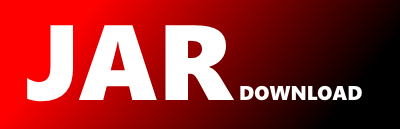
info.archinnov.achilles.generated.meta.entity.EntityWithDSESearch_AchillesMeta Maven / Gradle / Ivy
package info.archinnov.achilles.generated.meta.entity;
import com.datastax.driver.core.ConsistencyLevel;
import com.datastax.driver.core.DataType;
import com.google.common.collect.BiMap;
import com.google.common.collect.HashBiMap;
import com.google.common.reflect.TypeToken;
import info.archinnov.achilles.generated.function.Date_Type;
import info.archinnov.achilles.generated.function.Float_Type;
import info.archinnov.achilles.generated.function.Long_Type;
import info.archinnov.achilles.generated.function.String_Type;
import info.archinnov.achilles.internals.apt.annotations.AchillesMeta;
import info.archinnov.achilles.internals.codec.FallThroughCodec;
import info.archinnov.achilles.internals.entities.EntityWithDSESearch;
import info.archinnov.achilles.internals.metamodel.AbstractEntityProperty;
import info.archinnov.achilles.internals.metamodel.AbstractProperty;
import info.archinnov.achilles.internals.metamodel.SimpleProperty;
import info.archinnov.achilles.internals.metamodel.columns.ColumnInfo;
import info.archinnov.achilles.internals.metamodel.columns.ColumnType;
import info.archinnov.achilles.internals.metamodel.columns.FieldInfo;
import info.archinnov.achilles.internals.metamodel.columns.PartitionKeyInfo;
import info.archinnov.achilles.internals.metamodel.index.IndexInfo;
import info.archinnov.achilles.internals.strategy.naming.InternalNamingStrategy;
import info.archinnov.achilles.type.strategy.InsertStrategy;
import java.lang.Class;
import java.lang.Float;
import java.lang.Integer;
import java.lang.Long;
import java.lang.Override;
import java.lang.String;
import java.lang.SuppressWarnings;
import java.util.Arrays;
import java.util.Date;
import java.util.List;
import java.util.Optional;
/**
* Meta class of all entities of type EntityWithDSESearch
* The meta class is responsible for
*
* - determining runtime consistency levels (read/write,serial)
* - determining runtime insert strategy
* - trigger event interceptors (if any)
* - map a com.datastax.driver.core.Row back to an instance of EntityWithDSESearch
* - determine runtime keyspace name using static annotations and runtime SchemaNameProvider (if any)
* - determine runtime table name using static annotations and runtime SchemaNameProvider (if any)
* - generate schema during bootstrap
* - validate schema during bootstrap
* - expose all property meta classes for encoding/decoding purpose on unitary columns
*
*/
@AchillesMeta
public final class EntityWithDSESearch_AchillesMeta extends AbstractEntityProperty {
/**
* Meta class for 'id' property
* The meta class exposes some useful methods:
* - encodeFromJava: encode a property from raw Java to CQL java compatible type
* - encodeField: extract the current property value from the given EntityWithDSESearch instance and encode to CQL java compatible type
* - decodeFromGettable: decode from a {@link com.datastax.driver.core.GettableData} instance (Row, UDTValue, TupleValue) the current property
*
*/
@SuppressWarnings({"serial", "unchecked"})
public static final SimpleProperty id = new SimpleProperty(new FieldInfo<>((EntityWithDSESearch entity$) -> entity$.getId(), (EntityWithDSESearch entity$, Long value$) -> entity$.setId(value$), "id", "id", ColumnType.PARTITION, new PartitionKeyInfo(1, false), IndexInfo.noIndex()), DataType.bigint(), gettableData$ -> gettableData$.get("id", java.lang.Long.class), (settableData$, value$) -> settableData$.set("id", value$, java.lang.Long.class), new TypeToken(){}, new TypeToken(){}, new FallThroughCodec<>(Long.class));
/**
* Meta class for 'string' property
* The meta class exposes some useful methods:
* - encodeFromJava: encode a property from raw Java to CQL java compatible type
* - encodeField: extract the current property value from the given EntityWithDSESearch instance and encode to CQL java compatible type
* - decodeFromGettable: decode from a {@link com.datastax.driver.core.GettableData} instance (Row, UDTValue, TupleValue) the current property
*
*/
@SuppressWarnings({"serial", "unchecked"})
public static final SimpleProperty string = new SimpleProperty(new FieldInfo<>((EntityWithDSESearch entity$) -> entity$.getString(), (EntityWithDSESearch entity$, String value$) -> entity$.setString(value$), "string", "string", ColumnType.NORMAL, new ColumnInfo(false), IndexInfo.forDSESearch(true)), DataType.text(), gettableData$ -> gettableData$.get("string", java.lang.String.class), (settableData$, value$) -> settableData$.set("string", value$, java.lang.String.class), new TypeToken(){}, new TypeToken(){}, new FallThroughCodec<>(String.class));
/**
* Meta class for 'numeric' property
* The meta class exposes some useful methods:
* - encodeFromJava: encode a property from raw Java to CQL java compatible type
* - encodeField: extract the current property value from the given EntityWithDSESearch instance and encode to CQL java compatible type
* - decodeFromGettable: decode from a {@link com.datastax.driver.core.GettableData} instance (Row, UDTValue, TupleValue) the current property
*
*/
@SuppressWarnings({"serial", "unchecked"})
public static final SimpleProperty numeric = new SimpleProperty(new FieldInfo<>((EntityWithDSESearch entity$) -> entity$.getNumeric(), (EntityWithDSESearch entity$, Float value$) -> entity$.setNumeric(value$), "numeric", "numeric", ColumnType.NORMAL, new ColumnInfo(false), IndexInfo.forDSESearch(false)), DataType.cfloat(), gettableData$ -> gettableData$.get("numeric", float.class), (settableData$, value$) -> settableData$.set("numeric", value$, float.class), new TypeToken(){}, new TypeToken(){}, new FallThroughCodec<>(Float.class));
/**
* Meta class for 'date' property
* The meta class exposes some useful methods:
* - encodeFromJava: encode a property from raw Java to CQL java compatible type
* - encodeField: extract the current property value from the given EntityWithDSESearch instance and encode to CQL java compatible type
* - decodeFromGettable: decode from a {@link com.datastax.driver.core.GettableData} instance (Row, UDTValue, TupleValue) the current property
*
*/
@SuppressWarnings({"serial", "unchecked"})
public static final SimpleProperty date = new SimpleProperty(new FieldInfo<>((EntityWithDSESearch entity$) -> entity$.getDate(), (EntityWithDSESearch entity$, Date value$) -> entity$.setDate(value$), "date", "date", ColumnType.NORMAL, new ColumnInfo(false), IndexInfo.forDSESearch(false)), DataType.timestamp(), gettableData$ -> gettableData$.get("date", java.util.Date.class), (settableData$, value$) -> settableData$.set("date", value$, java.util.Date.class), new TypeToken(){}, new TypeToken(){}, new FallThroughCodec<>(Date.class));
/**
* Static class to expose "EntityWithDSESearch_AchillesMeta" fields for type-safe function calls */
public static final EntityWithDSESearch_AchillesMeta.ColumnsForFunctions COLUMNS = new EntityWithDSESearch_AchillesMeta.ColumnsForFunctions();
;
@Override
protected Class getEntityClass() {
return EntityWithDSESearch.class;
}
@Override
protected String getDerivedTableOrViewName() {
return "entitywithdsesearch";
}
@Override
protected BiMap fieldNameToCqlColumn() {
BiMap map = HashBiMap.create(4);
map.put("id", "id");
map.put("string", "string");
map.put("numeric", "numeric");
map.put("date", "date");
return map;
}
@Override
protected Optional getStaticReadConsistency() {
return Optional.empty();
}
@Override
protected Optional getStaticNamingStrategy() {
return Optional.empty();
}
@Override
protected List> getPartitionKeys() {
return Arrays.asList(id);
}
@Override
protected List> getClusteringColumns() {
return Arrays.asList();
}
@Override
protected List> getNormalColumns() {
return Arrays.asList(date,numeric,string);
}
@Override
protected List> getComputedColumns() {
return Arrays.asList();
}
@Override
protected boolean isCounterTable() {
return false;
}
@Override
protected Optional getStaticKeyspace() {
return Optional.of("achilles_dse_it");
}
@Override
protected Optional getStaticTableOrViewName() {
return Optional.of("search");
}
@Override
protected Optional getStaticWriteConsistency() {
return Optional.empty();
}
@Override
protected Optional getStaticSerialConsistency() {
return Optional.empty();
}
@Override
protected Optional getStaticTTL() {
return Optional.empty();
}
@Override
protected Optional getStaticInsertStrategy() {
return Optional.empty();
}
@Override
protected List> getStaticColumns() {
return Arrays.asList();
}
@Override
protected List> getCounterColumns() {
return Arrays.asList();
}
/**
* Utility class to expose all fields with their CQL type for function call */
public static final class ColumnsForFunctions {
/**
*
* Field to be used for manager.dsl().select().function(...) call
*
* This is an alias for the field "id" */
public final Long_Type ID = new Long_Type(Optional.empty()){
@Override
protected String cqlColumn() {
return "id";
}
@Override
public boolean isFunctionCall() {
return false;
}
}
;
/**
*
* Field to be used for manager.dsl().select().function(...) call
*
* This is an alias for the field "string" */
public final String_Type STRING = new String_Type(Optional.empty()){
@Override
protected String cqlColumn() {
return "string";
}
@Override
public boolean isFunctionCall() {
return false;
}
}
;
/**
*
* Field to be used for manager.dsl().select().function(...) call
*
* This is an alias for the field "numeric" */
public final Float_Type NUMERIC = new Float_Type(Optional.empty()){
@Override
protected String cqlColumn() {
return "numeric";
}
@Override
public boolean isFunctionCall() {
return false;
}
}
;
/**
*
* Field to be used for manager.dsl().select().function(...) call
*
* This is an alias for the field "date" */
public final Date_Type DATE = new Date_Type(Optional.empty()){
@Override
protected String cqlColumn() {
return "date";
}
@Override
public boolean isFunctionCall() {
return false;
}
}
;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy