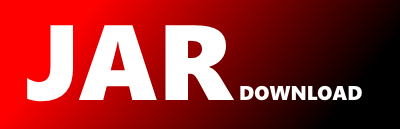
info.archinnov.achilles.generated.dsl.EntityForCastFunctionCall_Select Maven / Gradle / Ivy
package info.archinnov.achilles.generated.dsl;
import com.datastax.driver.core.querybuilder.QueryBuilder;
import com.datastax.driver.core.querybuilder.Select;
import info.archinnov.achilles.generated.meta.entity.EntityForCastFunctionCall_AchillesMeta;
import info.archinnov.achilles.internals.dsl.query.select.AbstractSelect;
import info.archinnov.achilles.internals.dsl.query.select.AbstractSelectColumns;
import info.archinnov.achilles.internals.dsl.query.select.AbstractSelectColumnsTypeMap;
import info.archinnov.achilles.internals.dsl.query.select.AbstractSelectFrom;
import info.archinnov.achilles.internals.dsl.query.select.AbstractSelectFromJSON;
import info.archinnov.achilles.internals.dsl.query.select.AbstractSelectFromTypeMap;
import info.archinnov.achilles.internals.dsl.query.select.AbstractSelectWhere;
import info.archinnov.achilles.internals.dsl.query.select.AbstractSelectWhereJSON;
import info.archinnov.achilles.internals.dsl.query.select.AbstractSelectWherePartition;
import info.archinnov.achilles.internals.dsl.query.select.AbstractSelectWherePartitionJSON;
import info.archinnov.achilles.internals.dsl.query.select.AbstractSelectWherePartitionTypeMap;
import info.archinnov.achilles.internals.dsl.query.select.AbstractSelectWhereTypeMap;
import info.archinnov.achilles.internals.entities.EntityForCastFunctionCall;
import info.archinnov.achilles.internals.metamodel.AbstractEntityProperty;
import info.archinnov.achilles.internals.metamodel.functions.FunctionCall;
import info.archinnov.achilles.internals.options.CassandraOptions;
import info.archinnov.achilles.internals.runtime.RuntimeEngine;
import info.archinnov.achilles.type.SchemaNameProvider;
import info.archinnov.achilles.validation.Validator;
import java.lang.Class;
import java.lang.Integer;
import java.lang.Long;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.SuppressWarnings;
import java.util.Arrays;
import java.util.List;
import java.util.Optional;
import java.util.stream.Collectors;
import org.apache.commons.lang3.ArrayUtils;
public final class EntityForCastFunctionCall_Select extends AbstractSelect {
protected final EntityForCastFunctionCall_AchillesMeta meta;
protected final Class entityClass = EntityForCastFunctionCall.class;
public EntityForCastFunctionCall_Select(RuntimeEngine rte, EntityForCastFunctionCall_AchillesMeta meta) {
super(rte);
this.meta = meta;
}
/**
* Generate a SELECT ... id ... */
public final EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectColumns id() {
select.column("id");
return new EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectColumns(select);
}
/**
* Generate a SELECT ... value ... */
public final EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectColumns value() {
select.column("value");
return new EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectColumns(select);
}
/**
* Use this method to call a system or user-defined function.
All the system functions are accessible from the {@link info.archinnov.achilles.generated.function.SystemFunctions} class
All the user-defined functions and aggregates are accessible from the {@link info.archinnov.achilles.generated.function.FunctionsRegistry} class
System and user-defined functions accept only appropriate type. To pass in an entity field as function argument, use the generated manager.COLUMNS class which exposes all columns with their appropriate type
Example:
* {@literal @}Table
* public class MyEntity {
*
* ...
*
* {@literal @}Column("value_column")
* private String value;
*
* {@literal @}Column("list_of_string")
* private List strings;
*
* ...
*
* }
*
* {@literal @}FunctionsRegistry
* public interface MyFunctions {
*
* String convertListToJson(List strings);
*
* }
*
*
* ...
*
*
* manager
* .dsl()
* .select()
* // This call will generate SELECT cast(writetime(value_column) as text) AS writetimeOfValueAsString, ...
* .function(SystemFunctions.castAsText(SystemFunctions.writetime(manager.COLUMNS.VALUE)), "writetimeOfValueAsString")
* ...
*
* manager
* .dsl()
* .select()
* // This call will generate SELECT convertlisttojson(list_of_string) AS strings_as_json, ...
* .function(FunctionsRegistry.convertListToJson(manager.COLUMNS.STRINGS), "strings_as_json")
* ...
*
*
*
* @param functionCall the function call object
* @param alias mandatory alias for this function call for easier retrieval from the ResultSet
* @return a built-in function call passed to the QueryBuilder object
*/
public final EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectColumnsTypedMap function(final FunctionCall functionCall, final String alias) {
functionCall.addToSelect(select, alias);
return new EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectColumnsTypedMap(select);
}
/**
* Generate ... * FROM ... */
public final EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectFrom allColumns_FromBaseTable() {
final Select.Where where = select.all().from(meta.getKeyspace().orElse("unknown_keyspace_for_" + meta.entityClass.getCanonicalName()), meta.getTableOrViewName()).where();
return new EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectFrom(where, new CassandraOptions());
}
/**
* Generate ... * FROM ... using the given SchemaNameProvider */
public final EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectFrom allColumns_From(final SchemaNameProvider schemaNameProvider) {
final String currentKeyspace = lookupKeyspace(schemaNameProvider, meta.entityClass);
final String currentTable = lookupTable(schemaNameProvider, meta.entityClass);
final Select.Where where = select.all().from(currentKeyspace, currentTable).where();
return new EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectFrom(where, CassandraOptions.withSchemaNameProvider(schemaNameProvider));
}
/**
* Generate ... * FROM ... */
public final EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectFromJSON allColumnsAsJSON_FromBaseTable() {
final Select.Where where = select.json().all().from(meta.getKeyspace().orElse("unknown_keyspace_for_" + meta.entityClass.getCanonicalName()), meta.getTableOrViewName()).where();
return new EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectFromJSON(where, new CassandraOptions());
}
/**
* Generate ... * FROM ... using the given SchemaNameProvider */
public final EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectFromJSON allColumnsAsJSON_From(final SchemaNameProvider schemaNameProvider) {
final String currentKeyspace = lookupKeyspace(schemaNameProvider, meta.entityClass);
final String currentTable = lookupTable(schemaNameProvider, meta.entityClass);
final Select.Where where = select.json().all().from(currentKeyspace, currentTable).where();
return new EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectFromJSON(where, CassandraOptions.withSchemaNameProvider(schemaNameProvider));
}
public class EntityForCastFunctionCall_SelectColumns extends AbstractSelectColumns {
public EntityForCastFunctionCall_SelectColumns(Select.Selection selection) {
super(selection);
}
/**
* Generate a SELECT ... id ... */
public final EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectColumns id() {
selection.column("id");
return this;
}
/**
* Generate a SELECT ... value ... */
public final EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectColumns value() {
selection.column("value");
return this;
}
/**
* Use this method to call a system or user-defined function.
All the system functions are accessible from the {@link info.archinnov.achilles.generated.function.SystemFunctions} class
All the user-defined functions and aggregates are accessible from the {@link info.archinnov.achilles.generated.function.FunctionsRegistry} class
System and user-defined functions accept only appropriate type. To pass in an entity field as function argument, use the generated manager.COLUMNS class which exposes all columns with their appropriate type
Example:
* {@literal @}Table
* public class MyEntity {
*
* ...
*
* {@literal @}Column("value_column")
* private String value;
*
* {@literal @}Column("list_of_string")
* private List strings;
*
* ...
*
* }
*
* {@literal @}FunctionsRegistry
* public interface MyFunctions {
*
* String convertListToJson(List strings);
*
* }
*
*
* ...
*
*
* manager
* .dsl()
* .select()
* // This call will generate SELECT cast(writetime(value_column) as text) AS writetimeOfValueAsString, ...
* .function(SystemFunctions.castAsText(SystemFunctions.writetime(manager.COLUMNS.VALUE)), "writetimeOfValueAsString")
* ...
*
* manager
* .dsl()
* .select()
* // This call will generate SELECT convertlisttojson(list_of_string) AS strings_as_json, ...
* .function(FunctionsRegistry.convertListToJson(manager.COLUMNS.STRINGS), "strings_as_json")
* ...
*
*
*
* @param functionCall the function call object
* @param alias mandatory alias for this function call for easier retrieval from the ResultSet
* @return a built-in function call passed to the QueryBuilder object
*/
public final EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectColumnsTypedMap function(final FunctionCall functionCall, final String alias) {
functionCall.addToSelect(selection, alias);
return new EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectColumnsTypedMap(select);
}
/**
* Generate a ... FROM xxx ... */
public final EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectFrom fromBaseTable() {
final Select.Where where = selection.from(meta.getKeyspace().orElse("unknown_keyspace_for_" + meta.entityClass.getCanonicalName()), meta.getTableOrViewName()).where();
return new EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectFrom(where, new CassandraOptions());
}
/**
* Generate a ... FROM xxx ... using the given SchemaNameProvider */
public final EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectFrom from(final SchemaNameProvider schemaNameProvider) {
final String currentKeyspace = lookupKeyspace(schemaNameProvider, meta.entityClass);
final String currentTable = lookupTable(schemaNameProvider, meta.entityClass);
final Select.Where where = selection.from(currentKeyspace, currentTable).where();
return new EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectFrom(where, CassandraOptions.withSchemaNameProvider(schemaNameProvider));
}
}
public class EntityForCastFunctionCall_SelectColumnsTypedMap extends AbstractSelectColumnsTypeMap {
public EntityForCastFunctionCall_SelectColumnsTypedMap(Select.Selection selection) {
super(selection);
}
/**
* Generate a SELECT ... id ... */
public final EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectColumnsTypedMap id() {
selection.column("id");
return this;
}
/**
* Generate a SELECT ... value ... */
public final EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectColumnsTypedMap value() {
selection.column("value");
return this;
}
/**
* Use this method to call a system or user-defined function.
All the system functions are accessible from the {@link info.archinnov.achilles.generated.function.SystemFunctions} class
All the user-defined functions and aggregates are accessible from the {@link info.archinnov.achilles.generated.function.FunctionsRegistry} class
System and user-defined functions accept only appropriate type. To pass in an entity field as function argument, use the generated manager.COLUMNS class which exposes all columns with their appropriate type
Example:
* {@literal @}Table
* public class MyEntity {
*
* ...
*
* {@literal @}Column("value_column")
* private String value;
*
* {@literal @}Column("list_of_string")
* private List strings;
*
* ...
*
* }
*
* {@literal @}FunctionsRegistry
* public interface MyFunctions {
*
* String convertListToJson(List strings);
*
* }
*
*
* ...
*
*
* manager
* .dsl()
* .select()
* // This call will generate SELECT cast(writetime(value_column) as text) AS writetimeOfValueAsString, ...
* .function(SystemFunctions.castAsText(SystemFunctions.writetime(manager.COLUMNS.VALUE)), "writetimeOfValueAsString")
* ...
*
* manager
* .dsl()
* .select()
* // This call will generate SELECT convertlisttojson(list_of_string) AS strings_as_json, ...
* .function(FunctionsRegistry.convertListToJson(manager.COLUMNS.STRINGS), "strings_as_json")
* ...
*
*
*
* @param functionCall the function call object
* @param alias mandatory alias for this function call for easier retrieval from the ResultSet
* @return a built-in function call passed to the QueryBuilder object
*/
public final EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectColumnsTypedMap function(final FunctionCall functionCall, final String alias) {
functionCall.addToSelect(selection, alias);
return this;
}
/**
* Generate a ... FROM xxx ... */
public final EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectFromTypedMap fromBaseTable() {
final Select.Where where = selection.from(meta.getKeyspace().orElse("unknown_keyspace_for_" + meta.entityClass.getCanonicalName()), meta.getTableOrViewName()).where();
return new EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectFromTypedMap(where, new CassandraOptions());
}
/**
* Generate a ... FROM xxx ... using the given SchemaNameProvider */
public final EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectFromTypedMap from(final SchemaNameProvider schemaNameProvider) {
final String currentKeyspace = lookupKeyspace(schemaNameProvider, meta.entityClass);
final String currentTable = lookupTable(schemaNameProvider, meta.entityClass);
final Select.Where where = selection.from(currentKeyspace, currentTable).where();
return new EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectFromTypedMap(where, CassandraOptions.withSchemaNameProvider(schemaNameProvider));
}
}
public class EntityForCastFunctionCall_SelectFrom extends AbstractSelectFrom {
EntityForCastFunctionCall_SelectFrom(Select.Where where, CassandraOptions cassandraOptions) {
super(where, cassandraOptions);
}
/**
* Generate a SELECT ... FROM ... WHERE ... */
public final EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectWhere_Id where() {
return new EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectWhere_Id(where, cassandraOptions);
}
/**
* Generate a SELECT statement without the WHERE clause */
public final EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectEnd without_WHERE_Clause() {
return new EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectEnd(where, cassandraOptions);
}
}
public class EntityForCastFunctionCall_SelectFromTypedMap extends AbstractSelectFromTypeMap {
EntityForCastFunctionCall_SelectFromTypedMap(Select.Where where, CassandraOptions cassandraOptions) {
super(where, cassandraOptions);
}
/**
* Generate a SELECT ... FROM ... WHERE ... */
public final EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectWhereTypedMap_Id where() {
return new EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectWhereTypedMap_Id(where, cassandraOptions);
}
/**
* Generate a SELECT statement without the WHERE clause */
public final EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectEndTypedMap without_WHERE_Clause() {
return new EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectEndTypedMap(where, cassandraOptions);
}
}
public class EntityForCastFunctionCall_SelectFromJSON extends AbstractSelectFromJSON {
EntityForCastFunctionCall_SelectFromJSON(Select.Where where, CassandraOptions cassandraOptions) {
super(where, cassandraOptions);
}
/**
* Generate a SELECT ... FROM ... WHERE ... */
public final EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectWhereJSON_Id where() {
return new EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectWhereJSON_Id(where, cassandraOptions);
}
/**
* Generate a SELECT statement without the WHERE clause */
public final EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectEndJSON without_WHERE_Clause() {
return new EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectEndJSON(where, cassandraOptions);
}
}
public final class EntityForCastFunctionCall_SelectWhere_Id extends AbstractSelectWherePartition {
public EntityForCastFunctionCall_SelectWhere_Id(Select.Where where, CassandraOptions cassandraOptions) {
super(where, cassandraOptions);
}
public final EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectWhere_Id.Relation id() {
return new EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectWhere_Id.Relation();
}
public final class Relation {
/**
* Generate a SELECT ... FROM ... WHERE ... id = ? */
@SuppressWarnings("static-access")
public final EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectEnd Eq(Long id) {
where.and(QueryBuilder.eq("id", QueryBuilder.bindMarker("id")));
boundValues.add(id);
encodedValues.add(meta.id.encodeFromJava(id, Optional.of(cassandraOptions)));
return new EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectEnd(where, cassandraOptions);
}
/**
* Generate a SELECT ... FROM ... WHERE ... id IN ? */
@SuppressWarnings("static-access")
public final EntityForCastFunctionCall_Select.EntityForCastFunctionCall_SelectEnd IN(Long... id) {
Validator.validateTrue(ArrayUtils.isNotEmpty(id), "Varargs for field '%s' should not be null/empty", "id");
where.and(QueryBuilder.in("id",QueryBuilder.bindMarker("id")));
final List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy