectionIndex(), (EntityWithComplexIndices entity$, List value$) -> entity$.setCollectionIndex(value$), "collectionIndex", "collectionindex", ColumnType.NORMAL, new ColumnInfo(false), IndexInfo.forNative(IndexType.COLLECTION, "collectionindex_index", "", "")), false, false, String.class, new SimpleProperty(FieldInfo. of("collectionIndex", "collectionindex", true), DataType.text(), gettable$ -> null, (udt$, value$) -> {}, new TypeToken(){}, new TypeToken(){}, new FallThroughCodec<>(String.class)));
/**
* Meta class for 'fullIndexOnCollection' property
* The meta class exposes some useful methods:
* - encodeFromJava: encode a property from raw Java to CQL java compatible type
* - encodeField: extract the current property value from the given EntityWithComplexIndices instance and encode to CQL java compatible type
* - decodeFromGettable: decode from a {@link com.datastax.driver.core.GettableData} instance (Row, UDTValue, TupleValue) the current property
*
*/
@SuppressWarnings({"serial", "unchecked"})
public static final SetProperty fullIndexOnCollection = new SetProperty<>(new FieldInfo<>((EntityWithComplexIndices entity$) -> entity$.getFullIndexOnCollection(), (EntityWithComplexIndices entity$, Set value$) -> entity$.setFullIndexOnCollection(value$), "fullIndexOnCollection", "fullindexoncollection", ColumnType.NORMAL, new ColumnInfo(true), IndexInfo.forNative(IndexType.FULL, "fullindexoncollection_index", "", "")), true, false, String.class, new SimpleProperty(FieldInfo. of("fullIndexOnCollection", "fullindexoncollection", true), DataType.text(), gettable$ -> null, (udt$, value$) -> {}, new TypeToken(){}, new TypeToken(){}, new FallThroughCodec<>(String.class)));
/**
* Meta class for 'indexOnMapKey' property
* The meta class exposes some useful methods:
* - encodeFromJava: encode a property from raw Java to CQL java compatible type
* - encodeField: extract the current property value from the given EntityWithComplexIndices instance and encode to CQL java compatible type
* - decodeFromGettable: decode from a {@link com.datastax.driver.core.GettableData} instance (Row, UDTValue, TupleValue) the current property
*
*/
@SuppressWarnings({"serial", "unchecked"})
public static final MapProperty indexOnMapKey = new MapProperty(new FieldInfo<>((EntityWithComplexIndices entity$) -> entity$.getIndexOnMapKey(), (EntityWithComplexIndices entity$, Map value$) -> entity$.setIndexOnMapKey(value$), "indexOnMapKey", "indexonmapkey", ColumnType.NORMAL, new ColumnInfo(false), IndexInfo.forNative(IndexType.MAP_KEY, "indexonmapkey_index", "", "")), false, false, new SimpleProperty(FieldInfo. of("indexOnMapKey", "indexonmapkey", true), DataType.text(), gettable$ -> null, (udt$, value$) -> {}, new TypeToken(){}, new TypeToken(){}, new FallThroughCodec<>(String.class)), new SimpleProperty(FieldInfo. of("indexOnMapKey", "indexonmapkey", true), DataType.text(), gettable$ -> null, (udt$, value$) -> {}, new TypeToken(){}, new TypeToken(){}, new FallThroughCodec<>(String.class)));
/**
* Meta class for 'indexOnMapValue' property
* The meta class exposes some useful methods:
* - encodeFromJava: encode a property from raw Java to CQL java compatible type
* - encodeField: extract the current property value from the given EntityWithComplexIndices instance and encode to CQL java compatible type
* - decodeFromGettable: decode from a {@link com.datastax.driver.core.GettableData} instance (Row, UDTValue, TupleValue) the current property
*
*/
@SuppressWarnings({"serial", "unchecked"})
public static final MapProperty indexOnMapValue = new MapProperty(new FieldInfo<>((EntityWithComplexIndices entity$) -> entity$.getIndexOnMapValue(), (EntityWithComplexIndices entity$, Map value$) -> entity$.setIndexOnMapValue(value$), "indexOnMapValue", "indexonmapvalue", ColumnType.NORMAL, new ColumnInfo(false), IndexInfo.forNative(IndexType.MAP_VALUE, "indexonmapvalue_index", "", "")), false, false, new SimpleProperty(FieldInfo. of("indexOnMapValue", "indexonmapvalue", true), DataType.cint(), gettable$ -> null, (udt$, value$) -> {}, new TypeToken(){}, new TypeToken(){}, new FallThroughCodec<>(Integer.class)), new SimpleProperty(FieldInfo. of("indexOnMapValue", "indexonmapvalue", true), DataType.text(), gettable$ -> null, (udt$, value$) -> {}, new TypeToken(){}, new TypeToken(){}, new FallThroughCodec<>(String.class)));
/**
* Meta class for 'indexOnMapEntry' property
* The meta class exposes some useful methods:
* - encodeFromJava: encode a property from raw Java to CQL java compatible type
* - encodeField: extract the current property value from the given EntityWithComplexIndices instance and encode to CQL java compatible type
* - decodeFromGettable: decode from a {@link com.datastax.driver.core.GettableData} instance (Row, UDTValue, TupleValue) the current property
*
*/
@SuppressWarnings({"serial", "unchecked"})
public static final MapProperty indexOnMapEntry = new MapProperty(new FieldInfo<>((EntityWithComplexIndices entity$) -> entity$.getIndexOnMapEntry(), (EntityWithComplexIndices entity$, Map value$) -> entity$.setIndexOnMapEntry(value$), "indexOnMapEntry", "indexonmapentry", ColumnType.NORMAL, new ColumnInfo(false), IndexInfo.forNative(IndexType.MAP_ENTRY, "indexonmapentry_index", "", "")), false, false, new SimpleProperty(FieldInfo. of("indexOnMapEntry", "indexonmapentry", true), DataType.cint(), gettable$ -> null, (udt$, value$) -> {}, new TypeToken(){}, new TypeToken(){}, new FallThroughCodec<>(Integer.class)), new SimpleProperty(FieldInfo. of("indexOnMapEntry", "indexonmapentry", true), DataType.text(), gettable$ -> null, (udt$, value$) -> {}, new TypeToken(){}, new TypeToken(){}, new FallThroughCodec<>(String.class)));
/**
* Static class to expose "EntityWithComplexIndices_AchillesMeta" fields for type-safe function calls */
public static final EntityWithComplexIndices_AchillesMeta.ColumnsForFunctions COLUMNS = new EntityWithComplexIndices_AchillesMeta.ColumnsForFunctions();
;
@Override
protected Class getEntityClass() {
return EntityWithComplexIndices.class;
}
@Override
protected String getDerivedTableOrViewName() {
return "entitywithcomplexindices";
}
@Override
protected BiMap fieldNameToCqlColumn() {
BiMap map = HashBiMap.create(10);
map.put("id", "id");
map.put("clust1", "clust1");
map.put("clust2", "clust2");
map.put("clust3", "clust3");
map.put("simpleIndex", "simpleindex");
map.put("collectionIndex", "collectionindex");
map.put("fullIndexOnCollection", "fullindexoncollection");
map.put("indexOnMapKey", "indexonmapkey");
map.put("indexOnMapValue", "indexonmapvalue");
map.put("indexOnMapEntry", "indexonmapentry");
return map;
}
@Override
protected Optional getStaticReadConsistency() {
return Optional.empty();
}
@Override
protected Optional getStaticNamingStrategy() {
return Optional.empty();
}
@Override
protected List> getPartitionKeys() {
return Arrays.asList(id);
}
@Override
protected List> getClusteringColumns() {
return Arrays.asList(clust1,clust2,clust3);
}
@Override
protected List> getNormalColumns() {
return Arrays.asList(collectionIndex,fullIndexOnCollection,indexOnMapEntry,indexOnMapKey,indexOnMapValue,simpleIndex);
}
@Override
protected List> getComputedColumns() {
return Arrays.asList();
}
@Override
protected List> getConstructorInjectedColumns() {
return Arrays.asList();
}
@Override
protected boolean isCounterTable() {
return false;
}
@Override
protected Optional getStaticKeyspace() {
return Optional.empty();
}
@Override
protected Optional getStaticTableOrViewName() {
return Optional.empty();
}
@Override
protected Optional getStaticWriteConsistency() {
return Optional.empty();
}
@Override
protected Optional getStaticSerialConsistency() {
return Optional.empty();
}
@Override
protected Optional getStaticTTL() {
return Optional.empty();
}
@Override
protected Optional getStaticInsertStrategy() {
return Optional.empty();
}
@Override
protected List> getStaticColumns() {
return Arrays.asList();
}
@Override
protected List> getCounterColumns() {
return Arrays.asList();
}
@Override
protected EntityWithComplexIndices newInstanceFromCustomConstructor(final Row row, final List cqlColumns) {
throw new UnsupportedOperationException("Cannot instantiate entity 'info.archinnov.achilles.internals.entities.EntityWithComplexIndices' using custom constructor because no custom constructor (@EntityCreator) is defined");
}
/**
* Utility class to expose all fields with their CQL type for function call */
public static final class ColumnsForFunctions {
/**
*
* Field to be used for manager.dsl().select().function(...) call
*
* This is an alias for the field "id" */
public final Long_Type ID = new Long_Type(Optional.empty()){
@Override
protected String cqlColumn() {
return "id";
}
@Override
public boolean isFunctionCall() {
return false;
}
}
;
/**
*
* Field to be used for manager.dsl().select().function(...) call
*
* This is an alias for the field "clust1" */
public final Integer_Type CLUST_1 = new Integer_Type(Optional.empty()){
@Override
protected String cqlColumn() {
return "clust1";
}
@Override
public boolean isFunctionCall() {
return false;
}
}
;
/**
*
* Field to be used for manager.dsl().select().function(...) call
*
* This is an alias for the field "clust2" */
public final Integer_Type CLUST_2 = new Integer_Type(Optional.empty()){
@Override
protected String cqlColumn() {
return "clust2";
}
@Override
public boolean isFunctionCall() {
return false;
}
}
;
/**
*
* Field to be used for manager.dsl().select().function(...) call
*
* This is an alias for the field "clust3" */
public final String_Type CLUST_3 = new String_Type(Optional.empty()){
@Override
protected String cqlColumn() {
return "clust3";
}
@Override
public boolean isFunctionCall() {
return false;
}
}
;
/**
*
* Field to be used for manager.dsl().select().function(...) call
*
* This is an alias for the field "simpleIndex" */
public final String_Type SIMPLE_INDEX = new String_Type(Optional.empty()){
@Override
protected String cqlColumn() {
return "simpleindex";
}
@Override
public boolean isFunctionCall() {
return false;
}
}
;
/**
*
* Field to be used for manager.dsl().select().function(...) call
*
* This is an alias for the field "collectionIndex" */
public final List_String_Type COLLECTION_INDEX = new List_String_Type(Optional.empty()){
@Override
protected String cqlColumn() {
return "collectionindex";
}
@Override
public boolean isFunctionCall() {
return false;
}
}
;
/**
*
* Field to be used for manager.dsl().select().function(...) call
*
* This is an alias for the field "fullIndexOnCollection" */
public final Set_String_Type FULL_INDEX_ON_COLLECTION = new Set_String_Type(Optional.empty()){
@Override
protected String cqlColumn() {
return "fullindexoncollection";
}
@Override
public boolean isFunctionCall() {
return false;
}
}
;
/**
*
* Field to be used for manager.dsl().select().function(...) call
*
* This is an alias for the field "indexOnMapKey" */
public final Map_String_String_Type INDEX_ON_MAP_KEY = new Map_String_String_Type(Optional.empty()){
@Override
protected String cqlColumn() {
return "indexonmapkey";
}
@Override
public boolean isFunctionCall() {
return false;
}
}
;
/**
*
* Field to be used for manager.dsl().select().function(...) call
*
* This is an alias for the field "indexOnMapValue" */
public final Map_Integer_String_Type INDEX_ON_MAP_VALUE = new Map_Integer_String_Type(Optional.empty()){
@Override
protected String cqlColumn() {
return "indexonmapvalue";
}
@Override
public boolean isFunctionCall() {
return false;
}
}
;
/**
*
* Field to be used for manager.dsl().select().function(...) call
*
* This is an alias for the field "indexOnMapEntry" */
public final Map_Integer_String_Type INDEX_ON_MAP_ENTRY = new Map_Integer_String_Type(Optional.empty()){
@Override
protected String cqlColumn() {
return "indexonmapentry";
}
@Override
public boolean isFunctionCall() {
return false;
}
}
;
/**
*
* Field to be used with SystemFunctions.token(xxx_AchillesMeta.COLUMNS.PARTITION_KEYS, "tokens") call
*
*/
public final PartitionKeys_Type PARTITION_KEYS = new PartitionKeys_Type(new ArrayList() {
{
add("id"); }
})
;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy